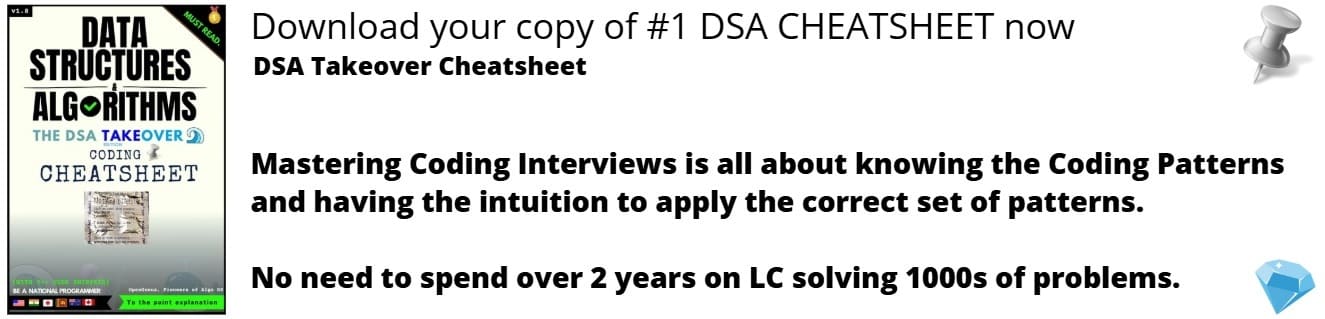
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
In this article, we will see different ways a string can be splitted in C++. This involve the use of find(), substr() and much more.
Breaking or Fragmenting a String in a number of words is called Splitting of string. There is no predefined function to split a string into number of substrings so we will discuss a number of ways in which we can do the spitting
Some Methods of Splitting a String in C++
1. Using find() and substr() Functions
Using this method we can split the string containing delimiter in between into a number of substrings.
Delimiter is a unique character or a series of characters that indicates the beginning or end of a specific statement or string. These delimiter need not to be a void space only , that can be any character or a group of character.
C++ Program
#include <bits/stdc++.h>
using namespace std;
void splitstr(string str, string deli = " ")
{
int start = 0;
int end = str.find(deli);
while (end != -1) {
cout << str.substr(start, end - start) << endl;
start = end + deli.size();
end = str.find(deli, start);
}
cout << str.substr(start, end - start);
}
int main()
{
string s = "This&&is&&an&&Article&&at&&OpenGenus"; // Take any string with any delimiter
splitstr(s, "&&");
cout << endl;
return 0;
}
Output
This
is
an
Article
at
OpenGenus
In this Program, we are using find() function inside the while loop to find the occurance of Delimiter repeatedly and every time we find the delimiter we use substr() function to print the substring and then we point start variable at the end of the last substring printed and then again find Delimiter and print the substring . This process continues till we find all the substring
2. Using custom splitStr() function
C++ Program
#include <bits/stdc++.h>
using namespace std;
void SplitStr(string str)
{
string s = "";
cout<<"The split string is:"
for (auto x : str)
{
if (x == ' ')
{
cout << s << endl;
s = "";
}
else {
s = s + x;
}
}
cout << s << endl;
}
int main()
{
string str = "Opengenus Article to Split the String";
SplitStr(str);
return 0;
}
Above is the code for splitting the String using our Custom splitstr() function
The step wise execution of this code is as follows
- Initializing the string str and calling the splitSrt() function ,passing str as parameter
- Taking s as a temporary string , we will store the string in s till we get aur delimiter (space in this case)
- When the delimiter is encountered the string s is printed and reinitialized to empty string
- This process is repeated till the eng of the string
Output
The split string is:
Opengenus
Article
to
Split
the
String
3. Using strtok() function
strtok() is a function which gives the pointer to the first token in the string in the first call , pointer the second token in the in second call ,till there is no more token left in the string.
After returning the pointer to the last token in the string , it returns NULL pointer.
how to use
char *ptr = strtok( str, delim)
where str is the string and deleim is delimiter or token which we want to search in the string. It can be anything e.g. comma(,) , space( ), hyphen(-) etc.
C++ Program
#include <iostream>
#include <cstring>
using namespace std;
int main()
{
char str[100]; // declare the size of string
cout << " Enter a string: " <<endl;
cin.getline(str, 100); // use getline() function to read a string from input stream
char *ptr; // declare a ptr pointer
ptr = strtok(str, " , "); // use strtok() function to separate string using comma (,) delimiter.
cout << " Split string using strtok() function: " << endl;
while (ptr != NULL)
{
cout << ptr << endl; // print the string token
ptr = strtok (NULL, " , ");
}
return 0;
}
Output
Enter a string:
This is one of the way to split a string in C++
Split string using strtok() function:
This
is
one
of
the
way
to
split
a
string
in
C++
With this article at OpenGenus, you must have the complete idea of how to split a string in C++.