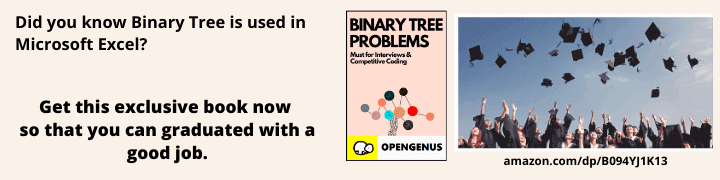
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
Reading time: 25 minutes
CSS Selectors are used to define the element(s) to which a particular set of CSS rules should be applied. The following article will take you through different selectors like class selector, attribute selectors, and selector combinations etc.
Universal Selector
Syntax : *
Use : Selects all the elements
Example :
HTML:
<p id="id1">I am the element "p" with id as "id1"</p>
<div class="class1">I am the element "div" with class as "class1"</div>
<span class="class2">I am the element "span" with class as "class2"</span>
CSS:
*{
color: blue;
}
Result:
Element Selector
Syntax : elementName
Use : Selects all the elements which are of the type elementName
Example :
HTML:
<p>I am the element "p"</p>
<span>I am the element "span"</span>
CSS:
p{
color: green;
}
Result:
Class Selector
Syntax : .className
Use : Selects all the elements which have a class as className
Example :
HTML:
<p class="first-element">I am the element with the class "first-element"</p>
<p class="second-element">I am the element with the class "second-element"</p>
CSS:
.first-element{
background-color : turquoise
}
Result:
Id Selector
Syntax : #idName
Use : Selects all elements which have an id as idName
Example :
HTML:
<p id="with-border">I am the element with id as "with-border"</p>
<p id="any-other"> I am the element with id as "any-other"</p>
CSS:
#with-border{
border : 2px solid blue
}
Result:
Attribute Selectors
CSS also supports numerous selectors, which filter the elements on the basis of their attribute values.
-
[attribute]
Selects all elements which have the given attribute, and can be src
, href
, target
etc.
Example:
HTML:
<a href="#"> A link with href attribute </a>
<p> A paragraph with no href attribute </p>
CSS:
[href]{
background-color: yellow
}
Result:
-
[attribute=value]
Selects all elements where the value of attribute is equal to value.
Example:
HTML:
<label><input type="text">Input with type "text"</label><br>
<label><input type="radio">Input with type "radio"</label>
CSS:
[type="text"]{
border: 2px red solid
}
Result:
-
[attribute~=value]
Selects all elements where the attribute contains the whole word value, either alone like box
, or separated with a space like blue box
.
Example:
HTML:
<img src="https://iq.opengenus.org/content/images/2019/07/box.PNG" title="box">
<img src="https://iq.opengenus.org/content/images/2019/07/blue-box.PNG" title="blue box">
<img src="https://iq.opengenus.org/content/images/2019/07/smallBox.PNG" title="smallBox">
CSS:
[title~="box"]{
border: 2px solid blue
}
Result:
-
[attribute|=value]
Selects all the elements with the attribute beginning with the whole word value, either standalone like main
or separated with a hyphen(-) like main-description
.
Example:
HTML:
<p class="main"> A paragraph with value of class as "main" </p>
<p class="main-description"> A paragraph with value of class as "main-description"</p>
<p class="end-main">A paragraph with value of class as "end-main"</p>
<p class="something main">A paragraph with value of class as "something main"</p>
<p class="mainabcd">A paragraph with value of class as "mainabcd"</p>
CSS:
[class|="main"]{
color: red
}
Result:
-
[attribute^=value]
Selects all elements where the attribute starts with value, not necessarily alone or separated by a hyphen, like main
, mainabcd
, main-description
will all be matched if the value is main
.
Example:
HTML:
<p class="main"> A paragraph with value of class as "main" </p>
<p class="main-description"> A paragraph with value of class as "main-description"</p>
<p class="end main">A paragraph with value of class as "end-main"</p>
<p class="something main">A paragraph with value of class as "something main"</p>
<p class="mainabcd">A paragraph with value of class as "mainabcd"</p>
CSS:
[class^="main"]{
background-color: cyan
}
Result:
-
[attribute$=value]
Selects all the elements where the attribute ends with value. For example, if the value is test
, it will match the attributes test
, abc-test
, box test
, lasttest
etc.
Example:
HTML:
<p class="abc-test">This is a paragraph with the value of class as "abc-test"</p>
<p class="first-test second">This is a paragraph with the value of class as "first-test second"</p>
<p class="box test">This is a paragraph with the value of class as "box test"</p>
<p class="lasttest">This is a paragraph with the value of class as "lasttest"</p>
CSS:
[class$="test"]{
background-color: yellow
}
Result:
-
[attribute*=value]
Selects all the elements where the attribute contains value as a substring. For example, blue
will match blue
, big-blue-box
, bluecolor
etc.
Example:
HTML:
<p class="blue">This is a paragraph with the value of class as "blue"</p>
<p class="bigbluebox">This is a paragraph with the value of class as "bigbluebox"</p>
<p class="big-blue-box">This is a paragraph with the value of class as "big-blue-box"</p>
<p class="boxer">This is a paragraph with the value of class as "boxer"</p>
<p class="bluecolor">This is a paragraph with the value of class as "bluecolor"</p>
CSS:
[class*="blue"]{
color: blue;
}
Result:
Note: You can write the value
enclosed in double quotation marks ("
) like box
, or without them like "box"
; both will work
Selector Combinations
Here, consider Foo
and Bar
to be two different selectors (of same or different types), for example Foo
can be #idName
and Bar
can be [href]
etc.
-
FooBar
To select element which satisfy the criteria for both Foo
and Bar
Example:
HTML:
<p class="class1">A paragraph with class as "class1"</p>
<p class="class2">A paragraph with class as "class2"</p>
<a class="class1">An anchor element with class as "class1"</a>
<div class="class3">A div element with class as "class3"</div>
CSS:
p.class1{
background-color: cyan
}
Result:
-
Foo,Bar
Selects all the elements satifying the criteria for at least one out of Foo
and Bar
Example:
HTML:
<p class="class1">A paragraph with class as "class1"</p>
<p class="class2">A paragraph with class as "class2"</p>
<a class="class1">An anchor element with class as "class1"</a>
<div class="class3">A div element with class as "class3"</div>
CSS:
p,.class1{
color: blue
}
Result:
-
Foo Bar
Selects all the elements which satisfy the criteria for Bar
, provided that they are also the descendants (not necessarily a child, but grandchildren etc. also) of an element satisfying the criteria for Foo
.
Example:
HTML:
<div class="first">
<span class="second">
<p href="#">An paragraph element with the class of its only parent and only grandparent as "second" and "first" respectively</p>
</span>
<p>A paragraph element with the class of its only parent as "first" </p>
</div>
<div class="third">
<p>A paragraph element with the class of parent as "third"</p>
</div>
CSS:
.first p{
border: 2px solid red
}
Result:
-
Foo > Bar
Selects all the elements satifying Bar
, provided they are also the direct children of an element satisfying Foo
Example:
HTML:
<div class="first">
<span class="second">
<p href="#">An paragraph element with the class of its only parent and only grandparent as "second" and "first" respectively</p>
</span>
<p>A paragraph element with the class of its only parent as "first" </p>
</div>
<div class="third">
<p>A paragraph element with the class of parent as "third"</p>
</div>
CSS:
.first > p{
border: 2px solid red
}
Result:
-
Foo + Bar
Select all the elements satisfying Bar
, provided they are placed immediately after an element satisfying Foo
Example:
HTML:
<div>The container div includes:
<h2>An h2 sub heading</h2>
<p>A small paragraph so the list isn't placed immediately after the h3 element</p>
<ul>
<li>List Item 1.1</li>
<li>List Item 1.2</li>
<li>List Item 1.3</li>
</ul>
<h3>An h3 sub heading</h3>
<p>A small paragraph so the list isn't placed immediately after the h3 element</p>
<ul>
<li>List Item 2.1</li>
<li>List Item 2.2</li>
<li>List Item 2.3</li>
</ul>
<h3>Another h3 sub heading which immediately preceeds the ul element</h3>
<ul>
<li>List Item 2.1</li>
<li>List Item 2.2</li>
<li>List Item 2.3</li>
</ul>
</div>
CSS:
h3 + ul{
border: 2px solid red
}
Result:
-
Foo ~ Bar
Selects all the elements satisfying Bar
, provided they have the common parent with an element satisfying Foo
, and are preceded (not necessarily immediately) by it
Example:
HTML:
<div>The container div includes:
<h2>An h2 sub heading</h2>
<p>A small paragraph so the list isn't placed immediately after the h3 element</p>
<ul>
<li>List Item 1.1</li>
<li>List Item 1.2</li>
<li>List Item 1.3</li>
</ul>
<h3>An h3 sub heading</h3>
<p>A small paragraph so the list isn't placed immediately after the h3 element</p>
<ul>
<li>List Item 2.1</li>
<li>List Item 2.2</li>
<li>List Item 2.3</li>
</ul>
<h3>Another h3 sub heading which immediately preceeds the ul element</h3>
<ul>
<li>List Item 2.1</li>
<li>List Item 2.2</li>
<li>List Item 2.3</li>
</ul>
</div>
CSS:
h3 ~ ul{
border: 2px solid red
}
Result: