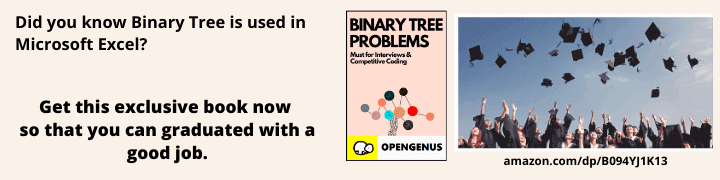
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
In this article at OpenGenus, we will examine 8 different ways to get random numbers in Python.
Table of Contents:
- Introduction
- The random Module
- Generating Random Numbers within a Range
- Generating Random Floats
- Generating Random Integers
- Random Sampling from a List
- Random Selection from a String
- Random Shuffling
- Cryptographically Secure Random Numbers
- Conclusion
Introduction
Numerous computer applications, including games, simulations, and encryption, depend heavily on random numbers. Python, a powerful programming language, offers a number of fast ways to produce random integers. In this article at OpenGenus, we will examine various methods for obtaining random numbers in Python and discover practical applications for them.
The 8 different ways to get a random number in Python are:
- The random Module
- Generating Random Numbers within a Range
- Generating Random Floats
- Generating Random Integers
- Random Sampling from a List
- Random Selection from a String
- Random Shuffling
- Cryptographically Secure Random Numbers
The random Module
A variety of functions are available in the built-in random
module of Python to produce random numbers. We must import the module first before we can utilize these functions:
import random
Generating Random Numbers within a Range
We often need random numbers that fall inside a certain range. The randrange(start, stop, step)
function is offered for this purpose by the random
module. Between start
(inclusive) and stop
(exclusive), it creates a random number, incrementing by step
:
import random
random_number = random.randrange(1, 100, 2)
print(random_number)
Sample output
59
The code above produces an odd number at random between 1 and 99.
Generating Random Floats
Using the random()
function of the random
module, we can get random floating-point values between 0 and 1:
import random
random_float = random.random()
print(random_float)
This code snippet generates a random float value between 0 and 1.
0.8765219845692345
Generating Random Integers
The randint(a, b)
function in the random
module also produces random numbers between a
and b
(inclusive):
import random
random_integer = random.randint(1, 10)
print(random_integer)
The code above generates a random integer between 1 and 10.
7
Random Sampling from a List
We might occasionally need to choose random items from a list. The sample(sequence, k)
function is provided for this by the random
module. It extracts k
distinct random items at random from the supplied sequence
:
import random
my_list = [1, 2, 3, 4, 5]
random_selection = random.sample(my_list, 3)
print(random_selection)
The output will be three random elements from the list.
[3, 2, 5]
Random Selection from a String
The choice(sequence)
function from the random
module may be used to choose a random character from a string as well:
import random
my_string = "Hello, World!"
random_character = random.choice(my_string)
print(random_character)
Executing the above code will print a random character from the string.
r
Random Shuffling
The shuffle(sequence)
method in Python's random
module enables us to shuffle a list at random:
import random
my_list = [1, 2, 3, 4, 5]
random.shuffle(my_list)
print(my_list)
Running the code above will shuffle the elements of the list randomly.
[3, 2, 5, 1, 4]
Cryptographically Secure Random Numbers
Use of cryptographically safe random numbers is crucial when working with security-sensitive applications. The following features are offered by the Python 3.6-added secrets
module:
import secrets
secure_random_number = secrets.randbelow(100)
print(secure_random_number)
The randbelow(n)
function creates a random number between 0 and n
(exclusively) that is cryptographically safe.
73
Conclusion
Random number generation is a common necessity in many programming activities. The random
module in Python provides a wide range of methods to effectively create random integers. The secrete
module was used to create random numbers that were cryptographically secure, as well as random floats and integers, samples from lists and strings, random shuffles of sequences, and random floats and integers within predetermined ranges. Utilizing these methods, programmers may add randomization to their apps, giving them more variety and unpredictability.