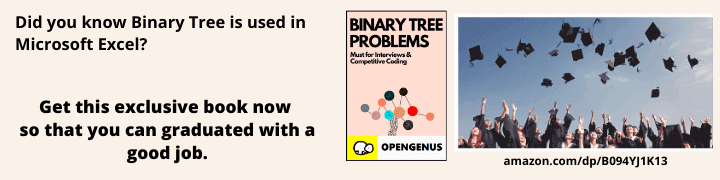
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
Python keywords are unique reserved words with specific meanings and use that can only be used for those specified purposes. You will never have to import these keywords into your code since they are always available.
Python keywords are distinct from built-in Python functions and types. The built-in functions and types are likewise always accessible, although their usage is not as restricted as keywords.
Assigning things to Python keywords is an example of something you can't do. If you attempt, you will receive a SyntaxError. If you try to assign something to a built-in function or type, you won't receive a SyntaxError, but it's still not a smart idea. Check out Invalid Syntax in Python: Common Reasons for SyntaxError for a more in-depth explanation of how keywords can be misused.
Python has thirty-five (35) keywords.
False | await | else | import | pass |
None | break | except | in | raise |
True | break | finally | is | return |
and | break | for | lambda | try |
as | break | from | nonlocal | while |
assert | break | global | not | with |
async | break | if | or | yield |
How to Identify Python Keywords
Python's keyword list has evolved. The await and async keywords, for example, were not introduced until Python 3.7. Also, print and exec were keywords in Python 2.7, but have since been converted to built-in functions in Python 3+ and are no longer listed as keywords.
In the sections that follow, you'll discover numerous methods for determining which words are keywords in Python.
Use an IDE With Syntax Highlighting
There are plenty of excellent Python IDEs available. They will all highlight keywords to distinguish them from other words in your code. This will assist you in rapidly identifying Python keywords when programming so that you do not use them erroneously.
Use Code in a REPL to Check Keywords
There are several ways to detect acceptable Python keywords and learn more about them in the Python REPL.
>>> help("keywords")
Here is a list of the Python keywords. Enter any keyword to get more help.
False class from or
None continue global pass
True def if raise
and del import return
as elif in try
assert else is while
async except lambda with
await finally nonlocal yield
break for not
As seen in the result above, you can then call help() again by giving in the precise term about which you want further information. You may accomplish this with the pass keyword, for example:
>>> help("pass")
The "pass" statement
********************
pass_stmt ::= "pass"
"pass" is a null operation — when it is executed, nothing happens. It
is useful as a placeholder when a statement is required syntactically,
but no code needs to be executed, for example:
def f(arg): pass # a function that does nothing (yet)
class C: pass # a class with no methods (yet)
Python additionally has a keyword module for programmatically interacting with Python keywords. Python's keyword module includes two useful members for working with keywords:
- list displays a list of all Python keywords for the Python version you're using.
- keyword () is a useful function for determining whether a string is also a keyword.
To receive a list of all the keywords in the Python version you're using, as well as a fast count of how many keywords are specified, call keyword.twist:
>>> import keyword
>>> keyword.twist
['False', 'None', 'True', 'and', 'as', 'assert', 'async', ...
>>> len(keyword.twist)
35
If you want to learn more about a keyword or work with keywords programmatically, Python has the documentation and tooling you need.
Look for a SyntaxError
Finally, another piece of evidence that a term you're using is a keyword is if you get a SyntaxError when you try to assign it, name a function with it, or do something else with it that isn't permitted. This one is a little more difficult to see, but it's a technique for Python to tell you when you're using a term wrongly.
Python Keywords and Their Usage
The sections that follow categorize Python keywords according to their usage. For example, the first group includes all terms used as values, whereas the second group includes all keywords used as operators. These classifications will help you better understand how keywords are used and will help you organize the huge list of Python keywords.
A few terminology mentioned in the following sections may be unfamiliar to you. They're defined here, and you should understand what they signify before continuing:
-
The Boolean assessment of a value is referred to as truthiness. A value's truthiness reveals whether it is true or false.
-
In the Boolean context, truthy refers to any value that evaluates to true. Pass a value as an argument to bool() to determine its veracity. If it returns True, the value is accurate. Non-empty strings, non-zero integers, non-empty lists, and many more are examples of truthy values.
-
Falsy refers to any value in a Boolean context that evaluates to false. Pass a value as an argument to bool() to determine if it is false. If it returns False, the value is incorrect. Examples of false values are ", 0, [], and set().
**Value Keywords: True, False, None **
Python keywords are used as values in three cases. These are singleton values, which may be used again and always refer to the same object. These values will most likely be seen and used frequently.
True and False Keywords
In Python programming, the True keyword represents the Boolean true value. False is a Python keyword comparable to True, but with the opposite Boolean value of false. In most programming languages, these keywords (true and false) are written in lowercase, however in Python, they are always written in uppercase.
True and False are Python keywords that may be applied to variables and directly compared to:
>>> x = True
>>> x is True
True
>>> y = False
>>> y is False
True
When most values in Python are provided to bool(), they evaluate to True. In Python, only a few values, to mention a few, will evaluate to False when supplied to bool(): 0, ", [], and. When you pass a value to bool(), it shows the truthiness of the value or the equivalent Boolean value. By giving the value to bool(), you may compare the truthiness of a value to True or False:
>>> x = "this is a truthy value"
>>> x is True
False
>>> bool(x) is True
True
>>> y = "" # This is falsy
>>> y is False
False
>>> bool(y) is False
True
It is worth noting that using it to compare a truthy value to True or False does not work. Only if you want to know if a value is True or False should you explicitly compare it to True or False.
When making conditional assertions based on the truthiness of a value, do not compare straight to True or False. You may rely on Python to do the truthiness check in conditionals for you:
>>> x = "this is a truthy value"
>>> if x is True: # Don't do this
... print("x is True")
...
>>> if x: # Do this
... print("x is truthy")
...
x is truthy
.
None Keyword
The Python keyword None has no meaning. None is represented in various programming languages as null, nil, none, under, or undefined.
None is also the default value returned by a function if it does not have a return statement:
>>> def func():
... print("hello")
...
>>> x = func()
hello
>>> print(x)
None
Check to see Null in Python: Understanding Python's NoneType Object for additional information on this highly essential and helpful Python keyword.
Keywords for Operator: and, or, not, in, is
As operators, several Python keywords are utilized. In other programming languages, these operators are represented by symbols such as &, |, and!. All of these Python operators are keywords:
Math Operator | Other Languages | Python Keyword |
---|---|---|
AND, ∧ | && | and |
OR, ∨ | || | or |
NOT, ¬ | ! | not |
CONTAINS, ∈ | in |
Python code was written with readability in mind. As a result, many operators in other programming languages that employ symbols are keywords in Python.
and Keyword
The Python keyword determines if both the left and right operands are true or false. If both operands are true, the outcome will also be true. If one is false, the result will also be false:
<expr1> and <expr2>
It is important to note that the outcomes of a statement are not always True or False. This is because of and's peculiar behavior. Rather than evaluating the operands to their Boolean values, it simply returns expr1> if it is false or expr2> otherwise. The results of a and statement might be provided to bool() to acquire an explicit True or False answer, or they could be utilized in a conditional if statement.
If you wanted to define an expression that accomplished the same thing as a and expression but did not utilize the and keyword, you could use the Python ternary operator:
left if not left else right
The preceding sentence yields the same outcome as left and right.
Because and returns the first operand if it is false and the last operand otherwise, you can use and in an assignment:
or Keyword
Python's keyword is used to determine whether at least one of the operands is true. If the or statement is true, it returns the first operand; otherwise, it returns the second operand:
<expr1> or <expr2>
The or keyword, like the and keyword, does not transform its operands to Boolean values. Instead, the outcome is determined by their honesty.
You might use a ternary expression to create something similar to an or expression without using or:
left if left else right
The expression has the same effect as either left or right. You'll also observe or exploit this behavior in assignments to capitalize on it. In most cases, this is avoided in favor of a more clear assignment.
not Keyword
The not keyword in Python is used to acquire the inverse Boolean value of a variable:
>>> val = " # Truthiness value is `False`
>>> not val
True
>>> val = 5 # Truthiness value is `True`
>>> not val
False
The not keyword is used to reverse the Boolean meaning or outcome in conditional statements or other Boolean expressions. In contrast to and or, not will determine the explicit Boolean value, True or False, and then return the inverse.
If you wanted to achieve the same result without using not, use the following ternary expression:
True if bool(<expr>) is False else False
.
in Keyword
The keyword in Python is a strong confinement check or membership operator. Given an element to locate and a container or sequence to search, it will return True or False depending on whether the element was found in the container:
Checking for a certain letter in a string is a suitable use of the in a keyword:
>>> name = "Chad"
>>> "c" in name
False
>>> "C" in name
True
The in keyword applies to all sorts of containers, including lists, dicts, sets, strings, and anything else that specifies contains() or can be iterated over.
is Keyword
The keyword in Python serves as an identity check. This is not the same as the == operator, which tests for equality. When two things are considered equal, they may not be the same object in memory. The is keyword indicates if two things are identical:
<obj1> is <obj2>
If obj1> and obj2> are the same object in memory, this will return True; otherwise, it will return False.
Most of the time, you'll see is used to determine whether an object is None. Because None is a singleton, only one instance of None can exist, all None values in memory are the same object.
If these ideas are unfamiliar to you, you may learn more about them by visiting Python '!='. Is Not 'is not': Python Object Comparison. Check see Operators and Expressions in Python for a more in-depth look at how it works.
Keywords for Control Flow: if, Elif, else
For control flow, three Python keywords are used: if, Elif, and else. These Python keywords enable you to apply conditional logic and run code in response to certain situations. These keywords are quite common—you'll find them in practically every Python program you see or develop.
if keyword
The if keyword begins a conditional statement. An if statement lets you construct a block of code that is only executed if the expression after the if is true.
An if statement's syntax begins with the keyword if at the beginning of the line, followed by a valid expression that will be evaluated for truthiness:
if <expr>:
<statements>
The if statement is a critical component of most programs. Check and read Conditional Statements in Python for additional information on the if statement.
Another application of the if keyword is in Python's ternary operator:
<var> = <expr1> if <expr2> else <expr3>
The following is a one-line variant of the if...else statement:
if <expr2>:
<var> = <expr1>
else:
<var> = <expr3>
If your expressions are simple statements, employing the ternary expression is a good technique to simplify your code. When the conditions become more complicated, it is often preferable to use the standard if statement.
elif Keyword
The elif statement looks and works similarly to the if statement, with two important exceptions:
- Elif can only be used after an if statement or another elif.
- You may use as many elif statements as necessary.
Elif is either else if (two independent words) or else if(both words mashed together) in other computer languages. When you encounter elif in Python, consider another if:
if <expr1>:
<statements>
elif <expr2>:
<statements>
elif <expr3>:
<statements>
Python does not contain a switch statement. Using if and elif to obtain the same capability that other programming languages give with switch statements is one option. Emulating switch/case Statements in Python has several techniques to reproduce the switch statement.
else Keyword
The otherwise statement, when used with the Python keywords if and elif, specifies a block of code that should be executed only if the other conditional blocks, if and elif, are all false:
if <expr>:
<statements>
else:
<statements>
It's worth noting that the else statement does not accept a conditional expression. Python programmers must understand the elif and else keywords and how to use them correctly. They, along with it, are some of the most frequently used components in any Python program.
Keywords for looping: for, while, break, continue, else
Looping and iteration are critical programming concepts. To generate and operate with loops, several Python keywords are utilized. These, like the Python keywords used for conditionals mentioned above, will be used and seen in almost every Python program you encounter. Understanding them and how to use them correctly can help you progress as a Python programmer.
for Keyword
The for loop is the most often used loop in Python. It is built by combining the Python keywords for and in, as previously discussed. A for loop's fundamental syntax is as follows:
for <element> in <container>:
<statements>
The for loop in Python is similar to the for-each loop in other programming languages. It assigns the value of each iteration to the variable given the object to iterate over:
>>> people = ["Yash", "Pratham", "keyur"]
>>> for the person in people:
... print(f"{person} was in The Office.")
...
Yash was in The Office.
Pratham was in The Office.
keyur was in The Office.
In this example, you begin with a list of people's names (container). The for loop begins with the for the keyword at the top of the line, then the variable to which each element of the list is assigned, the in keyword, and lastly the container (people).
Python's for loop is another essential component of every Python program. For additional information on loops, see Python "for" Loops (Definite Iteration).
while Keyword
Python's while loop uses the term while and functions similarly to a while loop in other programming languages. As long as the condition after the while keyword is true, the block following the while statement will be run repeatedly:
while <expr>:
<statements>
The while keyword with an expression that is always true is the simplest method to declare an endless loop in Python:
>>> while True:
... print("working...")
...
Check out Socket Programming in Python (Guide) for more examples of infinite loops in action. Python "while" Loops (Indefinite Iteration) are a good place to start learning more about while loops.
break Keyword
If you need to quit a loop quickly, use the break keyword. This keyword is applicable in both for and while loops:
for <element> in <container>:
if <expr>:
break
If you were summing the integers in a list of numbers and wanted to stop when the total exceeded a certain amount, you might use the break keyword:
>>> nums = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10]
>>> total_sum = 0
>>> for num in nums:
... total_sum += num
... if total_sum > 10:
... break
...
>>> total_sum
15
When working with loops, the Python keywords break and continue can be useful. Check out Python "while" Loops (Indefinite Iteration) for a more in-depth discussion of their applications. If you want to investigate another application for the break keyword, you may discover how to imitate do-while loops in Python.
continue Keyword
Python also provides a continue keyword for skipping to the next loop iteration. The continue keyword, like most other programming languages, allows you to stop running the current loop iteration and go to the next iteration:
for <element> in <container>:
if <expr>:
continue
While loops can also use the continue keyword. If the continue keyword is encountered in a loop, the current iteration is terminated and the next iteration of the loop is initiated.
else Keyword Used With Loops
You may use the else keyword in a loop in addition to conditional if expressions. When used in conjunction with a loop, the else keyword defines the code that should be executed if the loop ends properly, implying that the break was not used to quit the loop early.
The syntax for using else with a for loop is:
for <element> in <container>:
<statements>
else:
<statements>
This is analogous to using else with an if statement. Using else with a while loop appears to be similar:
while <expr>:
<statements>
else:
<statements>
You should read the section on using break and else with a for loop in the Python standard manual. It employs an excellent example to demonstrate the use of the else block.
It demonstrates a job of looping through the numbers two through nine to discover the prime numbers. One approach would be to use a regular for loop with a flag variable:
>>> for n in range(2, 10):
... prime = True
... for x in range(2, n):
... if n % x == 0:
... prime = False
... print(f"{n} is not prime")
... break
... if prime:
... print(f"{n} is prime!")
...
2 is prime!
3 is prime!
4 is not prime
5 is prime!
6 is not prime
7 is prime!
8 is not prime
9 is not prime
The prime flag can be used to indicate how the loop was exited. If it exited normally, the prime flag remains set to True. If it exited with a break, the prime flag will be False. Once outside the inner for loop, check the flag to see if the prime is True and, if so, print the prime number.
Keywords for Structure: def, class, with, as, pass, lambda
You'll need to use one of the Python keywords in this section to declare functions and classes or employ context managers. They're an important feature of the Python programming language, and knowing when to utilize them can help you become a better Python programmer.
def Keyword
The Python keyword def is used to define a class's function or method. In JavaScript and PHP, this is identical to function. The fundamental syntax for declaring a function using def is as follows:
def <function>(<params>):
<body>
Functions and methods may be extremely useful constructs in any Python program. Check read Defining Your Own Python Function to learn more about defining them and all of their intricacies.
class Keyword
In Python, the class keyword is used to declare a class. The following is the general syntax for declaring a class with class:
class MyClass(<extends>):
<body>
Classes are useful tools in object-oriented programming, and you should understand how to use them and define them. For further information, see Object-Oriented Programming (OOP) in Python 3.
with Keyword
Context managers are a very useful Python structure. Before and after the statements you define, each context manager performs specified code. You use the with keyword to utilize one:
with <context manager> as <var>:
<statements>
Using with allows you to declare code that will be executed within the context manager's scope. Working with file I/O in Python is the most fundamental example of this.
A context manager would be used if you wanted to open a file, do something with it, and then ensure that the file was properly closed. Consider the following example, in which the file names.txt includes a list of names, one per line:
The file I/O context manager supplied by open() and triggered by the keyword opens the file for reading, assigns the open file reference to input_file, and then runs the code specified in the with block. The file reference then closes when the block is performed. Even if you are with block code throwing an exception, the file reference will still shut.
Python Timer Functions: Three Ways to Monitor Your Code is a nice example of utilizing timer and context managers.
lambda Keyword
The lambda keyword is used to define a function that has no name and only one statement whose results are returned. Lambda functions are functions defined with the lambda operator:
lambda <args>: <statement>
This is the same as declaring a function with def:
def p10(x):
return x**10
A lambda function is commonly used to declare a distinct behavior for another function. Assume you wanted to sort a list of strings by their integer values. sorted()'s default behavior is to arrange the strings alphabetically. However, you can use sorted() to specify which key the list should be sorted on.
pass Keyword
Because Python lacks block indicators to indicate the end of a block, the pass keyword is used to indicate that the block is intentionally left blank. It's the same as saying "no operation." Here are some examples of how to use a pass to signal that the block is empty:
def my_function():
pass
class MyClass:
pass
if True:
pass
Keywords for passing back: return, yield
The Python keywords return and yield is used to define what is returned from functions or methods. Understanding when and where to utilize return is critical for improving your Python programming skills. The yield keyword is a more sophisticated Python feature, but it may also be a valuable tool to learn.
return Keyword
The return keyword in Python is only valid as part of a function declared with def. Python will exit the function when it sees this keyword and return the results of whatever comes after the return keyword:
def <function>():
return <expr>
When no expression is provided, a return will return None by default:
>>> def return_none():
... return
...
>>> return_none()
>>> r = return_none()
>>> print(r)
None
In a function, you can even use the return keyword multiple times. This gives you the ability to have numerous exit locations in your function. The following recursive approach to computing factorial is a classic example of when you would wish to have several return statements:
def factorial(n):
if n == 1:
return 1
else:
return n * factorial(n - 1)
There are two scenarios in which you might wish to return from the factorial function described above. The first is when the number is 1, and the second is when you wish to multiply the current number by the factorial value of the following number.
yield Keyword
The yield keyword in Python is similar to the return keyword in that it indicates what is returned from a function. When a function has a yield statement, the result is a generator. The generator may then be supplied to Python's built-in next() method to receive the function's next value.
When you use yield statements to invoke a function, Python performs the function until it reaches the first yield keyword and then returns a generator. These are referred to as generator functions:
def <function>():
yield <expr>
The most obvious example is a generator function that always returns the same set of values:
>>> def family():
... yield "Pam"
... yield "Jim"
... yield "Cece"
... yield "Philip"
...
>>> names = family()
>>> names
<generator object family at 0x7f47a43577d8>
>>> next(names)
'Pam'
>>> next(names)
'Jim'
>>> next(names)
'Cece'
>>> next(names)
'Philip'
>>> next(names)
Traceback (most recent call last):
File "<stdin>", line 1, in <module>
StopIteration
When the StopIteration exception is thrown, the generator has finished returning values. To run through the names again, you'd have to call family() and receive a new generator. A generator function is usually invoked as part of a for loop, which handles the next() calls for you.
Keywords for Import: import, from, as
You will need to import any tools that, unlike Python keywords and built-ins, are not currently available to your Python program. Many useful modules are available in Python's standard library and can be accessed by importing them. There are many additional important libraries and tools available in PyPI that you will need to import into your programs after they have been installed in your environment.
The three Python keywords used for importing modules into your program are briefly described here. Python Modules and Packages: An Introduction and Python Import: Advanced Techniques and Tips have further information on these keywords.
import Keyword
The import keyword in Python is used to incorporate or import a module into your Python program. The basic syntax is as follows:
import <module>
After that statement executes, your program will have access to the module>.
For example, if you wish to utilize the Counter class from the standard library's collections module, you may use the following code:
>>> import collections
>>> collections.Counter()
Counter()
When you import collections in this manner, your program gains access to the whole collections module, including the Counter class. You may access all of the tools in that module by using the module name. You may get to Counter by referencing it from the module: collections. Counter.
from Keyword
To import anything particular from a module, use the from keyword in conjunction with import:
from <module> import <thing>
This will import whatever is included within module> to be utilized within your program. The Python keywords from and import are used in tandem.
If you wish to utilize Counter from the standard library's collections module, you may import it specifically:
>>> from collections import Counter
>>> Counter()
Counter()
When you import Counter in this way, you get the Counter class but nothing more from the collections module. You no longer need to refer to the counter from the collections module.
as Keyword
To alias an imported module or tool, use them as the keyword. It's used in conjunction with the Python keywords import and from to modify the name of what's being imported:
import <module> as <alias>
from <module> import <thing> as <alias>
For modules with extremely long names or a frequently used import alias, as might be useful when constructing the alias.
If you wish to import the Counter class from the collections module but rename it, you may alias it with as:
>>> from collections import Counter as C
>>> C()
Counter()
The counter is now accessible for usage in your program, but it is referred to by C instead. Import aliases are more commonly used with NumPy or Pandas packages.
Keywords for error handling : try, except, raise, finally, else, assert
The raising and catching of exceptions is a frequent feature of every Python program. Because this is such an important aspect of all Python code, there are several Python keywords available to assist you in making this part of your code clear and concise.
The sections that follow go through these Python keywords and how to use them. Python Exceptions: An Introduction is a more in-depth lesson on these keywords.
try Keyword
Any exception-handling block in Python begins with the try keyword. This is true for the majority of other programming languages that include exception handling.
The try block contains code that may throw an exception. Several other Python keywords are associated with try and are used to define what should be done if various exceptions or situations are raised. Except, otherwise, and finally:
try:
<statements>
<except|else|finally>:
<statements>
A try block isn't acceptable unless it includes at least one of the other Python keywords for handling exceptions as part of the overall try statement.
If you wanted to compute and return the miles per gallon of gas (mpg) based on the number of miles traveled and the amount of gas consumed, you might construct a function like this:
def mpg(miles, gallons):
return miles/gallons
The first issue you may see is that your code may throw a ZeroDivisionError if the gallons argument is set to 0.
except Keyword
The except keyword in Python is used in conjunction with trying to describe what to do when certain exceptions are thrown. With a single try, you can have one or more except blocks. The fundamental syntax is as follows:
try:
<statements>
except <exception>:
<statements>
Using the previous mpg() example, you could also do something specific if someone passes types that will not work with the / operator. After defining mpg() in the preceding example, try using it with strings instead of numbers:
To tackle this circumstance, you may also rewrite mpg() and utilize several unless blocks:
def mpg(miles, gallons):
try:
mpg = miles/gallons
except for ZeroDivisionError:
mpg = None
except for TypeError as ex:
print("you need to provide numbers")
raise ex
return mpg
In this scenario, you modify mpg() such that it only throws a TypeError exception after showing a pleasant reminder on the screen.
It's worth noting that the until keyword can coexist with the as keyword. This has the same effect as the previous usage of as in that it gives the raised exception an alias so that you may deal with it in the unless block.
raised Keyword
The raise keyword causes an exception to be raised. If you need to raise an exception, you may do it by using raise followed by the exception to be raised:
raise <exception>
In the mpg() example, you previously used raise. You re-raise the exception after printing a message to the screen after you catch the TypeError.
final Keyword
Finally, the Python keyword is useful for expressing code that should be executed regardless of what occurs in the try, except, or otherwise blocks. Finally, use it as part of a try block and provide the statements that should be performed regardless of what happens:
try:
<statements>
finally:
<statements>
Using the previous example, it may be useful to declare that, regardless of what occurs, you want to know what parameters the function was called with. You might change mpg() to include a final block that does this:
def mpg(miles, gallons):
try:
mpg = miles/gallons
except for ZeroDivisionError:
mpg = None
except for TypeError as ex:
print("you need to provide numbers")
raise ex
finally:
print(f"mpg({miles}, {gallons})")
return mpg
Finally, in your exception-handling code, the keyword can be quite beneficial.
else Keyword Used With try and except
You've learned that the else keyword in Python can be used with both the if keyword and loops, but it has one additional application. It may be coupled with the Python keywords to try and accept. You can only use else this way if you also use at least one except block:
try:
<statements>
except <exception>:
<statements>
else:
<statements>
The code in the else block is only performed in this context if no exception was triggered in the try block. In other words, if the try block successfully performed all of the code, the otherwise block code would be executed.
assert Keyword
In Python, the assert keyword is used to indicate an assert statement or an assertion about an expression. An assert statement returns a no-op if the expression (expr>) is true, and an AssertionError if the expression is false. Use assert followed by an expression to define an assertion:
assert <expr>
In general, assert statements are used to ensure that anything that must be true is true. However, you should not rely on them because they may be ignored depending on how your Python program is executed.
Keywords for parallel programming: async, await
Asynchronous programming is a complicated subject. Async and await are two Python keywords that can assist make asynchronous programming more understandable and cleaner.
The parts that follow will teach the two asynchronous keywords and their fundamental syntax, but they will not delve into detail on asynchronous programming. Check out Async IO in Python: A Complete Walkthrough and Getting Started With Async Features in Python to learn more about asynchronous programming.
async Keyword
The async keyword is used in conjunction with def to define an asynchronous function, often known as a coroutine. The syntax is identical to that of declaring a function, with the addition of async at the start:
async def <function>(<params>):
<statements>
A function may be made asynchronous by using the async keyword before its usual declaration.
await Keyword
In asynchronous functions, the await keyword is used to identify a point in the function where control is returned to the event loop for other functions to perform. You may utilize it by putting the await keyword in front of any async function call.
await <some async function call>
# OR
<var> = await <some async function call>
When using await, you have two options: call the asynchronous function and ignore the results, or store the results in a variable when the function returns.
Keywords for variable manipulation: del, global, nonlocal
To operate with variables in Python, three keywords are needed. The del keyword is far more popular than the global and nonlocal keywords. However, knowing and understanding all three keywords is still beneficial so you can identify when and how to use them.
del Keyword
In Python, del is used to unset a variable or name. It may be used on variable names, but its most typical application is to delete indexes from a list or dictionary. To unset a variable, type del followed by the variable name:
del <variable>
Assume you want to clean up a dictionary you obtained from an API response by removing keys you know you won't use. You may accomplish this by using the del keyword:
>>> del response["headers"]
>>> del response["errors"]
The "headers" and "errors" keys will be removed from the dictionary response.
global Keyword
You must use the global keyword to alter a variable that is not declared in a function but is defined in the global scope. This works by indicating in the function which variables from the global scope should be fetched into the function:
global <variable>
A simple example is using a function call to increase a global variable. You may accomplish this using the global keyword:
>>> x = 0
>>> def inc():
... global x
... x += 1
...
>>> inc()
>>> x
1
>>> inc()
>>> x
2
This is not typically considered good practice, although it has its purposes. Check out Python Scope & the LEGB Rule: Resolving Names in Your Code for more information on the global keyword.
nonlocal Keyword
The nonlocal keyword, like global, allows you to edit variables from a separate scope. The scope you're pulling from with global is the global scope. The scope you're fetching from with nonlocal is the parent scope. The syntax is comparable to global:
nonlocal <variable>
This keyword isn't used frequently, although it can come in helpful at times. Python Scope & the LEGB Rule: Resolving Names in Your Code has further information on scoping and the nonlocal keyword.
Python obsolete Keywords
A Python keyword can sometimes become a built-in function. This was true for both print and executive. In version 2.7, these were Python keywords, but they have since been changed to built-in functions.
Former print Keyword
When print was a keyword, the syntax for printing something to the screen was as follows:
print "Hello, World"
It looks like a lot of the other keyword statements, with the keyword followed by the arguments.
Print is no longer a keyword, and printing is done with the built-in print() method. To print something to the screen, use the following syntax:
Former exec Keyword
In Python 2.7, the exec keyword took a string of Python code and executed it. This was accomplished using the following syntax:
exec "<statements>"
The same behavior may be obtained in Python 3+ by using the built-in exec() function. For example, if you wanted to execute "x = 12 * 7" in Python code, you could perform the following:
>>> exec("x = 12 * 7")
>>> x == 84
True
For additional information about exec() and its applications, see How to Run Your Python Scripts and Python's exec(): An Introduction. Run code generated dynamically.
Conclusion
Python keywords serve as the foundation of every Python program. Understanding how to utilize them correctly is critical to increasing your Python abilities and understanding.
You've seen a few things in this post to help you consolidate your grasp of Python keywords and develop more efficient and understandable code.
You've learned the following in this article:
- Python keywords in version 3.8 and their fundamental usage
- Several resources to help you better understand many of the keywords
- How to programmatically work with keywords using Python's keyword module