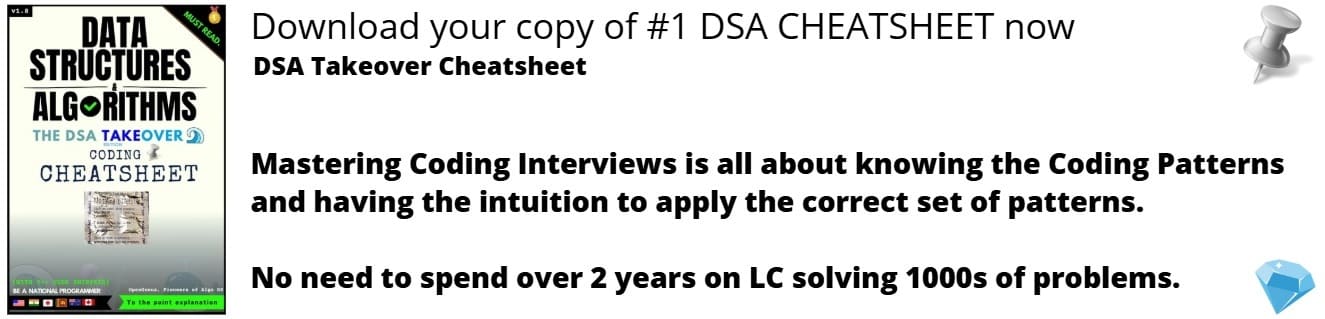
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
The various types of Garbage Collection events cleaning out different parts inside heap memory are:
-
Minor garbage collection
-
Major garbage collection
-
Full garbage collection
Let us recall the high-level memory organization to aid us moving forward:
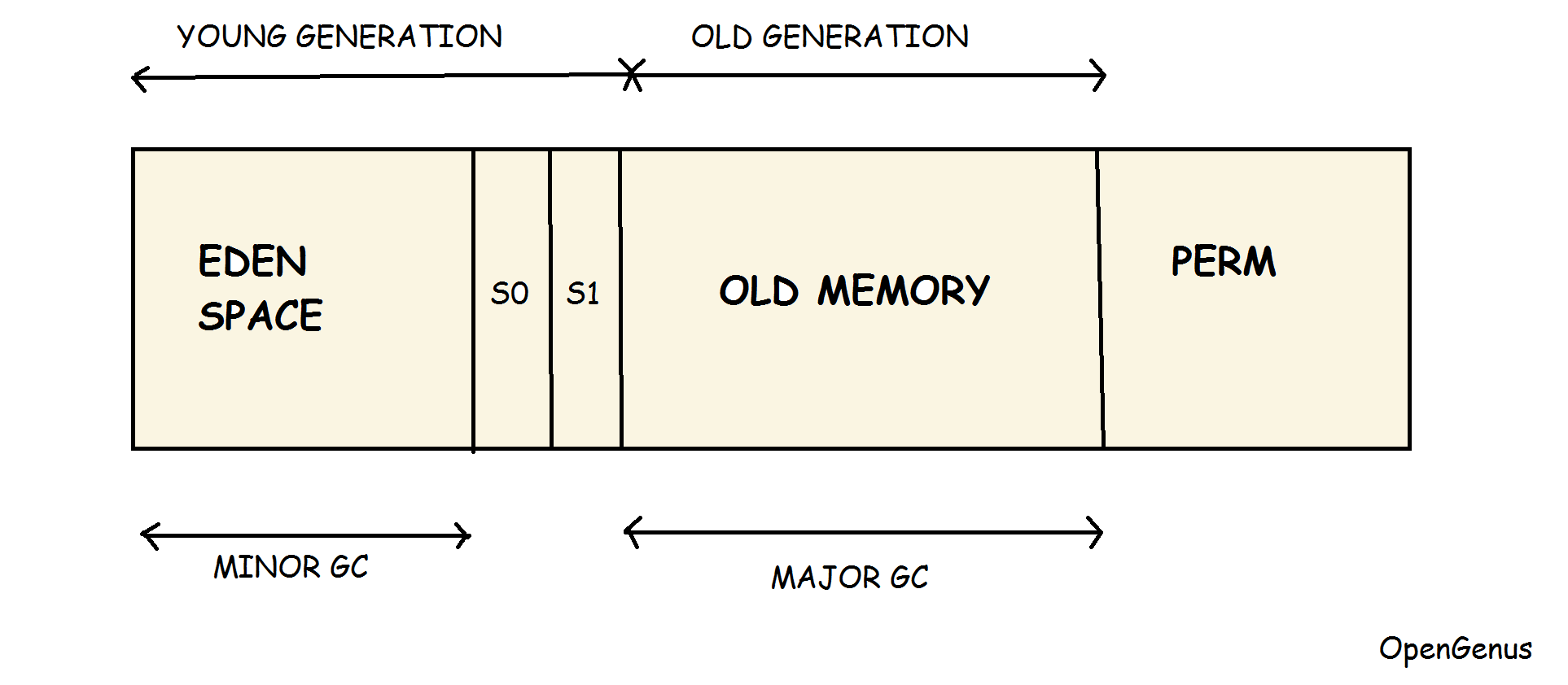
Note: Objects stored in Young generation memory space can have references to objects in the Young generation memory space as well as the Old generation memory space. Same is applicable to objects stored in the Old generation memory space.
Minor garbage collection
Collecting garbage from the Young generation memory space is called Minor garbage collection.
Key points regarding Minor garbage collection are:
- Minor garbage collection is always triggered when the Java Virtual Machine (JVM) is unable to allocate space for a new object; for instance: Eden memory space is full.
The higher the allocation rate, the more frequently Minor garbage collection occurs.
-
During a Minor garbage collection event, Old Generation memory space is effectively ignored.
- References from Old Generation to Young Generation are considered to be garbage collection roots.
- References from Young Generation to Old Generation memory space are simply ignored during the mark phase.
-
Minor GC does trigger stop-the-world pauses, suspending the application threads. For most applications, the length of the pauses is negligible latency-wise if most of the objects in the Eden can be considered garbage and are never copied to Survivor/Old spaces. If the opposite is true and most of the newborn objects are not eligible for collection, Minor garbage collection pauses start taking considerably more time.
In short, Minor garbage collection cleans the Young Generation memory space.
Major garbage collection
Note: There are no formal definitions for these terms in the JVM specification or the Garbage Collection research papers.
Major garbage collection cleans the Old generation memory space.
Key points regarding Major garbage collection are:
-
Major garbage collection only takes a look at the Old generation memory space.
-
Major garbage collection does trigger stop-the-world pauses, suspending the application threads.
Full garbage collection
Full garbage collection cleans the entire Heap โ both Young and Old spaces. It, also, cleans the permanent memory space.
Key points regarding Full garbage collection are:
-
Full garbage collection takes a look at the Young generation, Old generation and Permanent memory space.
-
Full garbage collection does trigger stop-the-world pauses, suspending the application threads.
Analysis
The real scenario is a bit more complex and confusing.
Two deep real-life key ideas:
-
Many Major garbage collections are triggered by Minor garbage collections, so separating the two is impossible in many cases.
-
Modern garbage collection algorithms like G1 perform partial garbage cleaning so, again, using the term โcleaningโ is only partially correct.
This leads us to the point where focus shifts from the quest of identifying whether the garbage collection is Major or Full garbage collections to focusing on finding out whether the garbage collection stopped all the application threads or was able to progress concurrently with the application threads.
Consider this small snippet of garbage collection analysis on a Java Virtual Machine running with Concurrent Mark and Sweep collector (-XX:+UseConcMarkSweepGC) using GCViewer:
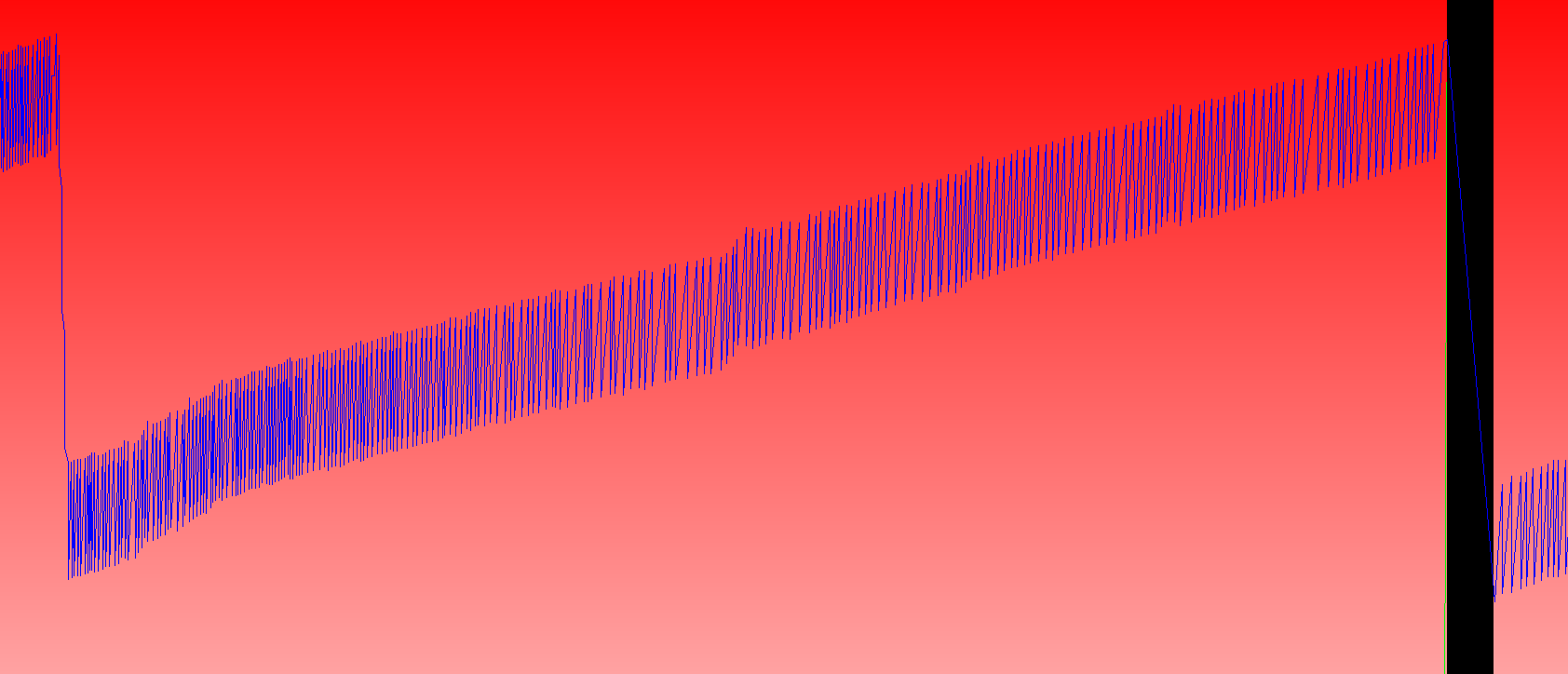
Visual observations:
-
There are "small zigzag" of the blue lines
-
There is the black bar with the corresponding garbage collection log entry is:
1751585.394: [Full GC 1433326K->660045K(1552832K), 10.1157600 secs]
-
There are two big drops of the blue line on the left and right with the corresponding garbage collection log entry being:
1749795.648: [GC 1299871K(1552832K), 0.0402933 secs]
Explanation of the above analysis image:
The "small zig-zags" are minor garbage collections in the young generation memory space.
The black bar is a full garbage collection.
The young generation is divided into an "Eden" space one or more "survivor" spaces. During one of those small zig-zags, dead objects in the Eden space are removed, and live objects are moved to one of the survivor spaces. This is a very quick operation, because the weak generational hypothesis states that most objects will be short-lived. A subsequent sweep of the survivor space may move objects that are still alive from the survivor space into the tenured generation. This is still considered a minor collection.
The big drop is a "promotion" from the survivor generations to the old generation.
The tenured generation is everything that is not young enough to be in the young generation. The tenured generation is much larger than the young generation, and therefore takes a lot of time to sweep. Full garbage collection refers to a sweep of the tenured generation.
The CMS garbage collector operates concurrently but it needs to stop the world for the mark operations on the young and tenured generations. The CMS collector is supposed to be very quick at this, even for large heaps. However, there's one other situation where CMS stops the world: concurrent mode failure.
The second big drop with the pause is a result of this, which occurs when the old generation is too full. We can adjust the size of the old generation and other parameters to avoid these.