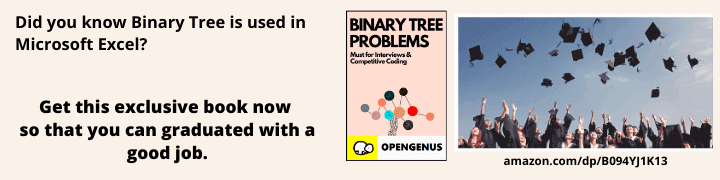
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
In this article, we will learn about all 32 keywords in C programming language and their uses.
As with other programming languages, there are words users are not allowed to use as variable names, a function name or expressions of any kind in C programming. These words are called Keywords.
Keywords are simply reserved words whose semantical meanings is already known to the compiler. If they are used outside their semantical meaning, it would result in a compilation error.
There are 32 keywords in the C programming language, and each one has a predefined meaning which is known to the compiler, and as such shouldn't be used otherwise. As a C developer, you should be aware of their existence and never use them for other purposes other than their original use.
Properties of keywords in C
Keywords in C has properties that should be noted when in use. Failure to adhere to these properties may result in unexpected behaviour.
- Keywords are case-sensitive. They are defined in lowercase letters and can only be used in lowercase.
- Keywords has a semantic meaning known to the C compilers and their meaning cannot be overridden by the users.
- Keywords cannot be used as a variable name, function and other expressions.
There 32 reserved keywords in C programming language.
The reserved words in C include;
S/N | Keywords | Meaning |
---|---|---|
1 | auto | Used to define automatic storage class |
2 | break | Used to terminate loop and switch statements |
3 | case | Used to represent a case(options) in switch statement |
4 | char | Used to define a character data type |
5 | const | Used to define a constant variable |
6 | continue | Used to skip a process in loop and pass control to the beginning of the loop |
7 | default | Used to represent a default case in switch statement |
8 | do | Used to define a block in a do-while loop |
9 | double | Used to define a floating double datatype |
10 | else | Used as a fallback statement in if-else statement for a falsy condition |
11 | enum | Used to define enumerated data type |
12 | extern | Used to extend variable or function to another program files |
13 | float | Used to define a float data type |
14 | for | Used to define a for-loop statement block |
15 | goto | Used to jump statement in loop block |
16 | if | Used to define a conditional if-else statement |
17 | int | Used to define an integer variable |
18 | long | Used to modify a basic data type |
19 | register | Used to define register CPU variable |
20 | return | Used to terminate function execution and return value to the calling function |
21 | short | Used to modify a basic data type |
22 | signed | Used to modify a basic data type |
23 | sizeof | Used to ascertain the size of a variable type |
24 | static | Used to define static variable |
25 | struct | Used to define structure object |
26 | switch | Used to define switch-case statement |
27 | typydef | Used to define a temporary name for data type |
28 | union | Used to combine different data type sharing the same memory space |
29 | unsigned | Used to modify a basic data type |
30 | void | Means Nothing - pass as a parameter and return value of a function |
31 | volatile | Used to define a volatile variable object |
32 | while | Used to define a while-loop statement |
auto
The auto keyword in C programming language is used to represent the automatic storage class. A variable declared with auto keyword can only be accessed within the function or block in which it was defined. By default, auto has garbage values assigned to them when they are uninitialized.
The example below demonstrates the use of the auto keywords in C
int main(void)
{
auto int age = 12;
printf("age value: %d\n", age);
return (0);
}
Output:
age value: 12
break and continue
The break and continue keywords are used in a loop to skip or terminate loop process. break terminates the execution of the innermost loop and switch statement whilst continue skips statement execution of a loop process.
The example below demonstrates the use of break and continue keywords in C
int main(void)
{
int i;
for (i = 1; i <= 10; i++)
{
if (i % 2 == 0)
{
continue;
}
if (i == 7)
{
break;
}
printf("%d, ", i);
}
return (0);
}
Output:
1, 3, 5
case, switch and default
These three keywords are used in the switch statement along with the break statement. The switch statement is usually used as an alternative to an if-else statement. Invariably, it is suitable for a single conditional statement with different cases.
The example below demonstrates the use of the switch, case and default keyword in C
int main(void)
{
char grade = 'B';
switch(grade)
{
case 'A':
printf("Excellent\n");
break;
case 'B':
printf("Very Good\n");
break;
case 'C':
printf("Good\n");
break;
case 'F':
printf("Fail\n");
break;
default:
printf("Enter correct grade!\n");
}
return (0);
}
Output:
Very Good
char
The char keyword is a reserved word used to declare single-character variables. It is well known as a data type in C as it is one of C's basic data types. This keyword stores a single character and requires a single byte of memory space.
The example below demonstrates the use of the char keyword in C
c
int main(void)
{
char ch = 'A';
printf("ch value: %c\n", ch);
return (0);
}
Output:
ch value: A
**const**
The const keyword is used for a declaration of a constant variable. As the name implies, a variable declared with a *const* can never be written to. This is because, *const* stores its value on the rodata (readonly) segment of the memory. C programmers uses const to declare a variable when they don't want its initial value to be changed or be overridden by users.
The example below demonstrates the use of the const identifier in C
```c
int main(void)
{
const float pi = 3.1416
pi = 3.1417 // Error
printf("Pi value: %f\n", pi);
return (0);
}
Output:
Pi value: 3.1416
do, while and for
There are three (3) types of loop statements in C programming language each with its usage but most of the time, used interchangeably, specifically for and while keywords.
do-while
The do-while statement is used when it is required for a program to run once before checking for the condition. The program will run at least once before the condition attached to it is checked.
while
The while statement is suitable for a loop condition without finite time of runtime. In other words, It is mostly used when we are testing for a condition that we are not so sure of the number of times it would run before a given condition is met.
for
The for keyword is used for for loop. It is the most common type of loops among the three (3) loops. It is used when the number of times the condition would be tested is known.
Without further ado, let's experiment with different use cases of the three (3) types of loops explained above.
// do-while loop
int main(void)
{
int i = 0;
do
{
printf("%d ", i);
i++;
}while(i < 5)
return (0);
}
Output:
0 1 2 3 4
// while loop
int main(void)
{
int i = 10;
while(i > 5)
{
printf("%d ", i);
i--;
}
return (0);
}
Output:
10 9 8 7 6
// for loop
int main(void)
{
char ch;
for(ch = 'a'; ch <= 'z'; ch++)
{
printf("%c", ch);
}
return (0);
}
Output:
abcdefghijklmnopqrztuvwxyz
double and float
The double and float keywords are used to present floating data types. Floating data type is simple number in decimal place. In C, numbers in decimals can be stored in either double or float data type.
The following pieces of code demonstrate double and float keyword
int main(void)
{
float x = 10.2;
double y = 20.34;
printf("Float value %f\n", x);
printf("Double value %f\n", y);
return (0);
}
Output
Float value 10.2
Double value 20.34
if and else
The if-else statements are conditional statement in C programming language. They are often used to make a technical decision. When control reaches the if statement, it checks the condition in it, if the condition evaluates to be true, the expression in the if block gets executed, otherwise it executes the else block.
The example below demonstrates the usage of if-else statement in C
int main(void)
{
int x = 10;
int y = 20;
if (x > y)
printf("%d greater than %d\n", x, y);
else
printf("%d is the greatest\n"y);
return (0);
}
Output
20 is the greatest
enum
The enum keyword is used to define enumerated data types. This is a special data type that is defined by the users.
Here is a simple demonstration of the use of enum in C
// Declare weekDay enum
enum weekDay{Mon, Tue, Wed, Thur, Fri};
int main(void)
{
enum weekDay day;
day = Fri;
printf("%d\n", day);
return (0);
}
Output
4
extern
The extern keyword is used to declare a variable or function that has external linkage outside the program file. It is used to extend variables or functions to other functions in another file.
Example of a use of extern;
// calcAge.c
int birthYear = 2000
def calcAge(int birthYear)
{
return 2023 - birthYear;
}
// main.c
extern int birthYear;
int main(void)
{
printf("%d\n", calcAge(birthYear));
}
Output:
23
goto
The goto keyword is an unconditional jump statement that transfers control to the label(identifiers). The label must have at least one statement. It is advisable to use *break, continue and return keywords in place of goto whenever possible.
The below example demonstrates the use of the goto statement in C
int main(void)
{
printf("statement 1\n");
goto label3;
printf("statement 2\n");
label3:
printf("statement 3\n");
printf("statement 4\n");
}
Output
statement 1
statement 3
statement 4
int
The keyword int is used to declare an integer variable. It is another basic and commonly used data type in C. It is used to store numbers that are not in decimal, and it usually takes 4 or 2 bytes of memory space, depending on the architecture of the machine.
The example below demonstrates the use of int keyword in C
int main(void)
{
int age = 10;
printf("I'm %d years old.\n", age);
}
output
I'm 10 years old.
short, long, signed and unsigned
These keywords are type modifiers that alter the basic data types to yield a new type. They take different bytes of memory and thus have different ranges of numbers they can store.
Range of int type data types
Data types | Range |
---|---|
short int | -32768 to 32767 |
long int | -2147483648 to 214743648 |
signed int | -32768 to 32767 |
unsigned int | 0 to 65535 |
The below example demonstrates the use of short, long, signed and unsigned statements in C
int main(void)
{
short int a = 10;
// L or l is used for long integers in c
long int b = 123455l;
signed int d = -10;
unsigned int c = 345;
printf("short integer %hd\n", a);
printf("long integer %ld\n", b);
printf("signed integer %d\n", c);
printf("unsigned integer %u\n",d);
}
Output
short integer 10
long integer 123455
signed integer -10
unsigned integer 345
register
The register keyword stores the variable in the register CPU rather than the memory. The value stored in the register CPU, is fast fetch and accessible. Frequently used variables are stored in the register. The address of register variables cannot be ascertained. Using & (reference) operator on the register variable will result in a compilation error. Also, register variable can not be used to declare a global variable.
This example demonstrates the use of a register statement in C
int main(void)
{
register int x = 10;
printf("Register varaible %d\n", x);
}
Output
Register variable 10
int main(void)
{
register int x = 10;
int *ptr = &x; //Error
}
Output
error: address of register variable ‘x’ requested
return
The return keyword is used in the function definition. It is used to terminate functions and also returns control to the calling function. It can as well, return value to the calling function.
The example below shows the use case of a return statement in C
int sum(int a, int b)
{
// Terminates and returns value
return a + b;
}
int main(void)
{
int x = 10;
int y = 20;
int res = sum(x, y);
printf("The sum of %d and %d = %d", x, y, res);
return (0);
}
Output:
The sum of 10 and 20 = 30
sizeof
The sizeof keyword in c programming language is a unary operator. It is used to obtain the size of the operand in c. The result of the sizeof operator is an unsigned integer donated by size_t
The example below demonstrates the use of the sizeof operator in C
Syntax:
sizeof(char);
sizeof(int);
sizeof(float);
sizeof(double);
int main(void)
{
char a = 'c';
int b = 10;
float c = 12.5;
double d = 12.348;
printf("Size of char: %d", sizeof(a));
printf("Size of int: %d", sizeof(b));
printf("Size of float: %d", sizeof(c));
printf("Size of double: %d", sizeof(d));
return (0);
}
Output:
Size of char: 1
Size of int: 4
Size of float: 4
Size of double: 8
static
The static keyword is used to declare a static variable in c. A static variable is a variable that preserves its value even after it is out of its scope. A variable declared with auto or int does not have such property - its value gets destroyed immediately after they are out of its scope.
Syntax:
static data_type variabl_name = variable_value;
static int x = 1;
Assuming we need to know the number of time a function gets called, the static variable can be very helpful in such a scenario.
def fun(void)
{
static int count = 0;
count++;
return count;
}
int main(void)
{
printf("Count: %d\n", fun());
printf("Count: %d\n", fun());
printf("Count: %d\n", fun());
return (0);
}
Output:
Count: 1
Count: 2
Count: 3
If ordinary int or auto was used in the above function to declare the count variable, the result of the fun() function will always be 1. Again, unlike static, int and auto don't preserve their initial value.
struct
struct is a keyword used to define a structure. A structure is a user-defined data type in C. It is used to group different data types as a single data type.
Syntax:
struct Student
{
char fullName[50];
int age;
bool isAdult;
};
An example of struct in use;
#include <stdio.h>
#include <string.h>
int main(void)
{
struct Student john;
strcpy(john.fullName, "Bob John");
john.age = 12;
john.isAdult = false;
printf("My name is %s, I'm %d years old.\n", john.fullName, john.age);
}
Output
My name is Bob John, I'm 12 years old.
typedef
The typedef keyword is used to define a temporary name for a data type. Its sole purpose is to make our code more clean and readable.
Syntax:
typedef struct *doubly_linked_list dllist;
dllist Node;
union
The union keyword is another user-defined data type in c programming language. Each member in a union shares the same memory space. The example below exemplifies the keyword better.
Syntax:
union student
{
int age;
int score;
}std;
#include <stdio.h>
#include <string.h>
int main(void)
{
std.age = 12;
std.score = 35;
printf("age: %d score: %d\n", std.age, std.score);
}
Output
age: 35 score: 35
Age and score value became 35 because they both share the same memory space,.this means the score value got overwritten by the age value.
void
The void keyword denotes NULL. It means nothing. When a void is passed as an argument to a function or the return value of a function is set to void, it means, it accepts nothing or returns nothing.
The below example demonstrates the use of the void keyword in C
// It takes no arguement
int foo(void);
// It returns nothing
void greet(char name[])
{
printf("Hey, %s!\n, name");
}
int main(void)
{
greet("Bob");
}
Output
Hey, Bob!
volatile
The volatile keyword is used to create a volatile object. A volatile object in C programming language is an object whose value can be changed by the code outside the current code, at any point in time.
Syntax:
const volatile score = 50;
The score variable above was declared with a const keyword. By semantic meaning, its value cannot be changed. With volatile added during the declaration, score variable has now been typecast to change. Therefore, the value stored in the variable can be changed by the hardware as it is a volatile object.
With this article at OpenGenus, you must have the complete idea of all keywords in C Programming Language.