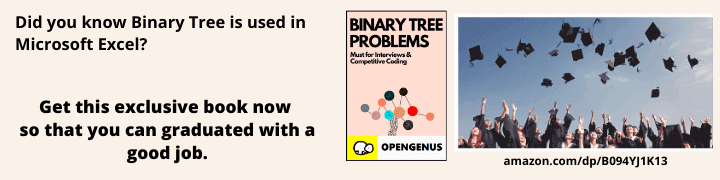
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
In this article, we have presented two different ways to do Dot Product of Two Vectors in C++. This involves the use of inner_product method in C++ STL (Standard Template Library).
Table of contents:
- Introduction to vector and dot product
- Dot product in C++: Iterative method
- Dot product in C++: inner_product in STL
Prerequisite: Vector in C++ STL
Let us get started with Dot Product of Two Vectors in C++.
Introduction to vector and dot product
A vector quantity is a physical quantity that has both magnitude and direction whereas a scalar quantity is a physical quantity that has only magnitude and no direction.
The geometric definition of dot product defines dot product between two vectors a and b as
a.b = |a||b|cosθ
where θ is the angle between vectors a and b.
In vector algebra dot product is the sum of the products of the corresponding elements of the two vectors.
The dot product between a pair of distinct standard unit vectors (Unit vectors are vectors with magnitude 1) is zero:
iâ‹…j = iâ‹…k = jâ‹…k = 0.
i, j and k are unit vectors along x, y and z directions respectively.
The dot product between a unit vector and itself is 1.
iâ‹…i = jâ‹…j = kâ‹…k = 1
E.g. We are given two vectors V1 = a1*i + b1*j + c1*k
and V2 = a2*i + b2*j + c2*k
where i, j and k are the unit vectors along the x, y and z directions. Then the dot product is calculated as
V1.V2 = a1*a2 + b1*b2 + c1*c2
The result of a dot product is a scalar quantity.
Dot product in C++: Iterative method
- Using iterative approach
#include <bits/stdc++.h>
using namespace std;
int dot_product(vector<int> &v1, vector<int> &v2){
int product = 0;
for(int i=0;i<v1.size();i++)
product += v1[i] * v2[i];
return product;
}
int main() {
vector<int> V1 ;
vector<int> V2;
int length1,length2,input,product = 0;
cout<<"Enter size of first vector:";
cin >> length1;
cout<<"Enter size of second vector:";
cin >> length2;
cout << "Enter elements of the first vector:";
for(int i=0;i<length1;i++){
cin>>input;
V1.push_back(input);
}
cout << "Enter elements of the second vector:";
for(int i=0;i<length2;i++){
cin>>input;
V2.push_back(input);
}
cout << "Dot product : " << dot_product(V1,V2);
return 0;
}
Output:
Enter size of first vector:5
Enter size of second vector:5
Enter elements of the first vector:1 2 3 4 5
Enter elements of the second vector:2 4 6 8 10
Dot product : 110
Explanation :
Here we are iterating through the vectors and finding the sum of the products of the corresponding elements i.e. 1*2 + 2*4 + 3*6 + 4*8 + 5*10 = 110
Dot product in C++: inner_product in STL
- Using std::inner_product
#include<iostream>
#include<numeric>
#include<vector>
using namespace std;
int main(){
vector<int> v1 { 1, 2, 3, 4, 5,};
vector<int> v2 { 2, 4, 6, 8, 10};
cout << "Dot Product : " << inner_product(v1.begin(), v1.end(), v2.begin(), 0) << endl;
}
Output :
Dot Product : 110
Explanation :
std::inner_product is included in the <numeric>
header file. It calculates the sum of the products on two ranges. The first range is given by the begin/end
iterators indicating the initial and final positions in the first sequence. The second range is only given by the initial position. It takes the same number of elements as the first range. The method takes another parameter, init
, which is the initial value of the sum of the products. Here our init value is 0
. The return value is the result of accumulating init and the product of all the pairs of elements in the given ranges.
With this article at OpenGenus, you must have a strong idea of how to do dot product of two vectors in C++ natively and using functions in C++ STL.