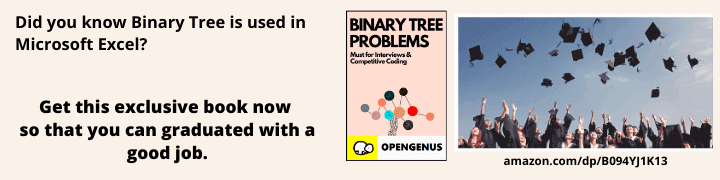
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
Reading time: 30 minutes | Coding time: 5 minutes
Type converison is the process where one data type is converted to another with or without loss in information. Note that in some conversions like double to int, information is lost while in other conversions like character to int, no information is lost.
There are 3 methods which can perform this conversion:
- Implicit type casting
- Explicit type casting
- Using cast operation (within explicit type + 4 sub operators)
How many parameters does a conversion operator may take?
Impicit Type Casting
Implicit Type Casting is the type conversion that is performed by the compiler automatically to ensure calculations between same data type take place. Some important points are:
-
Done by the compiler on its own without any input from the user.
-
Generally takes place when in an expression more than one data type is present. In such condition type casting (type conversion) takes place to avoid lose of data.
-
All the data types of the variables are upgraded to the data type of the variable with largest data type.
NOTE : It is possible for implicit conversions to lose information, signs can be lost (when signed is implicitly converted to unsigned), and overflow can occur (when long long is implicitly converted to float).
Example to demonstrate implicit type conversion:
// An example of implicit conversion
#include <iostream>
using namespace std;
int main()
{
int x = 20; // integer x
char y = 'a'; // character c
// y implicitly converted to int. ASCII
// value of 'a' is 97
x = x + y;
// x is implicitly converted to float
float z = x + 1.0;
cout << "x = " << x << endl
<< "y = " << y << endl
<< "z = " << z << endl;
return 0;
}
Output:
x = 117
y = a
z = 118
Explicit Type Casting
This process is also called explicit type casting and it is user-defined. Here the user can convert the result to make it of a particular data type.
In C++, it can be done by two ways:
Converting by assignment : This is done by explicitly defining the required type in front of the expression in parenthesis. This can be also considered as forceful casting.
Syntax:
type (expression)
where type indicates the data type to which the result is converted.
Example to demonstrate explicit type conversion
// C++ program to demonstrate
// explicit type casting
#include <iostream>
using namespace std;
int main()
{
double x = 100.6;
// Explicit conversion from double to int
int sum = int(x) + 1;
cout<< sum;
return 0;
}
Output:
101
Conversion using Cast operator
Conversion using Cast operator : A Cast operator is an unary operator which forces one data type to be converted into another data type.
C++ supports four types of casting:
1)Static Cast : This is the simplest type of cast which can be used. It is a compile time cast.It does things like implicit conversions between types (such as int to float, or pointer to void*), and it can also call explicit conversion functions (or implicit ones).
2)Dynamic Cast : Dynamic cast can only be used with pointers and references to classes (or with void*). Its purpose is to ensure that the result after the conversion points to a valid complete object.
3)Const Cast : Const cast is used to cast away the constness of variables.
4)Reinterpret Cast : It is used to convert one pointer of another pointer of any type, no matter either the class is related to each other or not.
Example of using static_cast
//Static Cast
#include <iostream>
using namespace std;
int main()
{
float f = 5.5;
// using cast operator
int b = static_cast<int>(f);
cout << b;
}
Output:
5
Example of using dynamic_cast
// Dynamic Cast
#include<iostream>
using namespace std;
class B
{ virtual void fun()
{}
};
class D: public B
{
};
int main()
{
B *b = new D;
D *d = dynamic_cast<D*>(b);
if(d != NULL)
cout << "works";
else
cout << "cannot cast B* to D*";
getchar();
return 0;
}
Output:
works
Example of using const_cast
//Const cast
#include <iostream>
using namespace std;
class student
{
private:
int roll;
public:
// constructor
student(int r):roll(r)
{
}
// A const function that changes roll with the help of const_cast
void fun() const
{
( const_cast <student*> (this) )->roll = 10;
}
int getRoll()
{
return roll;
}
};
int main(void)
{
student s(1);
cout << "Old roll number: " << s.getRoll() << endl;
s.fun();
cout << "New roll number: " << s.getRoll() << endl;
return 0;
}
Output:
Old roll number: 1
New roll number: 10
Example of using reinterpret_cast
//Reinterpret cast
#include <iostream>
using namespace std;
int main()
{
int* p = new int(65);
char* ch = reinterpret_cast<char*>(p);
cout << *p << endl;
cout << *ch << endl;
cout << p << endl;
cout << ch << endl;
return 0;
}
Output:
65
A
0x890a90
A
Advantage of Type Casting
1)This is done to take advantage of certain features of type hierarchies or type representations.
2)It helps to compute expressions containing variables of different data types.