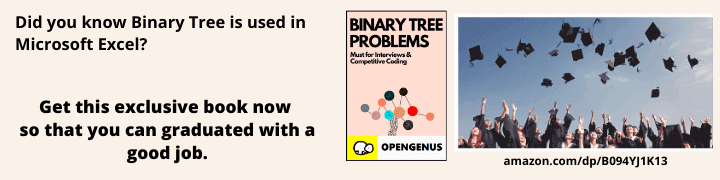
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
Transferring data from one programming language to another would have been almost impossible if there wasn't a way of writing and reading data from files. It is a very common practice to share data from one programming language to another. This is done by performing some operations on the file, saving the file and sharing the file through electronic means. The most widely accepted file format across all programming languages are JSON and CSV files.
Due to the persistence of data stored in files, It is sometimes used as a storage system. Similar to the database storage system, a file storage system, stores usersβ data in a file and the same data are read from the file to render dynamic content. However, data stored in files are not secured and that's why it's seldom used in production but it's acceptable in development.
Handling files in C programming languages requires knowledge and application of some file-handling operations. These operations allow us to be able to interact with files in C programming. With the countless and rapid usage of files in programming, it becomes imperative to learn and understand how to handle files properly. Hence, we will examine the file handling in C.
Table of contents
- What is file handling in C?
- What is a file in C?
- Importance of file handling in C
- Types of files in the C Program
- The most commonly used function in file handling in C
- Operations on file handling in C with examples
What is file handling in C?
File handling in C is the way of storing data in a file and retrieving data from the file. The data stored in a file can be generated from the output of C programs and stored in a file. similarly, the data used by the program can be fetched from the file as input.
What is a file in C?
A file refers to a source in which a program stores the data in the form of bytes of sequence on a disk permanently. The content stored in a file isn't volatile. However, a C program can perform various operations, such as creating, opening, reading a file, or even manipulating the data inside the file. The process is known as file handling in C.
Importance of file handling in C
There are times when compiling and running c programs do not serve our intended purpose. In such cases, we might want to store the program's output for future purposes. For instance, printing out program logs every time the program is run can be tedious. Keeping such an important program's event in a file is one of the importance of file handling in c.
Let us look at a few reasons why file handling is important and make our life easier as a developer.
Reusability: Whenever a C program is compiled, we have to keep running the program to generate the same output. That sometimes can be very stressful. Storing such output in a file and retrieving the result from the file can be very helpful. It allows us to reuse the same output without having to compile and run the program over and over. In other words, it allows us to preserve the data generated after running the program.
Saves Time: Getting huge data from users can be time-consuming and negatively impact the overall run time of the program. Reading the data from the file and selecting the part needed to improve the performance of the program, thus enhancing the easy accessibility of data.
Portability: Content in a file can be easily transferable from one program to another. It saves the loss of data.
Types of files in the C Program
The data stored in a file defines the type of the file. These files are in two (2) distinct forms in the C language, namely:
- Text files
- Binary files
Text Files
Text files are the most basic and simplest type of files that can be created in a C program. The text files are created using the .txt extension with the help of simple text editors such as notepad, vscode, and sublime among others.
Text files have their advantages and disadvantages. The major advantages of text files include being easy to access and use and they are human-readable. The major disadvantage is the lack of security. The content available in text files can be easily compromised and takes up a large space of memory compared to its counterparts. To address the security issue of demerit text files, we have another type of file called Binary files.
Binary Files
The binary files are files whose content is stored in a binary format (0's and 1's) consisting of sequential bytes each with an 8-bit length. The binary files store data in the same way the computer holds data in the memory. Thus, it takes lesser space in storage. Hence, it can be accessed more easily than text files.
The binary files are created using the .bin extension. A binary file is not humanly readable, but it's more secure than text files. Binary files are the most secure file in the C program.
The most commonly used function in file handling in C
Handling files in a C program require the use of some operators or functions in the C programming language to perform some operations on files. These operations include opening files, creating a new file, writing data to a file, close or deleting a file etc.
Functions | Description of Function | |
---|---|---|
fopen() | Opening files either new or existing one | |
fclose() | Closing or flushing the buffer of a program file | |
fscanf() | Reading data stored in a file | |
fprintf() | Writing data into available file | |
fgetc() | Reading a single character from a file at a time | |
fputc() | Writing a single character into available file | |
fgetw() | Reading an integer from a file | |
fputw() | Writing an integer into a file | |
fseek() | Used to move or shift file pointer to a specified position |
Operations in file handling in C with examples
Before examining the different functions in file operations in C, it's important to understand a bit about File Pointers. A file pointer stores the current position of a write or reads within a file. All operations within a file are made with a reference to the file pointer. The data of this pointer is defined in stdio.h header file and named FILE. The file pointer is declared as follows: FILE *fileptr
where fileptr is a file pointer.
File handling in C enables us to be able to perform some operations on the file. As mentioned earlier, the operations in file handling in C include:
- Opening a file that already exists
- Creating a new file
- Reading data from an existing file
- Writing data into the file
- Deleting data in the file or the file itself
Without further ado, let's look at the different file operations in C
Opening a file with fopen() function
File opening is simply the first procedure in interacting with files in the C programming language. It provides access to the file in the file mode in which is opened.
There are 6 major file modes through which you can open a file. These are:
- r - Open text file for reading
- r+ - Open for reading and writing
- w - Truncate file length to zero or open file for reading
- w+ - Open for writing and reading. It creates the file if it's not created
- a - Open for appending to a file. It adds to the end of the file
- a+ - Open for reading and appending (Writing to the end of the file)
There are file descriptors associated with each file mode aforementioned above.
fopen() modes | Open() flags | |
---|---|---|
r | O_RDONLY | |
W | O_WRONLY | O_CREAT | O_TRUNC | |
a | O_WRONLY | O_CREAT | O_APPEND | |
r+ | O_RDWR | |
w+ | O_RDWR | O_CREAT | O_TRUNC | |
a+ | O_RDWR | O_CREAT | O_APPEND |
fopen() function takes two arguments which are the filename and the mode in which the file should be open. This is its prototype: FILE *fopen(const char *restrict pathname, const char *restrict mode)
;
The default mode for opening files is text, but 't' can also be appended to the file mode to specify the text file type. For example, 'wt' can be specified to open a text file for writing. Also, 'b' can be appended to file modes to open binary files. 'rb' indicates opening binary files in read-only mode.
Example of fopen() function
Below are syntax of fopen function
FILE *fp = fopen("example.txt", "r");
FILE *fp = fopen("example2.bin", "wb");
The first line opens the example.txt (text file) in read-only mode. While the latter opens example2.bin (binary file) in write-only mode.
If the fopen() operation is completed successfully, a file pointer will be returned pointing to the beginning of the file otherwise NULL will be returned. It's very important to always test for the return of the fopen() function to prevent unexpected behaviour of our programs. The following example text for the return of the fopen() function.
#include <stdio.h>
int main()
{
FILE *fp = fopen("example.txt", "r");
if (fp == NULL)
{
printf("Unable to open the file")
}
return (0);
}
There are some security issues related to opening a file without closing it after working on the file. As a result, you must close the file after you have worked on it. Some programming languages automatically close a file after it has been worked on but in C programming, it must be explicitly closed.
How do we close a file?
Closing a file in C is relatively easy as frees memory space after finishing working with it. The fclose() function is used to close files. It is used to flush the stream or buffer pointed to by the file pointer. The prototype of fclose is int fclose(FILE *stream)
.
Upon successful completion of the fclose() function, 0 is returned, otherwise, EOF is returned which signifies the end of the file. After closing the file, the subsequent access to the file will result in undefined behaviour.
Example of fclose() function
#include <stdio.h>
int main()
{
FILE *fp = fopen("example.txt", "r");
res = fclose(fp)
return (0);
}
Reading and writing to file with fscanf() and fprintf() function
How do we write to a file?
We write to files by reading a sequence of characters from the output stream using fprintf() function and store it into a file. The prototype of the fprintf() function is - int fprintf(FILE *restrict stream, const char *restrict format, ...)
. It takes three (3) arguments, the first one is the file pointer, followed by the data format %d for integer and %s for string and pointer to the buffer. Alternatively, you can use the fputs() function to write only one character from the output stream at a time. In write mode, if the file has not yet been created, it will auto-create it.
Example of fprintf() function
#include <stdio.h>
int main()
{
FILE *fp = fopen("file.txt", "w");
char buff[255];
scanf("%s", buff);
fprintf(fp, "%s", buff);
res = fclose(fp)
return (0);
}
The file.txt is opened and the buff is defined for creating a char array to store the data on file. The scanf() was used to read data from standard input (stdin) and store it in the buff variable. After that, we use fprintf() to write the data to the buffer in the file. After finishing work with the file, it is closed and the main function is returned. If there was an error while writing data from the input stream, EOF is returned.
How do we read from a file?
The fscanf() function is used to read from the input stream and save it into the file. We can also use the fgetc() function to read a single character from the input stream at a time.
Example of fscanf() function
#include <stdio.h>
int main()
{
FILE *fp = fopen("file.txt", "r");
char buff[255];
while(fscanf(fp, "%s", buff)!=EOF){
printf("%s ", buff );
}
res = fclose(fp)
return (0);
}
The.txt file is opened and the buff is defined to create an array of characters to store the data in the file. The While loop was used to read the sequence of characters from the input stream and store the data in the file. The printf() statement in the while loop was used to output each sequence of characters saved in the file. After completing the work with the file, it is closed and the main function is returned. If there was an error while writing data from the input stream, EOF is returned and the while loop will terminate.
How do we delete files?
We delete files by making a call to remove functions defined in stdio.h header file which deletes files from the filesystem. It calls unlink for files and rmdir for the directory. The remove function takes a single argument which is the file path. Upon the successful deletion of the file, it returns zero (0) otherwise -1 is returned. If any processes are using the file or the file is opened somewhere, the file will not be deleted until the processes are killed or closed. Below is the prototype of the remove function.
int remove(const char *pathname);
Example of remove() function
#include <stdio.h>
int main()
{
int res = remove("example.txt");
if (res == 0)
printf("The File is successfully deleted\n")
else
printf("The file is not deleted\n")
return (0);
}
Generally, File handling in C makes working with files easy for C developers. This makes it possible for them to create a file, write to a file, read a file and delete a file. It saves time preserving their program's output and storing it in a file for easy retrieving of the data. They can as well use a file storage system to make their program persistent.