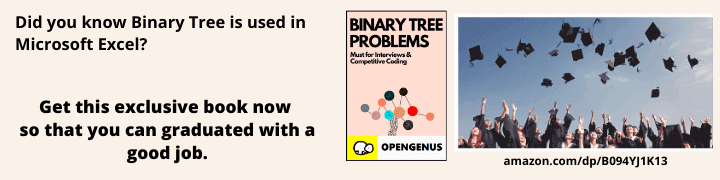
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
In this article, we have explained the reason behind the Python runtime error "TypeError: unsupported operand type(s) for +: 'NoneType' and 'NoneType'" and presented two ways to fix the error.
Table of contents:
- Understanding the error
- Fix with print()
- Fix 2: Function in Python
- Concluding Note
Understanding the error
If you run the following Python code:
list = [59, 47, 7, 17, 45]
f = lambda l: print(l[0]) if len(l) == 1 else print(l[0]) + f(l[1:])
sum = f(list)
You will get the following runtime error:
Traceback (most recent call last):
File "code.py", line 3, in <module>
sum = f(list)
File "code.py", line 2, in <lambda>
f = lambda l: print(l[0]) if len(l) == 1 else print(l[0]) + f(l[1:])
File "code.py", line 2, in <lambda>
f = lambda l: print(l[0]) if len(l) == 1 else print(l[0]) + f(l[1:])
File "code.py", line 2, in <lambda>
f = lambda l: print(l[0]) if len(l) == 1 else print(l[0]) + f(l[1:])
[Previous line repeated 1 more time]
TypeError: unsupported operand type(s) for +: 'NoneType' and 'NoneType'
The main issue is that you cannot add an element of type NoneType with another other element. In this case, both elements are of type NoneType. The other variants of this error will be:
TypeError: unsupported operand type(s) for +: 'NoneType' and 'Float'
TypeError: unsupported operand type(s) for +: 'NoneType' and 'Int'
Fix with print()
The fix is to remove or update the part where addition taken place to ensure that no element is of type NoneType.
For the original code, we presented that is:
list = [59, 47, 7, 17, 45]
f = lambda l: print(l[0]) if len(l) == 1 else print(l[0]) + f(l[1:])
sum = f(list)
The problem is the return type of print() built-in function in Python is NoneType and the lambda we are using is not returning anything so return type is NoneType. This is why addition happens between NoneType elements at the end.
Hence, for this case, the fix is to change the complete approach as if we use print and addition in the lambda, there is no way to fix the issue.
Fix 2: Function in Python
Another common source of the issue is that functions do not return value in each case and hence, addition takes place between NoneType.
Consider the following Python code where we have a function that doubles a number if it is positive:
def positive(number):
if(number > 0):
return number*2
print(positive(4) + positive(-1))
This gives the following error:
Traceback (most recent call last):
File "code.py", line 5, in <module>
print(positive(4) + positive(-1))
TypeError: unsupported operand type(s) for +: 'int' and 'NoneType'
The issue is the function has not handled the case of negative numbers and in this case, it does not return anything that is the return type is NoneType. This causes a runtime error if addition takes place with the function's return type.
The fix will be to handle negative numbers in the function that is return negative numbers as it is. The fixed Python code is as follows:
def positive(number):
if(number > 0):
return number*2
return number
print(positive(4) + positive(-1))
Output:
7
The output is correct as in the positive() user-defined function, we have added a new line "return number". This handles the case of negative numbers. If number is positive, we return the double of the number and if the number is negative, we return the negative number as it is.
Concluding Note
With this article at OpenGenus, you must have the complete idea of how to solve this error "TypeError: unsupported operand type(s) for +: 'NoneType' and 'NoneType'" in Python.
Always ensure that the elements you are adding is of correct data type. NoneType cannot be added with anything.