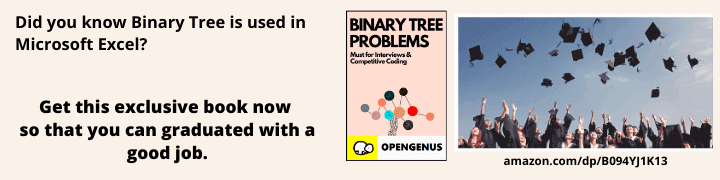
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
Perl is a general-purpose programming language with many applications. In this article, we look at Perl programming aspects such as files, regular expressions, subroutines, and Perl Object-Oriented Programming.
Table of contents.
- Introduction.
- Files.
- Regular expressions.
- Subroutines.
- Perl OOP.
- Summary.
- References.
Prerequisites.
Introduction.
Apart from being a useful tool for text processing and manipulation, Perl is also used in many other areas such as web development, network programming, automating system administration tasks in Linux, and more.
From the prerequisite article, we are introduced to Perl programming, we learned the basic concepts. In this article, we look at more advanced concepts needed to get us started in writing Perl scripts.
We look at programming aspects such as files, input/output, regular expressions, subroutines and how to write them, and object orientation in Perl programming language.
Files.
We may want to process data stored in a file, in Perl we use the open() function which takes the file as its argument.
The three basic modes of file handling are;
<: to only read the file
>: to create a new file, if one exists, it replaces it.
>>: to append to an already existing file.
Now, to read from the file.
First, we add some content to test_file.txt:
Then read from it:
open(r, "<", "test_file.txt");
# print file contents
print(<r>);
# closing the file
close(r);
To write to a file:
open(w, ">", "test_file.txt");
print w "THIS IS NEW CONTENT \n";
close(w);
To append to a file we write:
open(a, ">>", "test_file.txt");
print a "\n THIS IS APPENDED CONTENT \n";
close(a);
We can also use loops to read a file, this will do it line by line instead of reading the whole file at once:
# Looping through a file
my $test_file = 'test_file1.txt';
open(my $fl, $test_file) or die "Failed to open '$test_file' $!";
while(my $line = <$fl>){
chomp $line;
print "$line \n";
}
close $test_file
When done with a file, just like in other languages, we close the file. In Perl we close as follows:
close $file;
Regular expression.
To match a string in a text we write:
if(/searchString/){
/// action
}
When a search string is located after searching we can replace it by writing:
s/searchString/replaceString. An example:
$a =~ s/searchString/replaceString/;
This replaces the first found a match, to do this globally across the whole sentence or file we write s/searchString/replaceString/g:
An example of replacing a word using perl on the terminal:
perl -i -pe 's/NEW/REPLACE/g;' test_file.txt
Other regular expression matching constructs also apply here for example:
\d, matches all digits from 0 - 9.
\D, matches all non-digits.
\w, matches all word characters
\W, matches all non-words.
^, matches the start of a string.
$, matches the end.
\s, matches a whitespace character.
\S, matches a non-whitespace character.
Subroutines.
A subroutine is program instructions packaged as a unit that executes a specific task.
For example, let's write a subroutine for
sub routine{
my $arg = shift;
if($arg % 2 == 0){
print "Even! \n";
}else{
print "Odd! \n";
}
}
routine(4);
routine(9);
The shift passes its value as an argument to the function, that is we can call the function and pass a number:
Subroutines just like functions can also return, For example, let's get the factorial of a number:
# factorial
sub factorial{
my $n = shift;
if($n == 1){
return 1;
}else{
return ($n * factorial($n - 1));
}
}
print factorial(2), "\n";
print factorial(7), "\n";
Perl OOP.
In Perl, we have objects, methods and classes, An example of a class Car in Perl is shown below:
package Car;
sub new {
my $class = shift;
my $self = {
_name => shift,
_model => shift,
_year => shift,
};
print "This is a $self->{_name} $self->{_model} from the year $self->{_year}\n";
bless $self, $class;
return $self;
}
And now to create an object:
my $car_object = new Car( "Toyota", "Corolla", 1994);
Methods are similar to subroutines once created inside of a class, they can be used by its members.
Summary.
We have learned about file handling with Perl, regular expressions, subroutines, and a brief introduction to object-oriented programming in Perl.