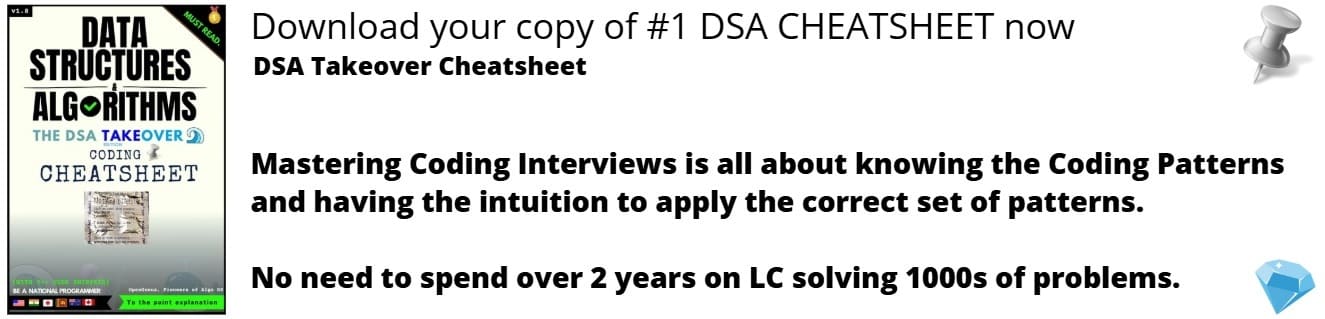
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
Table of Contents
- Prerequisites
- Introduction
- The Contact class
- The ContactBook class
- Putting it all together - The ContactBookClient class
- Summary
- Key Concepts
- Conclusion
Prerequistes
This OpenGenus article is intended for those who have knowledge of basic Java syntax such taking input/output from the user using Scanner, data types and variables, loops and conditions, classes and objects, exception handling and ArrayList and are ready to build their first project using object-oriented programming.
Tools
Installation of JDK 17 or higher and an IDE of your choice.
Introduction
In this OpenGenus article, you will learn how to apply the basic concepts of object-oriented programming (OOP) to develop a simple, console-based contact book application. This application will allow users to store, view, edit, and manage a list of contacts efficiently using core OOP principles such as classes, objects, encapsulation, and methods.Your application will be have the following features:
Add a contact: Users can add a new contact by providing the name, phone number, and email.
View contacts: Users can view the list of contacts stored in the Contact Book.
Edit a contact: Users can edit the details of an existing contact, including the name, phone number, and email.
Delete a contact: Users can delete a contact from the Contact Book.
In this article we will follow the principle of incremental development where I will give you some boilerplate code so that you can implement the code according to the directions. Please try writing the code yourself before looking at my implementation.
The Contact Class
Object-oriented programming (OOP) is a paradigm that uses objects to represent real-world entities and their interactions, helping us design more organized and maintainable applications. For example, consider the contacts list on your iPhone: each contact has attributes like name, email, and phone number. You can also perform actions such as adding, editing, and deleting contacts. We'll use OOP principles to model this relationship in our code. Let's start implementing our Contact
class, which represents a single contact or the most basic unit of our contact book.
Defining Instance Variables
public class Contact {
//define instance variables here
}
Answer
public class Contact {
private String name;
private String phoneNumber;
private String email;
}
In Java, it is best practice to keep instance variables private so that they cannot be modified from other classes.
Initializing our Contact object with a constructor
public Contact(String name, String phoneNumber, String email) {
//initialize instance variables here
}
Answer
public Contact(String name, String phoneNumber, String email) {
this.name = name;
this.phoneNumber = phoneNumber;
this.email = email;
}
A constructor in Java is a special method that is called when you create a new object of a class. It initializes the object and sets up its initial state. The keyword this is a reference to the current object (the object whose constructor is being called). It's used to distinguish between instance variables and parameters or other variables with the same name.
Key points:
- It has the same name as the class.
- It doesn't have a return type, not even void.
- It's used to assign values to the object's variables when the object is created
Getters and Setters
Getters and setters in Java help with encapsulation by keeping a class's variables private and controlling how they are accessed or changed. Getters let you read the value, while setters allow you to update it safely, ensuring the data stays valid and secure. This keeps the internal details of the class hidden from outside code. Let's add our getters and setters for the Contact
class.
public String getPhoneNumber() {
//your implementation here
}
public String getName() {
//your implementation here
}
public String getEmail() {
//your implementation here
}
public void setPhoneNumber(String phoneNumber) {
//your implementation here
}
public void setName(String name) {
//your implementation here
}
public void setEmail(String email) {
//your implementation here
}
Answer
public String getPhoneNumber() {
return this.phoneNumber;
}
public String getName() {
return this.name;
}
public String getEmail() {
return this.email;
}
public void setPhoneNumber(String phoneNumber) {
this.phoneNumber = phoneNumber;
}
public void setName(String name) {
this.name = name;
}
public void setEmail(String email) {
this.email = email;
}
Equals Method
We'll use this method to compare whether 2 contacts have the same name, email, and phone number in order to avoid adding duplicate contacts to our contact book.
public boolean equals(Contact contact) {
//your implementation here
}
Answer
public boolean equals(Contact contact) {
return this.name.equals(contact.email)
&& this.email.equals(contact.name)
&& this.phoneNumber.equals(contact.phoneNumber); //use .equals to compare strings
}
toString() Method
Java's default toString()
method returns a string that represents the object's memory address, which isn't very useful when we want to display meaningful information about an object. By overriding the default toString()
method in our class, we can customize how an object is represented as a string. This is important for providing clear and readable output when viewing the details of an object, such as a contact list.
public String toString() {
//your implementation here
}
Answer
public String toString() {
String name = "Contact name: " + this.name + "\n";
String email = "Contact email: " + this.email + "\n";
String phoneNumber = "Contact phone number: " + this.phoneNumber + "\n";
return name + email + phoneNumber;
}
The ContactBook class
Now that we've defined the attributes of the Contact object, we need to implement the methods that will let users view, add, edit, and delete contacts. To manage these operations, we'll create a new class called ContactBook. This class will serve as a collection and manager for our contacts.
ArrayList of Contacts
This is where we'll store the contact information. Since an ArrayList can hold objects of any type, we'll initialize it to store Contact objects specifically.
public class ContactBook {
//initialize ArrayList here
}
Answer
private ArrayList list = new ArrayList();
Again, we want to make our instance variables private to follow the principle of encapsulation.
View contact list
Prints out all our entries in our contact book along with each corresponding entry number. Hint: Can you use a method from the Contact class to display Contact info?
public void view() {
System.out.println("\nViewing all Contact Book entries");
//your implementation here
}
Answer
public void view() {
System.out.println("\nViewing all Contact Book entries");
for (int i = 0; i < list.size(); i++) {
System.out.println("-----------");
System.out.println("Entry #" + (i + 1));
System.out.println(list.get(i).toString());
}
}
Add a contact
Checks if the contact already exists in the contact book. If so, inform the user that they are trying to add a duplicate contact. Otherwise, add an instance of Contact
to our contact book. Hint: Can you use a method from the Contact class to check for duplicates?
public void add(String name, String email, String phoneNumber) {
Contact contact = new Contact(name, email, phoneNumber);
//your implementation here
}
Answer
public void add(String name, String email, String phoneNumber) {
Contact contact = new Contact(name, email, phoneNumber);
for (Contact c : list) {
if (c.equals(contact)) {
System.out.println("\nContact with phone number, name, and " + "email already exists in contact book");
return;
}
}
list.add(contact);
int entryNum = list.indexOf(contact) + 1;
System.out.println("Entry # " + entryNum + " successfully added to contact book!\n");
}
For editing and deleting contacts we will prompt the user for the entry that they would like to edit or delete in the main method.
Edit contact
Retrives and prints out information about the contact. Prompt the user to change the contact's name, email, and phone number and set them to the new values in our Arraylist
.
public void edit(int entry) {
int index = entry - 1;
//get the contact from the array list
System.out.println("\nEntry #" + (entry));
//print contact information
Scanner s = new Scanner(System.in);
System.out.print("\nEdit name: ");
//read user input and set name of contact
System.out.print("Edit Email: ");
//read user input and set email of contact
System.out.print("Edit phone number: ");
//read user input and set phone number of contact
System.out.println("\nContact edited successfully!");
}
Answer
public void edit(int entry) {
int index = entry - 1;
Contact contact = list.get(index);
System.out.println("\nEntry #" + (entry));
System.out.println(contact.toString());
Scanner s = new Scanner(System.in);
System.out.print("\nEdit name: ");
String name = s.nextLine();
contact.setName(name);
System.out.print("Edit Email: ");
String email = s.nextLine();
contact.setEmail(email);
System.out.print("Edit phone number: ");
String phoneNumber = s.nextLine();
contact.setPhoneNumber(phoneNumber);
System.out.println("\nContact edited successfully!");
}
Delete contact
Removes the entry from the ArrayList
. Hint: Java ArrayList's use 0 based indexing, meaning that the first element in the ArrayList will have an index of 0.
public void delete(int entry) {
//your implementation here
System.out.println("\nEntry #" + entry + " sucessfully deleted!");
}
Answer
public void delete(int entry) {
int index = entry - 1;
list.remove(index);
System.out.println("\nEntry #" + entry + " sucessfully deleted!");
}
Putting it all together - The ContactBookClient class
Now that we've finished writing the code for the Contact
and Contact Book
classes, we are ready to implement our main method (ContactBookClient class) that allows the user to interact with our contact book program through the console. Once again, I will provide you with boilerplate code. I recommend you run the provided code first to get an idea of the expected output.
You can either write your own unit tests or run your program in the console to verify the output is correct. My full implementation is provided in the GitHub repo linked at the bottom of this article.
ContactBookClient.java
import java.util.InputMismatchException;
import java.util.Scanner;
public class ContactBookClient {
private static Scanner s = new Scanner(System.in);
public static void main(String[] args) {
ContactBook contactBook = new ContactBook();
int userInput;
do {
displayMenu();
userInput = getValidMenuOption();
switch (userInput) {
case 1:
if (contactBook.getSize() != 0) {
//your code here
} else {
System.out.println("There are no contacts to view.");
}
break;
case 2:
//your code here
break;
case 3:
if (contactBook.getSize() != 0) {
//your code here
} else {
System.out.println("There are no contacts to edit.");
}
break;
case 4:
if (contactBook.getSize() != 0) {
//your code here
} else {
System.out.println("There are no contacts to delete.");
}
break;
case 5:
System.out.println("Exiting program...");
break;
default:
System.out.println("Invalid option.");
}
} while (userInput != 5);
s.close();
}
// Displays the menu options
private static void displayMenu() {
System.out.println("Contact Book Menu:");
System.out.println("1 - View contacts");
System.out.println("2 - Add a new contact");
System.out.println("3 - Edit a contact");
System.out.println("4 - Delete a contact");
System.out.println("5 - Quit");
System.out.print("Choose an option: ");
}
// Gets a valid menu option between 1 and 5
private static int getValidMenuOption() {
int userInput = -1;
boolean inputValid = false;
do {
try {
userInput = s.nextInt();
if (userInput >= 1 && userInput <= 5) {
inputValid = true;
} else {
System.out.println("Invalid input. Please enter a number between 1 and 5.");
}
} catch (InputMismatchException e) {
System.out.println("Invalid input. Please enter a valid number.");
s.next(); // Clear invalid input
}
} while (!inputValid);
s.nextLine(); // Consume leftover newline
return userInput;
}
// Adds a new contact to the contact book
private static void addContact(ContactBook contactBook) {
System.out.print("Enter contact name: ");
//read user input
System.out.print("Enter contact email: ");
//read user input
System.out.print("Enter contact phone number: ");
//read user input
//add name, phone number, and email to contact book
System.out.println("Contact added successfully.");
}
// Edits a contact from the contact book
private static void editContact(ContactBook contactBook) {
//process user input
//edit the contact's information
System.out.println("Contact edited successfully.");
}
// Deletes a contact from the contact book
private static void deleteContact(ContactBook contactBook) {
//process user input
//delete the contact from the contact book
System.out.println("Contact deleted successfully.");
}
//Processes user input to choose a valid entry from the contact book
public static int processUserInput(ContactBook contactBook) {
//implement this method following the same pattern as used in getValidMenuOption()
//if the input is invalid print a message to inform the user
int userInput = -1;
boolean inputInvalid;
do {
System.out.print("Choose an entry (1 to " + contactBook.getSize() + "): ");
try {
//your code here
}
} catch (InputMismatchException e) {
//your code here
}
} while (inputInvalid);
s.nextLine(); // Consume leftover newline
return userInput;
}
Summary
In this article, I have demonstrated object-oriented programming (OOP) in action. By developing a simple contact book program, I hope you’ve gained a clearer understanding of the core concepts of OOP and how they can be applied in real-world programming.
Key concepts
Class: A blueprint or template for creating objects. It defines the properties (fields) and behaviors (methods) that objects of that class will have.
Object: An instance of a class, representing a specific entity with its own set of values for the defined properties and methods.
Instance variable: A variable defined in a class for which each instantiated object (instance) of the class has its own copy.
Static: Describes a class member that belongs to the class itself rather than to any specific instance. Static members can be accessed directly using the class name, without needing to create an object first. Note that you cannot access non-static members from a static context.
Public: An access modifier that allows a class, method, or variable to be accessible from any other class.
Private: An access modifier that restricts access to a class's variables or methods to only within that class.
Encapsulation: The OOP principle of keeping data (variables) and methods together in a class and restricting direct access to some components using access modifiers like private.
Constructor: A special method in a class that is used to initialize new objects. It often sets the initial values of instance variables.
Getter: A method that retrieves or "gets" the value of a private instance variable.
Setter: A method that updates or "sets" the value of a private instance variable.
toString Method: A method that converts an object into a string representation, often for printing or debugging purposes.
equals Method: A method that compares two objects to determine if they are logically equal based on their content, rather than their memory addresses.
Resources
Complete Implementation: https://github.com/skottchen/ContactBook