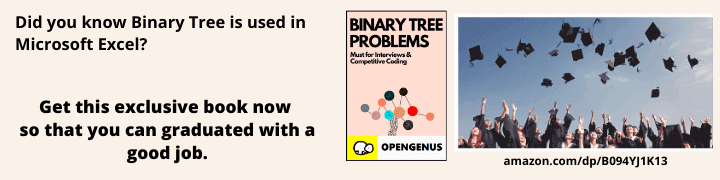
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
Set in C++ STL is a powerful data structure to maintain unique objects in sorted order. There are 5 different ways to initialize a set in C++ which we have covered in depth with C++ code examples.
The 5 Different ways to initialize Set in C++ STL:
- Default Constructor
- Initializer List
- Copy Constructor
- Range Constructor
- Move Constructor
We will dive into each method.
1. Default Constructor
It creates an empty set. Elements can be added to the set using the .insert() command.
#include <iostream>
#include <set>
using namespace std;
int main(){
set<int> s; // set s of size 0 is created
s.insert(2);
s.insert(8);
s.insert(4);
cout<<"After insertion set s has ";
for(int element : s)
cout<<element<<" ";
}
Output:
After insertion set s has 2 4 8
2. Initializer List
It constructs the set with contents of the initializer list. Note that this is works for c++11 or above only. If multiple elements in the list have same key values, then it is unspecified which element is inserted.
#include <iostream>
#include <set>
using namespace std;
int main(){
set<int> s ({9, 7, 8, 4, 1, 8});
cout<<"After insertion set s has ";
for(int element : s)
cout<<element<<" ";
}
Output:
After insertion set s has 1 4 7 8 9
3. Copy Constructor
Another way to initialize a set is to copy elements of another set and create a new set.
#include <iostream>
#include <set>
using namespace std;
int main(){
set<int> oldSet;
oldSet.insert(9);
oldSet.insert(3);
oldSet.insert(5);
oldSet.insert(3);
cout<<"Before copying oldSet has ";
for(int element : oldSet)
cout<<element<<" ";
set<int> s(oldSet);
cout<<"\nAfter copying oldSet has ";
for(int element : oldSet)
cout<<element<<" ";
cout<<"\nAfter insertion set s has ";
for(int element : s)
cout<<element<<" ";
}
Output:
Before copying oldSet has 3 5 9
After copying oldSet has 3 5 9
After insertion set s has 3 5 9
4. Range Constructor
It constructs the set with the contents of the range (first, last) from an iterable data structure. Note that if multiple elements in the range have the same key values, then it is unspecified which element is inserted.
#include <iostream>
#include <set>
using namespace std;
int main(){
int arr[5] = {3,2,4,0,1};
set<int> s(arr,arr+4);
cout<<"After insertion set s has ";
for(int element : s)
cout<<element<<" ";
}
Output:
After insertion set s has 0 2 3 4
5. Move Constructor
It is similar to the copy constructor, but instead of copying the items from the other set, it moves the elements to the new set, once the elements are moved, the previous set has no elements.
#include <iostream>
#include <set>
using namespace std;
int main(){
set<int> oldSet;
oldSet.insert(9);
oldSet.insert(3);
oldSet.insert(5);
oldSet.insert(3);
cout<<"oldSet before moving: ";
for(int element : oldSet)
cout<<element<<" ";
set<int> newSet(move(oldSet)); //now oldSet has 0 elements
cout<<"\noldSet after moving: ";
for(int element : oldSet)
cout<<element;
cout<<"\nnewSet after initializing: ";
for(int element : newSet)
cout<<element<<" ";
}
Output:
oldSet before moving: 3 5 9
oldSet after moving:
newSet after initializing: 3 5 9
With this article at OpenGenus, you must have the complete idea of different ways to initialize a set in C++ STL.