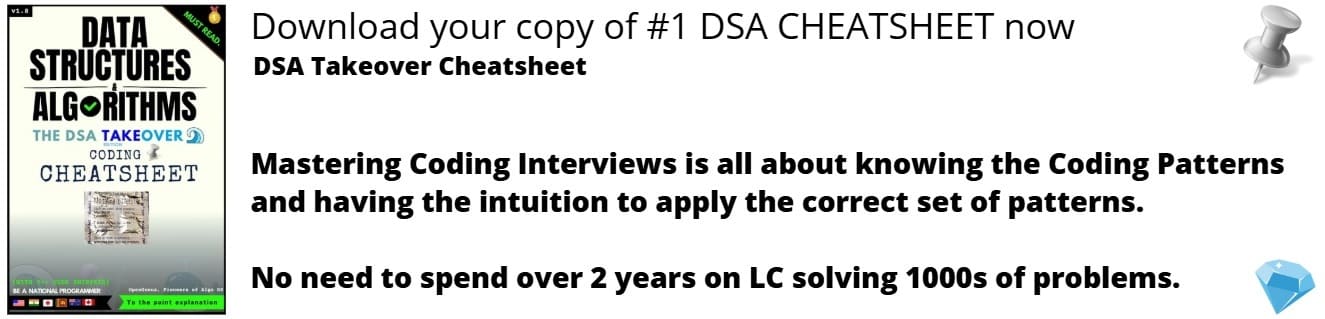
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
Inline function is an important feature of C++ which we have explored in depth in this article.
Firstly, we will understand why the inline function is used.
Inline in C++
When a program calls a function, the CPU saves the memory address of the call in a predefined area and returns control to the calling function. After the CPU executes the function, it records the function return from in the same location. If a function's execution time is shorter than the switching time from the calling function, it becomes overhead; for large functions, this is actually unimportant, but for small functions, the time spent calling the function actually consumes more time than it does performing its function. Inline functions were therefore developed in order to lessen this overhead problem.
Inline function is expanded when the function is called. Whole code of the inline function is inserted or substituted at the point where inline function is called.
Syntax-
inline return-type function-name(parameters)
{
// function code
}
Example of using inline
code-
#include <iostream>
using namespace std;
inline int add(int x,int y )
{
return(x+y);
}
int main()
{
cout<<"Addition of 'x'and 'y'is:"<<add(2,3);
return 0;
}
Output-
Addition of 'x'and 'y'is: 5
explanation-
In the above code whe the inline function is called then the add(2,3) is replaced by return(a+b) so the output is 5.
Now, we will discuss advantages ,disadvantages and limitations of using inline function.
Advantages of inline in C and C++
Advantages-
- Function call overhead doesnโt occur.
- As it doesn't call any functions, it doesn't need a stack on which we may push or pop variables.
- It also saves overhead of a return call from a function.
- Since an inline function produces less code than a regular function, it is mostly advantageous for embedded devices.
Disadvantages of inline in C and C++
Disadvantages-
- When we employ a lot of inline functions, the binary executable file grows in size as well, which increases the risk of thrashing and lowers computer performance.
- As we are using so many inline functions are used, the speed at which instructions are fetched from the cache memory to the primary memory can be decreased.
- For many embedded systems, inline functions are occasionally not beneficial since, in some circumstances, the size of the embedded is valued more highly than the speed.
Limitations of inline function
Limitations of inline functions-
There are some limitations to the inline functions which are as following-
- If a function contains a loop. (for, while, do-while)
- If a function contains static variables.
- If a function is recursive.
- If the return statement is absent from the function body and the return type of the function is not void.
- If a function contains switch or goto statement.
With this article at OpenGenus, you must have the complete idea of inline function in C and C++.