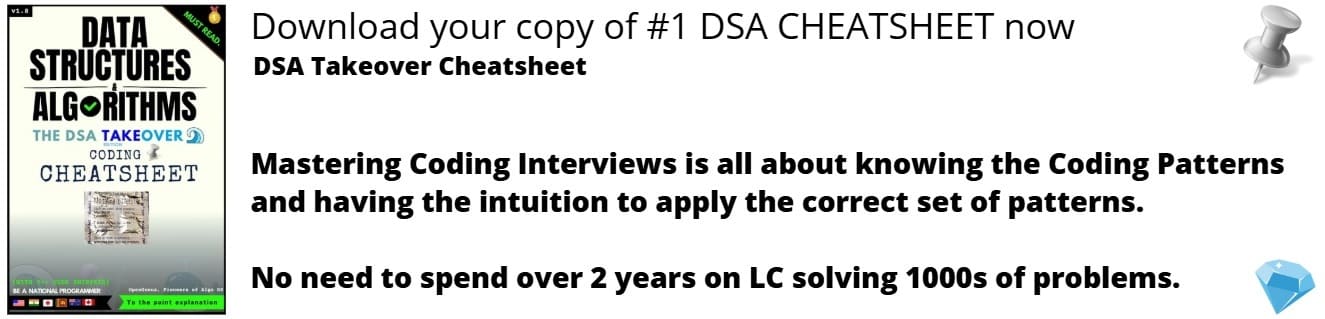
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
Table of Contents:
- Introduction
- Understanding Error Handling
a. Use Exception Handling
b. Create Custom Error Classes
c. Provide Meaningful Error Messages
d. Log Errors - The Importance of Logging
a. Use Different Logging Levels
b. Log Contextual Information
c. Centralized Log Management
d. Monitor Logs Regularly - Handling Unforeseen Errors
a. Implement Circuit Breakers
b. Graceful Degradation - Error and Logging Handling in Node.js Backend Applications
5.1 Setting Up the Node.js Backend Application
5.2 Error Handling in Node.js Backend Application
5.2.1 Example of Error Log
5.2.2 Solution
5.3 Logging in Node.js Backend Application
5.3.1 Example of Request Logs - Conclusion
Introduction
In the realm of software development, creating robust and reliable applications is of paramount importance. As backend applications play a critical role in processing data and handling business logic, effective error handling and logging are essential components of a well-structured and maintainable system. This article at OpenGenus delves into the significance of error handling and logging in backend applications, outlining best practices to ensure smooth operations, quicker debugging, and enhanced user experience.
Understanding Error Handling
Error handling is the process of identifying, capturing, and managing errors that occur during the execution of a backend application. These errors can stem from various sources, such as invalid input, database issues, external service failures, or programming errors. Properly managing these errors can prevent application crashes, unexpected behavior, and data corruption.
Best Practices for Error Handling
a. Use Exception Handling: Utilize programming language-specific exception handling mechanisms to capture and manage errors effectively. These exceptions allow you to handle errors gracefully, providing an opportunity to recover or fallback to alternative strategies.
b. Create Custom Error Classes: Implement custom error classes to categorize and differentiate various types of errors. This practice enhances readability and helps developers pinpoint the root causes of issues more efficiently.
c. Provide Meaningful Error Messages: Ensure that error messages are clear, concise, and user-friendly. Avoid exposing sensitive information in error messages, but include enough context for developers to diagnose and resolve the problems quickly.
d. Log Errors: Alongside handling errors gracefully, it is essential to log errors appropriately. This leads us to the next topic - the significance of logging in backend applications.
The Importance of Logging
Logging is the practice of recording important events, activities, and errors that occur within the backend application during its runtime. Effective logging is invaluable for developers and system administrators as it offers insights into application behavior and helps troubleshoot issues efficiently.
Best Practices for Logging
a. Use Different Logging Levels: Employ multiple logging levels (e.g., INFO, DEBUG, WARN, ERROR) to distinguish between informational messages and critical errors. This hierarchy aids developers in identifying the severity of issues and their impact on the application's functionality.
b. Log Contextual Information: Include relevant contextual data in log entries, such as timestamps, request IDs, user IDs, and any other data that might help correlate logs and trace the flow of a request through the application.
c. Centralized Log Management: Implement a centralized log management system to aggregate logs from various application instances and services. This approach simplifies log analysis, monitoring, and troubleshooting, especially in distributed systems.
d. Monitor Logs Regularly: Regularly monitor and analyze logs to detect potential issues before they escalate. Use tools that provide real-time log monitoring and alerting to address critical problems promptly.
Handling Unforeseen Errors
Despite careful planning, backend applications might encounter unforeseen errors in real-world scenarios. To better manage such situations:
a. Implement Circuit Breakers: Circuit breakers are a design pattern that prevents an application from repeatedly calling a failing service or component. This mechanism helps improve application resiliency and prevents cascading failures.
b. Graceful Degradation: Design your application to gracefully degrade functionality in case of non-critical errors. For instance, if an external service is unavailable, the application can offer a limited feature set instead of crashing entirely.
In this article we are going to dive into Nodejs in Error and Logging Handling in Backend Applications.
Apologies for the oversight. Let's include examples of error logs and their corresponding solutions in the Node.js backend application.
Error and Logging Handling in Node.js Backend Applications
Node.js has become a popular choice for building backend applications due to its non-blocking, event-driven architecture. However, handling errors and logging effectively are crucial aspects to ensure the stability and reliability of these applications. In this section, we will explore how to implement error handling and logging in a Node.js backend application using Express.js as the framework.
5.1 Setting Up the Node.js Backend Application
First, let's create a basic Node.js backend application using Express.js. Make sure you have Node.js and npm (Node Package Manager) installed on your system.
Step 1: Initialize the project and install dependencies:
mkdir backend-app
cd backend-app
npm init -y
npm install express morgan
Step 2: Create the main application file (index.js):
const express = require('express');
const morgan = require('morgan');
const app = express();
const port = 3000;
// Middleware: Logging HTTP requests
app.use(morgan('dev'));
// Route: Hello Nodejs
app.get('/', (req, res) => {
res.send('Hello, Nodejs!');
});
// Start the server
app.listen(port, () => {
console.log(`Server is running on http://localhost:${port}`);
});
5.2 Error Handling in Node.js Backend Application
Error handling is crucial to handle unexpected scenarios and prevent application crashes. Let's implement a custom error handler middleware to capture and manage errors gracefully.
Step 1: Add the custom error handler middleware:
function errorHandler(err, req, res, next) {
console.error(err.stack);
res.status(500).json({ error: 'Something went wrong!' });
}
app.use(errorHandler);
Step 2: Test the error handler by creating a route that throws an intentional error:
app.get('/error', (req, res, next) => {
const err = new Error('This is an intentional error for testing purposes');
next(err);
});
Example of Error Log:
Suppose the /error
route is accessed by a client, the custom error handler middleware will capture the error and log it to the console. The error log will look like this:
Error: This is an intentional error for testing purposes
at /path/to/your/project/index.js:14:23
at Layer.handle [as handle_request] (/path/to/your/project/node_modules/express/lib/router/layer.js:95:5)
at next (/path/to/your/project/node_modules/express/lib/router/route.js:137:13)
at Route.dispatch (/path/to/your/project/node_modules/express/lib/router/route.js:112:3)
at Layer.handle [as handle_request] (/path/to/your/project/node_modules/express/lib/router/layer.js:95:5)
at /path/to/your/project/node_modules/express/lib/router/index.js:281:22
at Function.process_params (/path/to/your/project/node_modules/express/lib/router/index.js:335:12)
at next (/path/to/your/project/node_modules/express/lib/router/index.js:275:10)
at Function.handle (/path/to/your/project/node_modules/express/lib/router/index.js:174:3)
at router (/path/to/your/project/node_modules/express/lib/router/index.js:47:12)
Solution:
The error log provides valuable information, including the error message and the stack trace showing the sequence of function calls that led to the error. In this case, the error occurred in the index.js
file at line 14, which was triggered intentionally for testing purposes.
The custom error handler middleware sends an HTTP response with a 500 status code and a JSON object containing the error message ({ error: 'Something went wrong!' }
). This user-friendly response ensures that the client receives an appropriate error message while keeping sensitive information hidden.
5.3 Logging in Node.js Backend Application:
Logging is essential for monitoring the application's behavior and diagnosing issues. We'll integrate logging into our backend application using the "morgan" middleware, which logs HTTP requests.
Step 1: Configure the logging middleware (already done in the initial setup):
const morgan = require('morgan');
app.use(morgan('dev'));
Example of Request Logs:
When the application receives HTTP requests, the "morgan" middleware logs the requests to the console. For example, accessing the root route /
and the /api/data
route might produce the following logs:
GET / 200 25.965 ms - 14
POST /api/data 404 8.682 ms - 23
The first log entry shows that a GET request was made to the root endpoint ('/'), and it returned a 200 status code with a response time of 25.965 ms.
The second log entry shows that a POST request was made to the '/api/data' endpoint, but the endpoint was not found (404 error), and the response time was 8.682 ms.
Conclusion
In this article at OpenGenus, we've explored how to implement error handling and logging in a Node.js backend application using Express.js. By setting up a custom error handler middleware, we can gracefully manage errors and provide meaningful responses to users.
Integrating logging using the "morgan" package allows us to monitor and diagnose application behavior effectively. Additionally, we examined error logs and how the custom error handler middleware responds to errors. We also explored request logs generated by the "morgan" middleware, which provide valuable insights into the application's runtime behavior.