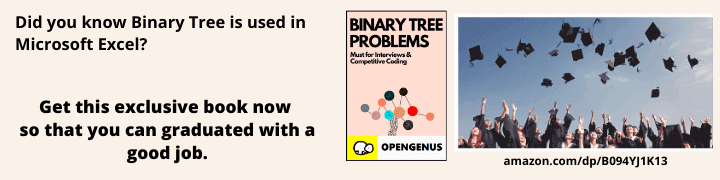
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
During the execution of the program, the developer needs to clear the screen or remove the previous output for new output. To clear the console screen in the c language many methods are available.
Here are some of the following :
- Clrscr() function
- System(“clear”) function
- System(“cls”) function
Important Note :
This function depends on the type of operating system, compiler, and other factors.
For example: If you try to compile the program using the clrscr() function in a modern compiler then it will generate an error. These errors are "Function is not declared " or "conio. h file not found " etc.
So it is recommended that use this function in their specified compiler.
1 .clrscr () function:
Clrscr() is a library function in the c language. It is used to clear the console screen. It moves the cursor to the upper left hand of the console. Clrscr() function is used with conio.h header file.
It clears the console screen whenever a function invokes. To use this function Users can call clrscr() in the main function or any function in which it is defined.
Note :
Clrscr() is not a standard library function.it is predefined function in conio.h [console input output header file ] header file . So it is only used to clear consoles in old compilers like Turbo C or C++.
Advantage:
- Make execution fast.
- Useful in MS-DOS console screen in the older compiler.
Disadvantage:
- Not useful for modern compilers.
- Only available in the window system.
- This function is optional.
- Only work for turbo c compiler.
- Every time user must include a conio.h file.
Syntax:
Void clrscr (void);
Or
Clrscr();
Parameter :
Void: it is a function that has no return data type.
clrscr() : function to clear screen
Return Type :
Don’t have any return type .as it uses the void function.
Example:
//Program to clear screen in Modern complier like Gcc
//Run in vscode
#include<stdio.h>
//include conio.h header file
#include<conio.h>
int main()
{
int a,b,sum;
clrscr(); //clear screen
printf("Enter No 1\n"); //This statement will input no 1
scanf("%d",&a);
printf("Enter No 2\n"); //This statement will input no 2
scanf("%d",&b);
sum=a+b;
printf("Sum Of Two Number=%d",sum); //This statement will sum of number
}
Output
Enter no1 : 20
Enter no2 : 20
Sum = 40
Explanation :
In this program, we calculate and print the sum of two numbers. after the declaration of two numbers, we have to call the clrscr() function. I will clear previous output screen .When we execute this program for first time it will print the sum of number and when we run this program for second time it will clear the previous output and only display current output .
If we don’t use clrscr() then it prints new output along with old output.like this
Enter no1 : 20
Enter no2 : 20
Sum = 40Enter no1 : 10
Enter no2 : 10
Sum = 20
Note :
This will not work on Dec C++ Complier . Use cleardevice() function.
2.system("clear")
The second method to clear console screen is clear() in linux.
As the name suggests it is used to clear the console screen. In which system() is a library function that is available in stdlib.h header file.
Syntax:
System.("clear")
parameter
System: the system used to run command prompt commands
Cls: clear the output screen or console screen.
Advantage :
- Useful for the Linux and macOS operating systems.
- Useful for modern compilers like GCC/gcc++ in Linux.
Disadvantage
- limited to specific operating system or complier.
- Only useful for Linux.
Example :
//Program to clear screen in Linux
#include<stdio.h>
//include stdlib header file
#include<stdlib.h>
int main()
{
printf("Hello"); //This statement will print Hello
getchar();
system("clear"); // This statement will clear previous output.
printf("World "); //This statement will print World
}
Output:
Hello
After Clear The Ouput
World
Explanation :
In the given program we have used the system("clear") function to clear the screen . in the first step it prints “ Hello “ and the getch will wait to accept a character and doesn’t echo it on the screen.
Then system(“clear”) will clear the previous output and print the next statement World on the console.
3.system.cls()
Cls() function is used to clear the console screen like clrscr(). Where system() is a library function available inside stdlib.h [ standard library library] header file.
Syntax:
System.cls()
parameter:
System: used to run command prompt commands and also wait for a user to enter or press the key to terminate the program.
Cls: clear the output screen.
Advantage :
- Useful for Modern Complier like GCC.
- Useful for the window.
- Useful for Turbo C compiler
Disadvantage :
- This is only used for window system.
Example :
//Program to clear screen in Modern complier like Gcc
//Run in vscode
#include<stdio.h>
//include stdlib header file
#include<stdlib.h>
int main()
{
printf("Hello"); //This statement will print Hello
getchar();
system("cls"); // This statement will clear previous output.
printf("World "); //This statement will print World
}
Output :
Hello_
After clear the output
world
Explanation :
In the given program we have used the system(“cls”) function to clear the screen. in the first step, it prints “ hello “ and the getch will wait to accept a character and doesn’t echo it on the screen.
Then system(“cls”) will clear the previous output and print the next statement World on the console.
Application :
To clear console screen.
To display output in manner .
Check Your Understanding
Question
Which Of the follwing is not used to clear console screen ?
Question
To clear console screen in old complier like turbo c which function is used ?
With this article at OpenGenus, you must have the complete idea of how to clear console in C Programming Language.