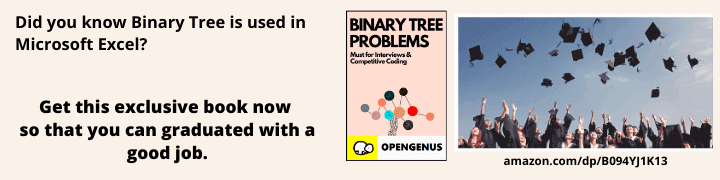
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
Reading time: 20 minutes | Coding time: 5 minutes
In Java, an inner class is a type of nested class. A nested class is a class which is defined inside of another class. Just as we nest the conditional statements such as if...else, switch and loops in the same way we can nest classes in Java. It provides better encapsulation.
The basic syntax of Inner class in Java is as follows:
class OuterClassName
{
//code
class InnerClassName
{
//code
}
}
To create an object of the Inner class, the syntax is as follows:
OuterClassName.InnerClassName inner =
new OuterClassName().new InnerClassName();
Uses of Inner class
- It can access private members of the outer class, which increases encapsulation.
- It is better to create a class as nested when it needs to be used only by one class.
- It makes our source code more readable and maintainable.
Types of Nested Classes
The different types of Nested classes in Java are:
- Non Static Nested Class (Inner Class)
- Method Local Inner Class
- Anonymous Inner Class
- Static Nested Class
Non Static Inner Class
A non-static nested class is also known as inner class which is just a simple nested class. It can access all variables of the outer class. If it is declared as private then it cannot be accessed by any other class except the class in which it is declared.
In this example, we will create a class A which will have class B as an inner class. We will create a object of class A and try to class B constructor.
Example
Java code for A.java:
//File name opengenus_A.java
class opengenus_A{
private int a = 10;
class opengenus_B{
public void print(){
System.out.println("a = " + a);
}
}
}
Java code for Main.java:
//File name Main.java
class Main{
public static void main(String[] args){
//this is the way of creating an instance of an inner class.
opengenus_A.opengenus_B inner = new opengenus_A().new opengenus_B();
inner.print();
}
}
Output:
a = 10
As you can see in the above example we have created a class B inside of class A and in B's print() method we are printing the value of A's private variable a.
Method Local Inner Class
When a class is declared inside a method then it is known as method local inner class. The scope of this type of inner class is only within the method it is declared. It can be instantiated only in the method it is defined.
In the following Java code example, notice that the inner class B is a part of the myMethod() function of class A. It is local to that function.
Example
//File name opengenus_A.java
class opengenus_A{
void myMethod(){
int a = 50;
class opengenus_B{
public void print(){
System.out.println("a = " + a);
}
}
opengenus_B b = new opengenus_B();
b.print();
}
}
Output:
a = 50
Anonymous Inner Class
An anonymous inner class is an inner class which is declared without any name. Like other anonymous classes we declare and instantiate it at the same time. Anonymouns inner classes are usefull for overriding methods.
Follow the following Java code example to understand it better.
Example
//File name B.java
abstract class opengenus_A{
public abstract void method();
}
public class opengenus_B{
public static void main(String[] args){
opengenus_A a = new opengenus_A(){
public void method(){
System.out.println("Inside anonymous class.");
}
};
a.method();
}
}
Output:
Inside anonymous class.
Static Nested Class
A static nested class is declated with the keyword static. Static classes cannot be called an inner class as they are just like other static members of a class. It cannot access the non-static variables of the outer class.
Follow the following Java code example to understand it better:
Example
class opengenus_A{
static int a = 100;
static class opengenus_B{
void print(){
System.out.println("a = " + a);
}
}
}
class Main{
public static void main(String[] args){
opengenus_A.opengenus_B b = new opengenus_B();
b.print();
}
}
In the above case we do not need to create an object of the outer class as the inner class is static and static members can be accessed without creating it's instance.
Output:
a = 100
If we create a static method inside of a static class then we can access it just like any normal static method of class.
With this article at OpenGenus, you have the complete knowledge of Inner classes in Java and its different kinds. Enjoy.