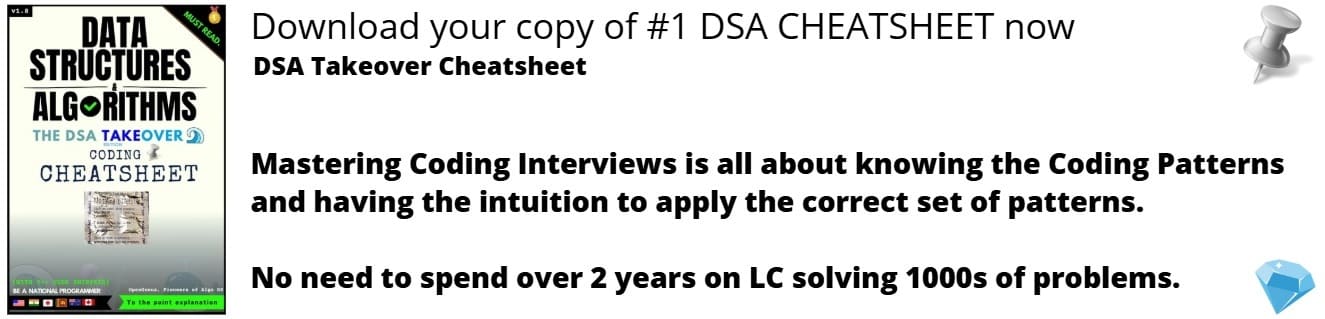
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
Reading time: 20 minutes | Coding time: 2 minutes
Vectors in C++ STL are same as the array in C++. The basic difference between a vector and an array is vectors are dynamic arrays with the facility to resize itself whenever a new element is pushed inside the vector or deleted from a vector unlike arrays which have predefined lengths.
Briefs about Vectors
- Dyanmic arrays present inside C++ STL library.
- Abitily to resize automatically.
- Stored in contiguous locations
- Accessed through iterators and pointers.
In this article, we take a look at different ways to initialize a vector in C++ Standard Template library including ways to use predefined array and vector to define a new vector.
Vector Initlialization Ways in C++
Let's say, We want to insert [10,20,30,40] in the vector. The following will be ways to initialize it.
- First way
In this way, we define a vector without a predefined size. he size increases dynamically in this case.
vector<int> v; //vector declaration without predefined size
v.push_back(10);
v.push_back(20);
v.push_back(30);
v.push_back(40);
As you can see, we used here push_back method which is used to push the elements into the vector from backside.
- Second Way
In this way, we define the vector by setting a fixed size.
vector<int> v(n); //by defining the vector size n. Assume n to be 4
v[0]=10;
v[1]=20;
v[2]=30;
v[3]=40;
- Third Way
In this way, we define a vector with a fixed size and a constant value for all elements.
vector<int> v(n,10);
//here n is the size and the above statement will copy 10 to all the indices in the vector
- Fourth Way
In this approach, we define all elements of the vector at the beginning itself.
vector<int> v{ 10, 20, 30, 40 };
//same as array initialization
- Fifth Way
In this way, we use another existing vector to define a new vector.
vector<int> v1{10,20,30,40};
vector<int> v2(v1.begin(),v1.end())
//initializing from another vector
- Sixth Way
In this way, we define a vector using an existing array.
int a[] = {10,20,30,40}
int n = sizeof(a)/sizeof(a[0]);
vector<int> v(a,a+n);
//initialization through array
- Seventh Way
If other libraries are allowed.
One can use #include <boost/assign/std/vector.hpp> header file
#include<boost/assign/std/vector.hpp>
using namespace boost::assign
vector<int> v;
v += 1,2,3,4;
Element Access from Vectors in C++
- First Way : Same Like Array
vector<int> v{10,20,30};
for(int i=0;i<v.size();i++)
{
cout<<v[i]<<" ";
}
- Second Way
for(auto it=v.begin();it!=v.end();it++)
{
cout<<*it<<" ";
}