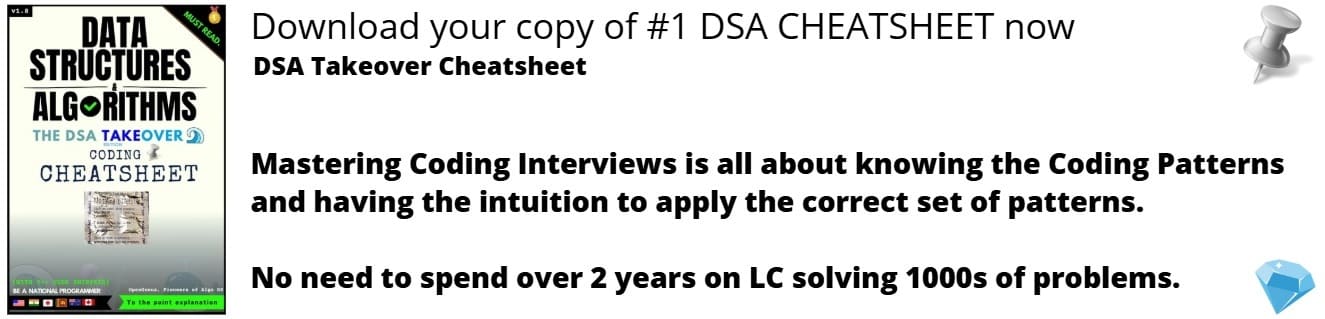
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
Reading time: 20 minutes | coding time: 2 minutes
Inheritance is one of the most important topic of object oriented programming.It provides reusability of code.
Multipath Inheritance in C++ is derivation of a class from other derived classes, which are derived from the same base class.This type of inheritance involves other inheritance like multiple, multilevel, hierarchical etc.
- Here class D is derived from class B and C.
- Class B and C are child of class A.
- From the above two points,we can say class D is indirectly derived from class A.
Example Code
#include <iostream>
#include <conio>
using namespace std;
class person
{
public:
char name[100];
int code;
void input()
{
cout<<"\nEnter the name of the person : ";
cin>>name;
cout<<endl<<"Enter the code of the person : ";
cin>>code;
}
void display()
{
cout<<endl<<"Name of the person : "<<name;
cout<<endl<<"Code of the person : "<<code;
}
};
class account:virtual public person
{
public:
float pay;
void getpay()
{
cout<<endl<<"Enter the pay : ";
cin>>pay;
}
void display()
{
cout<<endl<<"Pay : "<<pay;
}
};
class admin:virtual public person
{
public:
int experience;
void getexp()
{
cout<<endl<<"Enter the experience : ";
cin>>experience;
}
void display()
{
cout<<endl<<"Experience : "<<experience;
}
};
class master:public account,public admin
{
public:
char n[100];
void gettotal()
{
cout<<endl<<"Enter the company name : ";
cin>>n;
}
void display()
{
cout<<endl<<"Company name : "<<n;
}
};
int main()
{
master m1;
m1.input();
m1.getpay();
m1.getexp();
m1.gettotal();
m1.person::display();
m1.account::display();
m1.admin::display();
m1.display();
return 0;
}
Note: virtual keyword is used so that the grandchild class will get only one copy of parent class data members and functions.
Output:
Enter the name of the person : Chetali
Enter the code of the person : 1224
Enter the pay : 20000
Enter the experience : 2
Enter the company name : Hitech
Name of the person : Chetali
Code of the person : 1224
Pay : 20000
Experience : 2
Company name : Hitech
Limitations
- If virtual keyword is not used then the grandchild class will get two copies of data members from parent class.
- This type of inheritance is very ambiguous.