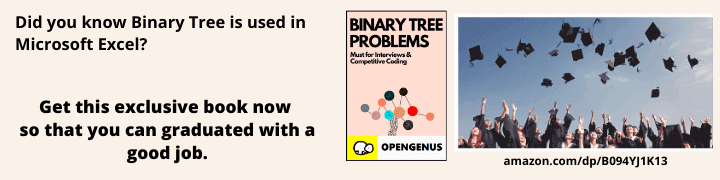
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
In this article, we have explained the approach to check which date comes earlier among two dates (in format DD:MM:YYYY) and implemented this approach in C Programming Language.
Table of contents:
- Problem Statement
- Approach
- Implementation in C
- Output
Problem Statement
In this problem, we have to implement a program in C Programming Language which takes 2 dates as input and output is the date which comes earlier/ first.
The dates will be in format DD:MM:YYYY.
For example, if the two dates are:
Date 1: 21:03:2009
Date 2: 19:02:2019
Then, date 1 comes first (21/03/2009).
Approach
The approach to check which date is earlier is as follows:
- The date format is in the form DD:MM:YYYY where DD is the date, MM is the month and YYYY is the year.
- Take 2 dates as input in the above format.
- If the year (YYYY) of the first date is less than the year of second date, then the first date comes first.
- Else if the year (YYYY) of the first date is greater than the year of second date, then the second date comes first.
- Else if the month (MM) of the first date is less than the month of the second date, then the first date comes first.
- Else if the month (MM) of the first date is greater than the month of the second date, then the second date comes first.
- Else if the date (DD) of the first date is less than the date of the second date, then the first date comes first.
- Else if the date (DD) of the first date is greater than the date of the second date, then the second date comes first.
Implementation in C
Following is the implementation in C programming language:
#include<stdio.h>
int main()
{
int a,b,c,d,e,f;
printf("Enter the first date=");
scanf("%d%d%d",&a,&b,&c);
printf("Enter the second date=");
scanf("%d%d%d",&d,&e,&f);
if(c>f)
{
printf("%d/%d/%d is earlier",d,e,f);
}
else if(c<f)
{
printf("%d/%d/%d is earlier",a,b,c);
}
else if(b<e)
{
printf("%d/%d/%d is earlier",a,b,c);
}
else if(b>e)
{
printf("%d/%d/%d is earlier",d,e,f);
}
else if(a<d)
{
printf("%d/%d/%d is earlier",a,b,c);
}
else
{
printf("%d/%d/%d is earlier",d,e,f);
}
return 0;
}
Output
Following is the command to compile and run the above C program:
gcc opengenus.c
./a.out
Sample input:
23
11
2012
23
07
2012
Output:
23/7/2012 is earlier
With this article at OpenGenus, you must have the complete idea of how to check which date comes earlier.