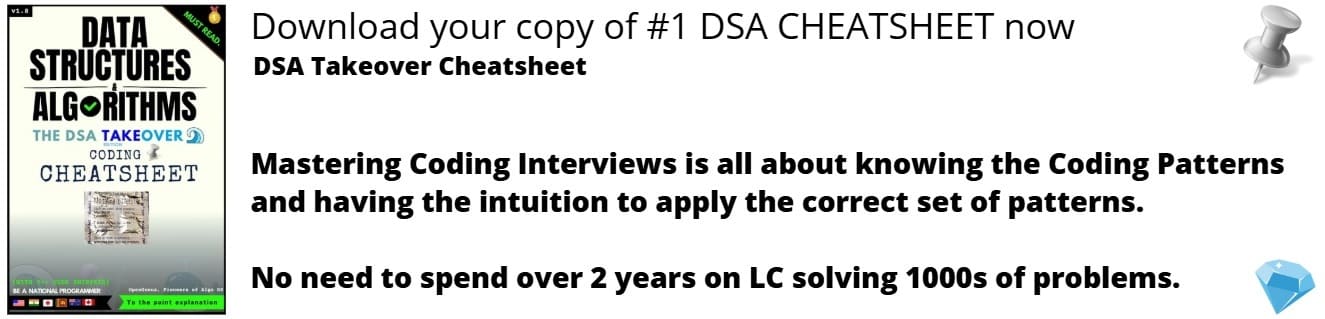
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
We all must the idea of the Constructor
in Java. But the difference is, the Static Initialization Block is a set of instructions that are run only once when the class is loaded into memory. On the other hand, constructors are run each time a new instance of a class is created. We will explore this later.
Static Initialization Block
Topics that we will cover here:
- What is Static Initialization Block In Java?
- How can we create a static initialization block?
- Properties or Features of Static Initialization Block.
- Uses
What is Static Initialization Block in Java?
In Java, the static keyword is used for the management of memory mainly. the static keyword can be used with Variables, Methods, Block and nested class.
Now let's look at a code before defining a static block.
public class Main{
public static void main(String[] args){
System.out.println("Hello World");
}
}
Output
Hello World
We are all familiar with it. Now the question is that,
Can we print Hello World without the presence of the main Method?
The answer is Yes.
Now let us see the definition of Static Initialization Block.
A static block in a program is a set of statements which are executed by the JVM (Java Virtual Machine) before the main method.Java does not care if this block is written after the main( ) method or before the main( ) method, it will be executed before the main method( ) regardless.
public class Sample
{
static{
System.out.println("Hello World");
System.exit(0);
}
}
Note:
This code is working only on java versions up to 1.6. A newer version of java
does not support this feature anymore. We have to include the main method
in our class with the static block.
How can we create a static initialization block?
public class Main
{
static double percentage;
static int rank;
// Static Initialization Block
static
{
percentage = 44.6;
rank = 12;
System.out.println("STATIC BLOCK");
}
public static void main(String args[])
{
Main st = new Main();
System.out.println("MAIN METHOD");
System.out.println("RANK: " + rank);
}
}
Output
STATIC BLOCK
MAIN METHOD
RANK: 12
Properties:
- Static blocks execute automatically when the class is loaded in the memory.
- We can make multiple static initialization blocks in one class.The static blocks were executed serially in the order in which they were declared and the values assigned by the first two static blocks is replaced by the final (third static block).
public class Main{
static{
System.out.println("I am in first static block.");
}
public static void main(String[] args){
System.out.println("Hello World.");
}
static{
System.out.println("I am in second static block.");
}
}
Output:
I am in first static block.
I am in second static block.
Hello World.
- In the entire program, the Static Initialization Block will execute only one time.
- This block will not return anything.
- Checked exceptions cannot be thrown.
Uses:
- Static initialization block is executed at class loading, hence at the time of class loading if we want to perform any activity, we have to define that inside static block.
- Static block is used to load native methods.
- Static block is used to initialize the static members.
Real World Implementation
Problem Statement:
You are given a class Solution with a main method. Complete the given code so that it outputs the area of a parallelogram with breadth and height. You should read the variables from the standard input.
If H<=0 or B<=0 , the output should be "java.lang.Exception: Breadth and height must be positive" without quotes.
Input:
the breadth and height of the parallelogram.
Output:
Area, if both B and H greater than 0.
print exception, otherwise.
Constraints:
-100<= B,H <= 100
Explanation:
Simple question,Here we will take input, check them, and print exceptions if required.But the interesting thing is that we will take input or print the exception with the help of static blocks. Let's start it.
import java.io.*;
import java.util.*;
import java.text.*;
import java.math.*;
import java.util.regex.*;
// Step 1: Create the Solution class
public class Solution {
// Step 2: Create the required instance variable(must be static)
static int B,H;
static boolean flag = true;
/* Step 3: Initialize those variable (take user input) with the help of static block*/
static{
Scanner sc= new Scanner(System.in);
B= sc.nextInt();
H= sc.nextInt();
try{
if(B<=0 || H<=0){
/* Step 4: make the flag false and throw the exception with the specific message */
flag= false;
throw new Exception("Breadth and height must be positive");
}
}
catch(Exception e){
// Step 5: catch the exception and print it.
System.out.println(e);
}
}
public static void main(String[] args){
// Step 6: give the logic for finding the area
if(flag){
int area=B*H;
System.out.print(area);
}
}//end of main
}//end of class
Try it in your editor.
With this article at OpenGenus, you must have the complete idea of Static Initialization Block in Java.