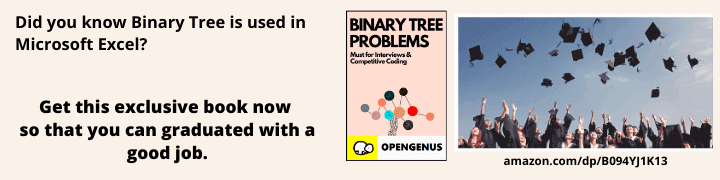
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
In this article, we have explained how to find the sum of two numbers and write a C program for it taking the two numbers as command line arguments.
Through this C program, you will learn the concepts of:
- Taking input as command line arguments
- There are different header files for different functions.
- How to convert char* (array of char) to float?
- How to print a variable of float datatype?
Table of contents:
- Problem Statement
- Solution
- Implementation
- Compile and run
- Time and Space Complexity
Problem Statement
In this problem, we have to take two numbers as input as command line arguments and output the sum of the two numbers. The numbers can be floating point numbers.
Write a program in C Programming Language for it.
For example, if the input numbers are 1.02 and 3.99, then the output will be 5.01.
The C program should generate an executable like a.out which will take input like:
./a.out 2 10
Solution
The approach to solve this problem and to implement it in C Programming Language is explained as follows:
- Step 1: Include the following two header files
#include <stdio.h>
#include <stdlib.h>
You will see, we will use printf() function of stdio header file to print the sum of the two numbers and atof() function of stdlib header file to convert a number from char*
datatype to float.
- Step 2: We define the main() function as follows:
int main(int argc, char** argv) {
// ...
return 1;
}
argc is the number of command line parameters passed and argv is the array of each command line parameter. By default, argc is 1 so if M parameters are passed, argc becomes M+1 and the parameters are argv[1], argv[2] and so on and each is in char* that is in string format.
- Step 3: Within the main() function, we need to check if the correct number of parameters has been passed.
if (argc != 3) {
printf("Wrong number of input parameters\n");
exit(1);
}
- Step 4: We need to get the two numbers from the command line argument and save them in variables of float datatype.
float number1 = atof(argv[1]);
float number2 = atof(argv[2]);
- Step 5: Do the sum of the above two numbers.
float sum = number1 + number2;
- Step 6: Finally, print the sum using printf().
printf("The sum of the two numbers %f and %f is %f", number1, number2, sum);
Implementation
Following is complete C program to add two numbers:
#include <stdio.h>
#include <stdlib.h>
int main(int argc, char** argv) {
if (argc != 3) {
printf("Wrong number of input parameters\n");
exit(1);
}
float number1 = atof(argv[1]);
float number2 = atof(argv[2]);
float sum = number1 + number2;
printf("The sum of the two numbers %f and %f is %f", number1, number2, sum);
return 1;
}
Compile and run
Save the above code in a file named "code.c" and use the following command to compile it:
gcc code.c -o sum
This will generate an executable file named "sum".
Run it as follows:
bash ./sum 4.56 9.01
The output will be:
The sum of the two numbers 4.56 and 9.01 is 13.57
Now, if we pass wrong number of command line parameters, then the error message will be generated.
bash ./sum
OR
bash ./sum 1 10 3 55
Output:
Wrong number of input parameters
Time and Space Complexity
If you are a beginner, you may not understand this part. This requires knowledge of Complexity Analysis in Algorithms and Data Structures.
We are using a direct addition of two numbers so the time and space complexity may seem to be O(1) but it is not.
If the input number is N, then the number of bits will be O(logN).
Addition has linear time complexity for the number of bits involved. So, for a number O(N), the time complexity of addition will be O(logN).
Hence, the time and space complexity of the program is O(logN). Now, as the input number is limited by 64 bits in most modern computer (if you are using primitive data type in C), then it is reasonable to assume that time and space complexity is constant that is O(1).
With this article at OpenGenus, you must have the complete idea of how to add two numbers in C Programming Language.