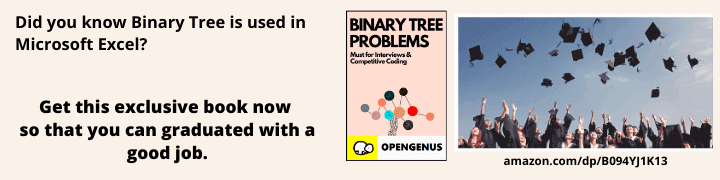
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
Steganography is one of the ways for people to secure data, and it is the art of hiding information within other media, such as images. Python offers a variety of tools and libraries that enable developers to implement steganography. In this article at OpenGenus, we will explore how to hide text data within images using Python, and delve into various methods and libraries that facilitate this process.
Table of Contents
- Understading Steganography and Image Steganography
- Python Libraries for Image Steganography
- Hiding Text Data in Images using Pillow
- Hiding Text Data in Images using Stegano
- Compare the Implementation Using Pillow with Stegano
- Best Practices for Image Steganography
- Conlcusion
Understanding Steganography and Image Steganography
Steganography is the art and science of concealing information within other forms of data, making it covert and difficult to detect. It is a technique used to ensure the confidentiality and secrecy of sensitive information. Image steganography, specifically, involves hiding data within digital images without noticeably altering their appearance. The goal is to embed the information in a way that remains undetectable to the human eye or even sophisticated analysis tools. By leveraging the properties of images, such as the vast amount of data they contain and the complexity of their pixel values, image steganography provides a means to securely transmit or store confidential data within innocuous-looking images, thereby adding an extra layer of protection.
Python Libraries for Image Steganography
- Pillow: Pillow is a powerful Python imaging library that supports various image file formats and provides extensive image processing capabilities. With Pillow, developers can read, modify, and save images. Although it does not offer direct steganography functionality, it forms the foundation for many steganography techniques.
- Stegano: Stegano is a lightweight steganography library designed for Python. It offers a simple and intuitive interface to encode and decode hidden data in images. Stegano supports various steganotraphic algorithms.
If we embed OpenGenus into this image, let's see the sample code of image steganography using Pillow and Stegano
Hiding Text Data in Images using Pillow
To use Pillow, developers can first
from PIL import Image
- Encoding Text into the Image: The encoding process contains converting text message into binary form and embedding it into the image. This process can start with modifying the lesat significant bits(LSBs)of the image pixels. By altering the LSBs, which is for the image's color intesnity, developers can insert the encoded message.
def hide(image_path, output_path, text):
image = Image.open(image_path)
binary_str = ''.join(format(ord(char), '08b') for char in text)
Len = (image.width * image.height)
if len(binary_str)>Len:
messagebox.showerror("Error", "Error")
pixel_index = 0
for bit in binary_str:
pixel = image.getpixel((pixel_index % image.width, pixel_index))
new_pixel = (pixel[0] & 0xFE) | int(bit)
image.putpixel((pixel_index % image.width, pixel_index), new_pixel)
pixel_index += 1
image.save(output_path)
messagebox.showinfo("Success", "Successful encoding")
- Decoding Text from the Image: Developers can start with extracting the modified LSBs and converting them back to text format. By examining the specific bit positions, developers can reconstruct the original message.
def show (image_path, text):
image = Image.open(image_path)
binary_str = text
for pixel_index in range(image.width * image.height):
pixel = image.getpixel((pixel_index % image.width, pixel_index))
binary_str += str(pixel[0] & 1)
text = ''.join(chr(int(binary_str[i:i+8], 2)) for i in range(0,
len(binary_str), 8))
return text
Hiding Text Data in Images using Stegano
The Stegno module in Python can help developers simplify the process of encoding and decoding text message in images.
To use the Stegano module, developers can first
from stegano import lsb
- Encoding Text into the Image: Stegano offers an API to encode text into an image. By specifying the image and the text message, Stegano handles the encoding process internally, ensuring the hidden data is seamlessly embedded.
def hide(image_path, output_path, text):
img = lsb.hide(image_path, text)
img.save(output_path)
messagebox.showinfo("Success", "Successful hide the message in the
image")
- Decoding Text from the Image: Stegano provides a convenient method to decode the hidden text from an image. By passing the image with encoded data to the decoding function, this module automatically extracts the hidden message.
def show(image_path):
img = lsb.reveal(image_path)
return img
Compare the Implementation Using Pillow with Stegano
Comparison | Pillow | Stegano |
---|---|---|
Ease of Use | Requires manual pixel manipulation | Provides higher-level functions for simplified usage |
Functionality | General-purpose image processing library | Specialized library focuesd on steganography |
Supported Techniques | Custom implementation of various techniques | Built-in support for common steganographic techniques |
Performance | Performance depends on implementation | Optimized for steganography operations |
Community | Large community and extensive documentation | Smaller community but still offers support and docs |
Best Practices for Image Steganography
Best Practices for Image Steganography are:
-
Choose appropriate steganographic algorithms: The choice of steganographic algorithms depends on the desired level of security and robustness. Consider algorithms that provide strong encryption and embedding techniques to protect the hidden data. Popular algorithms include LSB substitution, frequency domain techniques (DCT or DWT), and spread spectrum techniques.
-
Consider the image format: Different image formats may have varying levels of susceptibility to steganalysis and modifications. Some image formats may be more tolerant to embedding data without affecting the visual quality, while others may introduce noticeable artifacts. It is essential to understand the strengths and limitations of the chosen image format and its impact on the steganography process.
-
Test against attacks: Assess the strength and resilience of the steganographic algorithm by testing it against various attacks. These attacks may include statistical analysis, visual inspection, or attempts to extract hidden data. By subjecting the algorithm to rigorous testing, you can identify vulnerabilities and make necessary improvements to enhance the security of the hidden data.
-
Consider image size and quality: The size and quality of the image can impact the effectiveness of steganography. Large images with more pixels provide greater capacity for hiding data, but they may also attract suspicion. On the other hand, smaller images may limit the amount of data that can be embedded. Additionally, compressing or resizing the image can affect the embedded data and potentially make it more detectable. Striking a balance between image size, quality, and the amount of hidden data is crucial.
Conclusion
Image Steganography helps people save the text data in the message. Whether you choose to work with Pillow or Stegano or explore advanced techniques, image steganography in Python provides a versatile and powerful means to protect sensitive data. You can even try to use this to play with your friends using steganography to send message to each other. You may also use Python GUI like Tkinter associate with the Image Steganography to develop a small python project.