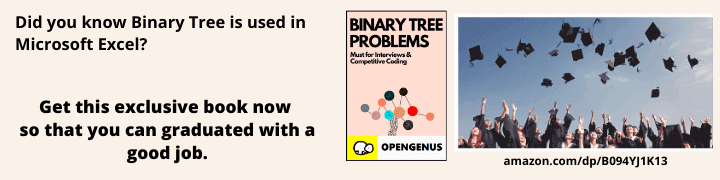
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
In this article at OpenGenus, we have solved the Mario less and Mario more problem in CS50. This involves printing a pyramid pattern.
Table of contents:
- Mario-less Programme
- Mario-more
Mario-less Programme
_
Problem statement
Design a program that allows the user to enter a positive integer between 1 and 8 as the height of a pyramid. The program should validate the input and then print a pyramid structure using the '#' symbol. Each row of the pyramid should have a number of bricks equal to the row number. The program should ensure that the pyramid is symmetrically aligned and properly spaced. Additionally, it should repeatedly prompt the user for a valid height until a correct value is entered. The goal is to create a visually appealing pyramid structure based on the user's input.
code in C
#include <stdio.h>
#include <cs50.h>
int main(void)
{
int input;
do
{
input = get_int("height: "); //take input
}
while (input < 1 || input > 8); //return input when input < 1 or input > 8
for (int row = 1; row <= input; row++)
{
int spaces = row <= input ? input - row : 0;
// spaces = till row is less than input print input - row times.
for (int j = 0; j < spaces; j++)
{
printf(" ");
}
for (int col = 1; col <= row; col++)
{
printf("#");
}
printf("\n");
}
}
Code explanation
- The program prompts the user to enter a positive integer between 1 and 8, inclusive, as the height of a pyramid.
- It uses the get_int function from the CS50 library to get the user input.
- The program continues to ask for input until a valid value is entered (between 1 and 8).
- The outer loop iterates over each row of the pyramid, from 1 to the input height.
- Inside the outer loop, a variable called spaces is calculated. It represents the number of spaces to be printed before the current row of the pyramid.
- If the current row is less than or equal to the input height, spaces is equal to input - row, where row is the current row number.
- If the current row is greater than the input height, spaces is set to 0.
- The first inner loop is responsible for printing the spaces before the pyramid's bricks on each row.
- The second inner loop prints the bricks (#) of the pyramid on each row. The number of bricks is equal to the current row number.
- Finally, a newline character is printed to move to the next row.
- The program exits after printing the complete pyramid.
input
> gcc mario-less.c -o mario-less
> ./mario-less
> height: 5
Output
#
##
###
####
#####
Mario-more
Double sided pattern.
Problem statement
The program aims to generate a pyramid structure based on user input. The objective is to prompt the user to enter the height of the pyramid within the range of 1 to 8. The program should then construct the pyramid with each row consisting of a combination of spaces and hash symbols, following a specific pattern. The program should ensure that the input is valid and meet the specified range.
#include <stdio.h>
#include <cs50.h>
int main(void)
{
int input;
do
{
input = get_int("Height: "); //get input
}
// return the input when input is less than 1 and input is greater than 8
while (input < 1 || input > 8);
for (int row = 1; row <= input; row++)
{
//spaces = till row is less than equal to input , print the statement input - row times.
int spaces = row <= input ? input - row : 0;
// spaces2 = till row is less than equal to input , print the statement two times.
int spaces2 = row <= input ? 2 : 0;
for (int j = 0; j < spaces; j++)
{
printf(" ");
}
for (int col = 1; col <= row; col++)
{
printf("#");
}
for (int j = 0; j < spaces2; j++)
{
printf(" ");
}
for (int col = 1; col <= row; col++)
{
printf("#");
}
printf("\n"); // print new line. so that all loop can take place on next line.
}
}
Code Explanation
_
- The code begins with the inclusion of necessary header files, stdio.h for input/output operations and cs50.h for the get_int function.
- Inside the main function, a variable named input is declared to store the user's input for the pyramid height.
- The code enters a do-while loop that prompts the user to enter the height of the pyramid using the get_int function. This loop will continue executing until a valid input within the range of 1 to 8 (inclusive) is provided.
- Following the input validation, a for loop is used to iterate through each row of the pyramid. The loop variable row represents the current row number.
- Inside the loop, a variable spaces is calculated to determine the number of spaces to be printed before each row. This value is calculated as the difference between the input value and the current row number.
- Next, a loop is used to print the required number of spaces before the pyramid's left side.
- Another loop is used to print the required number of # symbols for the left side of the pyramid.
- After printing the left side, another loop is used to print the required number of spaces for the gap between the two sides of the pyramid. This loop uses a separate variable spaces2, which is set to 2 for all rows except the last one.
- Finally, a loop is used to print the required number of # symbols for the right side of the pyramid.
- At the end of each row, a newline character is printed to move to the next line.
- Once all rows of the pyramid are printed, the program terminates.
Input
> gcc mario-more.c -o mario-more
> ./mario-more
> height: 5
Output
# #
## ##
### ###
#### ####
##### #####
With this article at OpenGenus, you must have the complete idea of solving the problem of Mario less and Mario more.