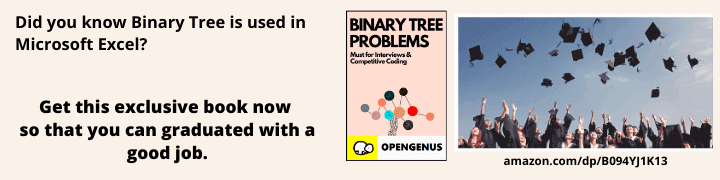
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
In this article, we have take a look at how to print a triangular pattern (right angle triangle) with integers 1 to 15 in C Programming Language.
Following is the pattern:
1
2 3
4 5 6
7 8 9 10
11 12 131415
Table of contents
- Problem statement
- Approach to solve
- Implementation
- Output
Problem statement
In this problem, we have to print the following triangular pattern:
1
2 3
4 5 6
7 8 9 10
11 12 131415
Note the last line where 13, 14 and 15 are printed without any space.
Approach to solve the problem
Following is the approach to solve this problem:
-
For this program we need to run two for loops, one for the rows and another for the columns. First we will declare i for row, j for column and another variable a and initialise it with a value 1. The outer loop will run for the row and inner one for the column. The outer loop will run from 5 and will decrease by 1 till it reaches 1. The inner loop will run from 5 till the value of i and will keep decreasing by 1. Then we print a and increaase the value of a by 1.
-
First i=5, it satisfies the condition that i>=1 so it enters the inner loop. For j=5 it also satisfies the condition of j>=i so it prints the value of a i.e. 1 and then value of a is incremented by 1. Now j=4 but since it doesn't satisfy the condition of J>=i where i=5 it exits the loop and the cursor goes to the next line as there is a new line statement outside the inner loop.
-
Next i=4, it satisfies the condition that i>=1 so it enters the inner loop. For j=5 it also satisfies the condition of j>=i so it prints the value of a i.e. 2 and then value of a is incremented by 1. Now j=4 which also satisfies the condition of j>=i where i=4 so prints 3 on the same line.Now j=3 but since it doesn't satisfy the condition of J>=i where i=4 it exits the loop and the cursor goes to the next line. This continues till i=1 and we get the required pattern.
Implementation
Following is the implemention of the code in C programming language:
#include<stdio.h>
int main()
{
int i,j,a=1;
for(i=5;i>=1;i--)
{
for(j=5;j>=i;j--)
{
printf("%2d",a);
a++;
}
printf("\n");
}
return 0;
}
Output
Run the code as follows:
gcc code.c
./a.out
Following is the output of the program:
1
2 3
4 5 6
7 8 9 10
11 12 131415
With this article at OpenGenus, you must have the complete idea of how to print the triangular pattern in C Programming Language.