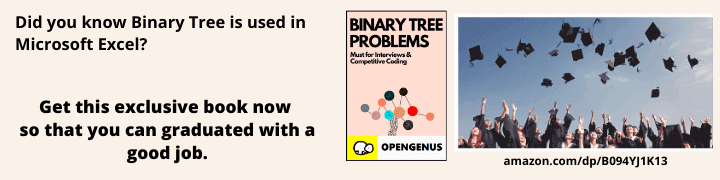
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
In this article, we will explore different ways to delete an element in a List using Python's built-in methods and functions and apply it to delete the first and last element of a List.
Table of Content:
- Introduction to List
- Removing element using built-in function remove()
- Removing elements by index or slice using del keyword
- Removing an element by index using list pop()
- Removing all elements using List clear()
- Remove first element of the list
- Remove last element of the list
Introduction of List
Lists are one of the most commonly used data structures in Python. They allow us to store and manipulate a sequence of values of any type. Sometimes, we may need to remove an element from a list based on its value or its index. In this blog post, we will explore different ways to do that using Python's built-in methods and functions.
Removing element using built-in function remove()
The remove()
method is one of the ways you can remove elements from a list in Python. The remove()
method removes an item from a list by its value and not by its index number.
The syntax of the remove() method is:
list_name.remove(value)
where list_name
is the name of the list you're working with and value
is the item you want to remove.
For example, suppose we have a list of fruits:
fruits = ["apple", "banana", "cherry", "orange"]
If we want to remove "cherry" from the list, we can use:
fruits.remove("cherry")
This will modify the original list and make it:
fruits = ["apple", "banana", "orange"]
Some important points to note about the remove()
method are:
- It only removes the first occurrence of the value in the list. If there are multiple items with the same value, it will not remove them all.
- It does not return any value. It modifies the original list in-place.
- It raises a ValueError exception if the value is not found in the list.
The time complexity of the remove()
method is O(n), where n is the length of the list. This is because it has to scan through all the elements until it finds a match.
The remove()
method is useful when you know the value of the element you want to delete and you don't care about its position.
Removing elements by index or slice using del keyword
Another way to delete an element from a list using Python is by using del
statement. The del
statement can be used to delete an item from a list by specifying its index.
The syntax of del
statement is:
del list_name[index]
where list_name
is again name of your List object and index
represents position at which you want to delete an item.
For example, if we want to delete "banana" from our fruits list, we can use:
fruits = ["apple", "banana", "cherry", "orange"]
del fruits[1]
This will also modify our original fruits List object as follows:
fruits = ["apple", "cherry", "orange"]
Some important points about del statement are:
- It can also be used to delete multiple items at once by using slicing notation. For example,
del fruits[1:3]
will delete items at index 1 and 2 (but not 3). - It does not return any value either. It modifies original List object directly.
- It raises an IndexError exception if index out-of-range for given List object.
The time complexity of del statement depends on how many items are deleted and how many items are shifted after deletion. In general, it can be O(n) where n again represents length of List object.
The del
statement is useful when you know position (index) at which you want to delete an item or multiple items at once.
Removing an element by index using list pop()
Another built-in method that can be used for removing elements from lists in Python is pop()
. The pop()
method removes an element from a list based on its index and returns it.
The syntax for pop()
method is:
list_name.pop(index)
where list_name
again represents name for your List object while index
represents position at which you want to pop (remove) an item.
For example, if we want to pop (remove) last item ("orange") from our fruits List object then we can use following code snippet:
fruits = ["apple", "cherry", "orange"]
last_item = fruits.pop()
print(last_item)
# Output: orange
print(fruits)
# Output: ["apple", "cherry"]
As you can see above code snippet prints popped (removed) item as well as modified version for our original fruits List object after popping last item ("orange").
Some important points about pop()
method are:
- If no index argument passed then it pops last item by default.
- If index argument passed then it pops item at given index position.
- It returns popped (removed) item as output unlike other two methods discussed above which do not return
Removing all elements using List clear()
The clear()
method is a built-in function that removes all the elements from a list. It does not return any value; it just modifies the original list object.
The syntax of using clear()
is:
list_name.clear()
where list_name is the name of the list that you want to clear.
For example:
numbers = [1, 2, 3, 4]
numbers.clear()
print(numbers) # prints []
The clear() method is available in Python 3.x versions. If you are using Python 2.x versions, you can use del instead.
Remove first element of the list
- Remove first element of the list using remove()
list_name.remove(list_name[0])
In this, we pass the first element of the list to be removed. As remove() removes the first occurance of an element, the first element gets removed.
- Remove first element of the list using del
del list_name[0]
The second approach is to use the del function and delete the element at index 0 that is the first element.
- Remove first element of the list using pop()
list_name.pop(0)
Similar to remove(), on using pop() on index 0, the first element is deleted.
Remove last element of the list
- Remove last element of the list using del
del list_name[len(list_name)-1]
This approach is to use the del function and delete the element at index len(list_name)-1 (that is the last index) that is the last element.
- Remove last element of the list using pop
list_name.pop()
By default, if no parameter is passed to pop(), the last element is removed.
With this article at OpenGenus, you must have the complete idea of removing elements from a List in Python.