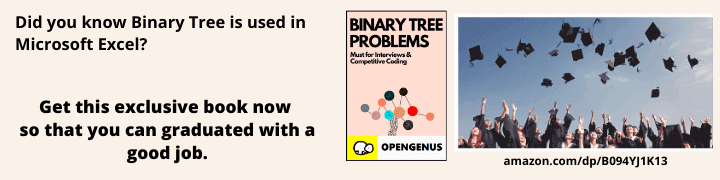
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
In this article, we will be going to solve a problem named convert octal to decimal. This is a very basic problem of computer science involving ideas of base in number system and conversion.
Tables of contents:
- Introduction to Problem Statement
- Properties of the Octal
- Rules for octal to Decimal conversion
- Tracing a Few Sample Examples
- Implementing the Solution in C++
- Explanation of C++ code
- Time and Space Complexity
Introduction to Problem Statement
In this problem, the octal form of a number is given and we have to convert the octal form to decimal form.
Example: like the octal form a number=30 (this is given)
After converting this into decimal we get the number= 18
Input : 30 //octal
Output : 18 //decimal
Input: 214 //octal
Output: 140 //decimal
Input: 1732 //octal
Output: 986 //decimal
Properties of the Octal
First of all, we will see that, what is the octal number?
The octal number system is one of the forms of representing a number with base-8. That means the representation of octal number consists of only the first 8 numerals i.e. 0, 1, 2, 3, 4, 5, 6, 7.
But in decimal representation, we denote the numbers with all 10 numerals i.e. 0, 1, 2, 3, 4, 5, 6, 7, 8, 9.
The octal number is represented with base 8. Example: (24)base(8) etc.
The octal and decimal forms of the first 10 numbers are:
Decimal Octal
0 0
1 1
2 2
3 3
4 4
5 5
6 6
7 7
8 10
9 11
10 12
Rules for octal to Decimal conversion
For converting the octal form to the decimal form, the following rules should be followed :
-
Multiply each digit of the number with the increasing power of 8 (starting with 0 to number of digits-1 i.e. 8^0, 8^1, 8^2, 8^3, 8^4, ......, 8^(number of digit-1) ) from the right side.
-
Sum all the multiples obtained.
-
The resultant sum is the decimal form of that given octal number.
Tracing a Few Sample Examples
#Sample 1
Input: 214 //Octal form
Output: 140 //Decimal form
Explanation : 214
4*(8^0) = 41 = 4
1(8^1) = 18 = 8
2(8^2) = 2*64 = 128
Sum= 4 + 8 + 128 = 140
=> The Decimal representation of the 214 = 140
#Sample 2
Input: 1732 //Octal form
Output: 986 //Decimal form
Explanation : 1732
2*(8^0) = 21 = 2
3(8^1) = 38 = 24
7(8^2) = 764 = 448
1(8^3) = 1*512 = 512
Sum= 2 + 24 + 448 + 512 = 986
=> The Decimal representation of the 1732 = 986
#Sample 3
Input: 179 //Octal form
Output: Invalid Input
Explanation: We know that the octal representation of any number contains only the first eight numerics i.e. 0, 1, 2, 3, 4, 5, 6, 7. But the given input number contains a digit 9 which should not be in octal representation. So, the given Input is Invalid.
#Sample 4
Input: 81 //Octal form
Output: Invalid Input
Explanation : Exlained in Sample 3.
Implementing the Solution in C++
For the implementation of converting octal to decimal, we shall implement using C++.
> 1 #include <iostream>
> 2 using namespace std;
> 3
> 4
> 5 int ConvertOctaltoDecimal ( int octal ) {
> 6 int decimal = 0 , position =0 ;
> 7 while ( octal > 0 ) {
> 8 int temp = octal % 10 ;
> 9 decimal = decimal + temp * pow( 8 , position );
> 10 octal = octal / 10 ;
> 11 position = position + 1 ;
> 12 }
> 13 return decimal ;
> 14 }
> 15
> 16
> 17 int CheckOctal ( int octal ) {
> 18 while ( octal > 0 ) {
> 19 int temp = octal % 10 ;
> 20 if ( temp == 8 || temp ==9 ) {
> 21 return 0 ;
> 22 }
> 23 octal = octal / 10 ;
> 24 }
> 25 return 1;
> 26 }
> 27
> 28
> 29
> 30 int main() {
> 31 int octal;
> 32 cin >> octal;
> 33
> 34 int check = 0 ;
> 35 check = CheckOctal ( octal );
> 36 if( check == 0 ){
> 37 cout << "Invalid Input" ;
> 38 }
> 39 else {
> 40 int decimal = 0;
> 41 decimal = ConvertOctaltoDecimal ( octal ) ;
> 42 cout << decimal ;
> 43 }
> 44 return 0 ;
> 45 }
> 46
Explanation of C++ code
Line 1-2 Include the required library
Line 30-45
In line 31 define a number and, in line 32 we take input octal number which we have to convert it to decimal
From lines 34-38 we check the octal number is valid or not with a function CheckOctal. If it is not valid as discussed in sample-3 & sample-4, we print Invalid Input.
Else ( from line 39- 42) if it is a possible octal number then we convert that into a decimal number by using function ConvertOctaltoDecimal and print the decimal form of a given octal number.
Line 17-26
Function check possible octal number or not. In a while loop, for every digit check whether it is 8 or 9 if it is. Then, return 0. If not then continue the loop and check the next digit. If no digit detected 8 or 9 then lastly return 1 means possible.
Line 5-14
ConvertOctaltoDecimal function convert the octal number to decimal form. In line 6, decimal calculates the final decimal number and position detects the position of the digit starting from 0. In the while loop, every time temp calculates the last digit of octal and finally we add the required product with temp and power of 8 as discussed in sample-1, sample-2. Lastly, we divide octal by 10 so that every time we got temp as the next digit. And we increase the position by 1 so that we multiply next time with higher power of 8.
Time and Space Complexity
let n = the number of digits in the octal form of the number.
The time complexity of the written C++ code is O( n ).
And the Space complexity of the written C++ code is O( 1 ).
As in ConvertOctaltoDecimal and CheckOctal function, we run one while loop which runs n times in both the functions. So, time complexity will be O( n ).
In the worst and best case the time complexity will be O( n ).
As in the whole code, we define only int not an array or another data structure that takes constant space. So, the space complexity will be O( 1 ) in all cases.
With this article at OpenGenus, you must have the complete idea of how to Convert Octal to Decimal.