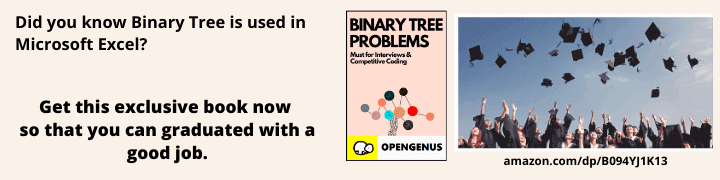
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
Printing text in color can add visual appeal to your C/C++ programs and make them more user-friendly. In this article, we will explain how to print text in color by using escape sequences in C and C++ Programming Language.
In C/C++, you can print text in color by using ANSI escape codes. These codes are special characters that are interpreted by the terminal to change the text color or other formatting options.
Table of contents:
- Method 1: Using escape sequences in C
- Method 2: Using system() function in C++
- Method 3: Using GetStdHandle() and SetConsoleTextAttribute() in C++
- Coloring Specific Words
- Summary
Method 1: Using escape sequences in C
Escape sequences are special characters that are preceded by a backslash () and are used to change the formatting of the text being printed. In C/C++, we can use escape sequences to change the color of the text. The most common escape sequence used for printing text in color is the ANSI escape code. The ANSI escape code is a standardized code that can be used to change the color and formatting of text in the terminal.
In C, you can use escape sequences to change the color of the text. The basic format of the escape sequence for printing text in color is \033[1;<color code>m
, where \033
is the escape character and [1;<color code>m
is the code for setting the text color.
\033[1;31m
\033[1;0m
is used to reset the color back to normal. All text after this will not be colored.
The different colors are as follows:
- Black: 30
- Red: 31
- Green: 32
- Yellow: 33
- Blue: 34
- Magenta: 35
- Cyan: 36
- White: 37
- Reset: 0
Program
#include <stdio.h>
int main()
{
printf("\033[1;31mWELCOME TO OPENGENUS\033[0m\n");
return 0;
}
Output: The text "WELCOME TO OPENGENUS" is printed in red color.
If you want to color only a specific word like "OPENGENUS" like in the above C code, the updated C program will be as follows:
#include <stdio.h>
int main()
{
printf("WELCOME TO \033[1;31mOPENGENUS\033[0m\n");
return 0;
}
Method 2: Using system() function in C++
This method uses the system() function to run a command on the command prompt. To change the text color, the command Color <color code>
is used. The <color code>
represents the color that you want to print the text in.
For example, to print text in red, you can use the following code:
Program
#include <iostream>
#include <windows.h>
using namespace std;
int main()
{
system("Color 0C");
cout << "WELCOME TO OPENGENUS";
return 0;
}
Output
Explanation:
Here, #include <windows.h>
is an include statement that includes the Windows API library, which allows the program to use the Windows-specific functions to change the text color.
In the main function, the system("Color 0C"); uses the system function to run a command on the command prompt. The command "Color 0C" changes the color to red
The second line cout << "WELCOME TO OPENGENUS"; uses the cout function to print the text "WELCOME TO OPENGENUS" to the console.
Finally, the last line return 0; is the return statement that ends the main function and returns the value 0 to the operating system, indicating that the program ran successfully.
Method 3: Using GetStdHandle() and SetConsoleTextAttribute() in C++
This method uses the GetStdHandle() function to get the standard handle for the console output buffer and the SetConsoleTextAttribute() function to set the text color of the console output buffer.
Program
#include <iostream>
#include <Windows.h>
int main()
{
HANDLE hConsole = GetStdHandle(STD_OUTPUT_HANDLE);
SetConsoleTextAttribute(hConsole, 9);
std::cout << "This text is blue" << std::endl;
SetConsoleTextAttribute(hConsole, 7);
}
Output
Output: The text "This text is blue" is printed in blue color.
Explanation:
In the main function, the first line HANDLE hConsole = GetStdHandle(STD_OUTPUT_HANDLE); declares a variable hConsole of type HANDLE, which is a handle to the console output buffer. The GetStdHandle function is used to get the standard handle for the console output buffer, and it is passed the constant STD_OUTPUT_HANDLE as an argument.
The SetConsoleTextAttribute(hConsole, 9); uses the SetConsoleTextAttribute function to set the text color of the console output buffer to blue. The first argument of the function is the handle to the console output buffer, which is passed as the hConsole variable. The second argument is an integer value representing the color, in this case 9 is blue.
The third line std::cout << "This text is blue" << std::endl; uses the cout function to print the text "This text is blue" to the console. The std::endl is a manipulator that inserts a newline character at the end of the text, so the text will appear on a new line.
Finally, the last line SetConsoleTextAttribute(hConsole, 7); uses the SetConsoleTextAttribute function to set the text color of the console output buffer back to the default color (white), this is done by passing the integer value 7 as the second argument.
Coloring Specific Words
To color only a specific word in the text, you can use the escape sequence before and after the word.
For example:
Program
#include <iostream>
#include <Windows.h>
int main()
{
HANDLE hConsole = GetStdHandle(STD_OUTPUT_HANDLE);
SetConsoleTextAttribute(hConsole, 9);
std::cout << "This text is blue" << std::endl;
SetConsoleTextAttribute(hConsole, 7);
}
Output
Explanation:
Where \033 is the escape character and [1;<color code>m
is the code for setting the text color. The <color code>
represents the color that you want to print the text in.
Summary
In conclusion, printing text in color is a simple and effective way to add visual appeal to your C/C++ programs. Escape sequences are a powerful tool for formatting text in the terminal, and the ANSI escape code is a standardized code that can be used to change the color and formatting of text in C/C++. However, keep in mind that these escape sequences may not work in all terminals, and you may also use libraries such as "ncurses" for this functionality.