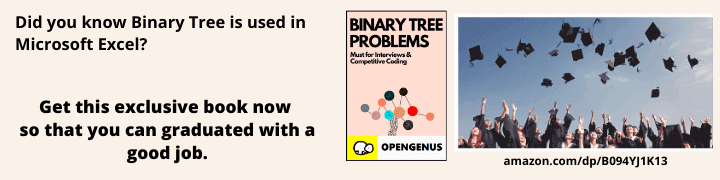
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
malloc is a C++ library function that allocates the requested memory and returns a pointer to it. This is called dynamic memory allocation and allows for memory allocation on the go. It is the predecessor to the c++ new operator used for memory allocation.
The number of bytes to allocate is passed as the single parameter. On success the function returns a pointer to the start of the allocated memory or return a null pointer upon failure.
Syntax
void *malloc (size_t size)
Return type is a pointer to the start of the allocated memory. Or null pointer on failure
Parameter is number of bytes to allocate
Note
After usage of the pointer is done, free() function is called by passing the returned pointer. This deallocates the assigned section of memory and avoid memory leakage.
Example:
#include <stdio.h>
#include <stdlib.h>
int main(){
char *str;
//call malloc and cast to string before adding "Man The Guns!" to 'str'
str = (char *) malloc(15);
strcpy(str, "Man The Guns!");
printf("String = %s, Address = %u\n",str,str);
//to increase the size of string realloc is used. We now add string ". Now What?" to the string.
str =(char *)realloc(str,25);
strcat(str," Now What?");
printf("String = %s, Address=%u\n",str,str);
//Finally, we call free up the memory allocated by calling 'free()'
free(str);
return 0;
}
malloc, free and realloc
Malloc function is used with free() and realloc() to handle safe dynamic memory allocation. Although in modern c++ applications new and delete operators are recommended.
Reasons to use new over malloc():
-
Error handling, since malloc() does not throw errors. You need to check for null value instead.
-
malloc() is not type safe, if you look at the example code your see that we need to cast the returned value to get usable data.
-
malloc() is not object oriented. Hence mixing malloc() and new in your c++ code makes it error prone.
Only few cases require the specific use of malloc() as an alternative to the new operator. Such as the reallocating data size which the new operator cannot do.
Question
#include <stdio.h>
#include <stdlib.h>
int main(){
char *str;
str = (char *) malloc(15);
strcpy(str, "Man The Guns!");
printf("String = %s, Address = %u\n",str,str);
free(str);
str =(char *)malloc(5);
strcpy(str," What?");
printf("String = %s, Address=%u\n",str,str);
free(str);
return 0;
}
- What are the String = outputs?
- Will Address be same value?
With this article at OpenGenus, you must have a good knowledge on malloc() in C++.