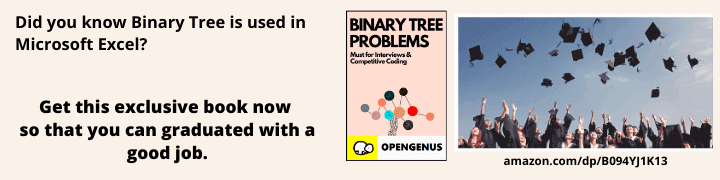
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
Reading time: 20 minutes | coding time: 10 minutes
In this article, you will learn:
- What is a function pointer and how to use it?
- How to pass a function as an argument to another function?
What is a Function pointer?
A pointer is a variable that holds the address of another variable. Function pointers are similar, except that instead of pointing to variables, they point to functions!
Declaration of function pointer
Syntax:
returnType * functionName(param list);
Note: Where, returnType is the type of the pointer that will be returned by the function functionName.
And param list is the list of parameters of the function which is optional.
Example:
int (*p2f)(double, char)
Note: Here int is a return type of function, p2f is name of the function pointer and (double, char) is an argument list of this function. Which means the first argument of this function is of double type and the second argument is char type.
#include<iostream>
using namespace std;
int *biger(int &, int &);
void main()
{
int num1, num2, *c;
cout<<"Enter two integers\n";
cin>>num1>>num2;
c = biger(num1, num2);
cout<<"The bigger value = "<<*c;
}
int *biger(int &x, int &y)
{
if(x>y)
{
return(&x);
}
else
{
return(&y);
}
}
Output:
Enter two integers
10
11
The bigger value = 11
Note : The above program passes two integers by reference to the function big() that compares the two integers values passed to it and returns the memory address of bigger value. Since the return value of big() is int ∗ i.e., an int pointer, it is received in an int pointer c (Notice the statement c = big(a, b);).
Then the value being pointed to by pointer c is printed on the screen.
How to initialize Function Pointers?
To initialize a function pointer, you must give it the address of a function in your program. The syntax is like any other variable:
#include <iostream>
using namespace std;
void my_int_func(int x)
{
cout<<x<<endl;
}
int main()
{
void (*foo)(int);
/* the ampersand is actually optional */
foo = &my_int_func;
return 0;
}
How to pass functions as arguments to other functions?
- One of the most useful things to do with function pointers is pass a function as an argument to another function. Functions used as arguments to another function are sometimes called callback functions.
- If you pass the pointer (address) of a function as an argument to another, when that pointer is used to call the function it points to it is said that a call back is made.
The use of callbacks is shown below-
#include<iostream>
using namespace std;
int adds(int a, int b)
{
return a+b;
}
int subs(int a, int b)
{
return a-b;
}
int DoIt(int x, int y, int (*c)(int, int))
{
cout<<"DoIt : "<<c(x,y)<<endl;
return 0;
}
int main()
{
DoIt(3,4,adds);
DoIt(4,3,subs);
//subs by another name
int (*minus)(int,int) = subs;
DoIt(4,2,minus);
return 0;
}
Output:
DoIt : 7
DoIt : 1
DoIt : 2