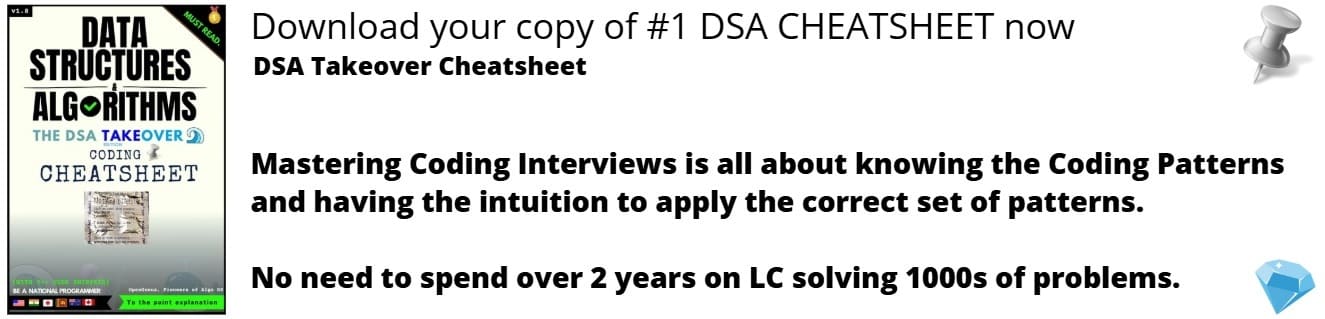
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
The java.lang.String class in Java provides a lot of methods to work on string. By the help of these methods, we can perform operations on string such as trimming, concatenating, converting, comparing, replacing strings etc.
String is a sequence of characters, for e.g. âHelloâ is a string of 5 characters. In java, string is an immutable object which means it is constant and can cannot be changed once it has been created.
JAVA String Class
There are two ways to create a String object:
-
By string literal : Java String literal is created by using double quotes.
For Example: String s=âWelcomeâ; -
By new keyword : Java String is created by using a keyword ânewâ.
For example: String s=new String(âWelcomeâ);
It creates two objects (in String pool and in heap) and one reference variable where the variable âsâ will refer to the object in the heap.
Java String Pool: Java String pool refers to collection of Strings which are stored in heap memory. In this, whenever a new object is created, String pool first checks whether the object is already present in the pool or not. If it is present, then same reference is returned to the variable else new object will be created in the String pool and the respective reference will be returned.
Difference between String literal and New String object
When you use new String( "Cosmos is Awesome" ), it explicitly creates a new and referentially distinct instance of a String object. It is an individual instance of the java.lang.String class. String s="Cosmos is Awesome"; may reuse an instance from the string constant pool if one is available (String Pool is a pool of Strings stored in Java heap memory ). Moreover, it is a Java language concept and when you use a string literal the string can be interned.
Ways to make new String
- Using String Literal : You can create String objects with String literal.
String str="Cosmos";
- Using new keyword : When we create string objects by using "new" keyword so the objects are stored in a special memory area known as "Heap".
String str= new String("Cosmos");
- Using character array : You could also convert character array into String here.
char ch[]={ 'C','o','s','m','o','s'};
String str1=new String(ch);
How String Works
Memory allotment of String
Whenever a String Object is created, two objects will be created- one in the Heap Area and one in the String constant pool and the String object reference always points to heap area object.
Interfaces and Classes in Strings in Java
- String : In java, objects of String are immutable which means a constant and cannot be changed once created.
String str="Cosmos"; //It is a Constant
- CharBuffer : CharBuffer is an abstract class of the java.nio package. CharBuffer is the buffer for characters. CharBuffer object is created by calling allocate(). We need to pass the capacity of CharBuffer to allocate method.
CharBuffer chbuff = CharBuffer.allocate(1024);
- StringBuffer : StringBuffer class is used to create a mutable string object i.e its state can be changed after it is created. It represents growable and writable character sequence.
StringBuffer str = new StringBuffer("Cosmos");
- StringBuilder : StringBuilder is a mutable sequence of characters. StringBuilder is used when we want to modify Java strings in-place. StringBuffer is a thread-safe equivalent similar of StringBuilder.
StringBuilder has methods such as append(), insert(), or replace() that allow to modify strings.
StringBuilder str = new StringBuilder();
str.append("Cosmos");
- StringTokenizer : n Java, the string tokenizer class allows an application to break a string into tokens. The tokenization method is much simpler than the one used by the StreamTokenizer class. The StringTokenizermethods do not distinguish among identifiers, numbers, and quoted strings, nor do they recognize and skip comments.
If you want to split by new line then you could use below:
String lines[] = String.split("\\r?\\n");
- StringJoiner : It can be used for joining Strings making use of a delimiter, prefix, and suffix.
StringJoiner joiner = new StringJoiner(",", PREFIX, SUFFIX);
joiner.add("Red")
.add("Green")
.add("Blue");
JAVA String Constructors
- String(byte[] byte_arr) : This constructs a new String by decoding the specified array of bytes using the platform's default charset.
byte[] b_arr = {67, 111, 115, 109, 111, 115};
String s_byte =new String(b_arr); //Cosmos
- String(byte[] byte_arr, Charset char_set) : This constructs a new String by decoding the specified array of bytes using the specified charset.
byte[] b_arr = {67, 111, 115, 109, 111, 115};
Charset cs = Charset.defaultCharset();
String s_byte_char = new String(b_arr, cs); //Cosmos
- String(byte[] byte_arr, String char_set_name) : This constructs a new String by decoding the specified array of bytes using the specified charset.
byte[] b_arr = {67, 111, 115, 109, 111, 115};
String s = new String(b_arr, "US-ASCII"); //Cosmos
- String(byte[] byte_arr, int start_index, int length) : This constructs a new String by decoding the specified subarray of bytes using the platform's default charset.
byte[] b_arr = {67, 111, 115, 109, 111, 115};
String s = new String(b_arr, 2, 5); //smos
- String(byte[] byte_arr, int start_index, int length, Charset char_set) : This constructs a new String by decoding the specified subarray of bytes using the specified charset.
byte[] b_arr = {67, 111, 115, 109, 111, 115};
Charset cs = Charset.defaultCharset();
String s = new String(b_arr, 2, 5, cs); //smos
- String(byte[] byte_arr, int start_index, int length, String char_set_name) :
This constructs a new String by decoding the specified subarray of bytes using the specified charset.
byte[] b_arr = {67, 111, 115, 109, 111, 115};
String s = new String(b_arr, 2, 5, "US-ASCII"); //smos
- String(char[] char_arr) : This allocates a new String so that it represents the sequence of characters currently contained in the character array argument.
char char_arr[] = {'C', 'o', 's', 'm', 'o', 's'};
String s = new String(char_arr); //Cosmos
- String(char[] char_array, int start_index, int count) : This allocates a new String that contains characters from a subarray of the character array argument.
char char_arr[] = {'C', 'o', 's', 'm', 'o', 's'};
String s = new String(char_arr , 2, 5); //smos
- String(int[] uni_code_points, int offset, int count) : This allocates a new String that contains characters from a subarray of the Unicode code point array argument.
int[] uni_code = {67, 111, 115, 109, 111, 115};
String s = new String(uni_code, 2, 5); //smos
- String(StringBuffer s_buffer) : This allocates a new string that contains the sequence of characters currently contained in the string buffer argument.
StringBuffer s_buffer = new StringBuffer("Cosmos");
String s = new String(s_buffer); //Cosmos
- String(StringBuilder s_builder) : This allocates a new string that contains the sequence of characters currently contained in the string builder argument.
StringBuilder s_builder = new StringBuilder("Cosmos");
String s = new String(s_builder); //Cosmos
JAVA String Methods
- char charAt(int index) : It returns the character at the index position of the string.
"Cosmos".charAt(3); // returns âmâ
- boolean equals( Object Obj) : Compares string with the given object.It is case sensitive
Boolean out = âCosmosâ.equals(âCosmosâ); // returns true
Boolean out = âCosmosâ.equals(âcosmosâ); // returns false
- boolean equalsIgnoreCase (String string) : Compares string with the given object.It is not case sensitive.
Boolean out = âCosmosâ.equalsIgnoreCase(âCosmosâ); // returns true
Boolean out = âCosmosâ.equalsIgnoreCase(âcosmosâ); // returns true
- int compareTo(String string) : Compares two string lexicographically.
int comapre = string1.compareTo(string2);
This returns difference string1-string2.
If :
compare < 0 // string1 comes before string2 lexicographically
compare = 0 // string1 and string2 are equal lexicographically.
compare > 0 // string1 comes after string2 lexicographically.
- int compareToIgnoreCase(String string) : Same as CompareTo method however it ignores the case during comparison.
int comapre = string1.compareToIgnoreCase(string2);
This returns difference s1-s2. If :
compare < 0 // string1 comes before string2 lexicographically.
compare = 0 // string1 and string2 are equal lexicographically.
compare > 0 // string1 comes after sstring2 lexicographically.
- int indexOf(ch) : Return index position of first occurrence of given character in given string
String str1 = new String("Cosmos");
int pos = str1.indexOf('s'); // 2 is stored in pos
- int lastindexOf(ch) : Return index position of last occurrence of given character in given string
String str1 = new String("Cosmos");
int pos = str1.lastindexOf('s'); // 4 is stored in pos
- String substring(int beginIndex) :It returns the substring of the string.
String str1 = new String("Cosmos is awesome");
String str2 = str1.substring(4) // "os is awesome" is stored in str2"
String str3 = str1.substring(4,8) // "os i" is stored in str2"
- String concat(String str) : Concats the string at the end of other string
String str1 = "Cosmos ";
String str2 = "is awesome."
String str3 = str1.concat(str2); // "Cosmos is awesome." is stored in str3
- String toLowerCase() : Converts all the characters of the String to lower case characters.
String str1 = "CoSmOs";
String str2 = str1.toLowerCase();// "cosmos" is stored in str2
- String toUpperCase() : Converts all the characters of the String to Upper case characters.
String str1 = "CoSmOs";
String str2 = str1.toLowerCase();// "COSMOS" is stored in str2
- String trim() : Removes whitespace from both the ends and gives a copy of the original string.
String str1 = " Cosmos ";
String str2 = str1.trim();// "Cosmos" is stored in str2
- int length : Finds the length of the given string.
String str1 = "Cosmos is awesome";
int l = str1.length();// 17 is stored in l
Question
String str1 = "Cosmos Community is very helpful";
String str2 = str1.toUpperCase();
System.out.println(str2);
What will be the output of the above code ?
With this article at OpenGenus, you must have the complete idea about String class in Java. Enjoy.