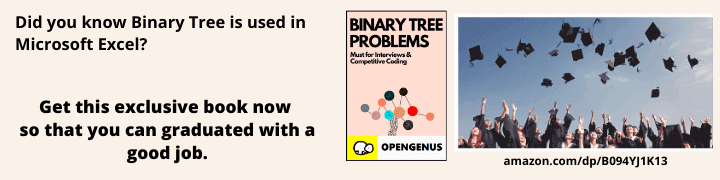
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
In this article, we have explained how to find the multiplication table of 2 numbers using functions and implement the technique in C Programming Language.
Table of contents
- Problem statement
- Approach to solve
- Implementation
- Output
Problem statement
In this problem, we have to find the multiplication table of 2 numbers using functions. Given a number N, we have to print the multiplication table till 10 that is:
N x 1 = ...
N x 2 = ...
...
N x 10 = ...
For example, if N = 5, then the multiplication table will be:
5 x 1 = 5
5 x 2 = 10
5 x 3 = 15
5 x 4 = 20
5 x 5 = 25
5 x 6 = 30
5 x 7 = 35
5 x 8 = 40
5 x 9 = 45
5 x 10 = 50
Similarly, implement a program in C Programming Language that will take 2 numbers as input and print the multiplication table for both numbers.
Approach to solve
Following is the approach to solve the problem:
- First we create a function multable(int a). int a inside the bracket is the parameter.
- Then inside this function we run a for loop from 1 to 10 as we have to find the multiplication table from 1 to 10.
- Now there are two ways to find the multiplication table
- First, we can simply multiply the given number by the loop variable
- Or we can initialise a variable sum of data type int with zero outside the loop and then print the result.
- Then we create the function main
- We create a variable named inputa and inputb of the data type int and take input through argv
- argv is an array of strings and it has to be converted to int. This can be done by atoi.
#include<stdlib.h>
is to be included at the top of the program before the functions as we have written atoi.- Then we pass the function.
Implementation
Following is the implemention of the code in C programming language:
#include<stdio.h>
#include<stdlib.h>
int multable(int a)
{
int sum=0;
for(int i=1;i<=10;i++)
{
sum=sum+a;
printf("%d x %d= %d\n",a,i,sum);
}
}
int main(int argc,char** argv)
{
int inputa=atoi(argv[1]);
int inputb=atoi(argv[2]);
multable(a);
multable(b);
return 1;
}
Output
Run the code as follows:
gcc code.c
./a.out 3 7
Following is the output of the program:
3x 1= 3
3 x 2= 6
3 x 3= 9
3 x 4= 12
3 x 5= 15
3 x 6= 18
3 x 7= 21
3 x 8= 24
3 x 9= 27
3 x 10= 30
7 x 1= 7
7 x 2= 14
7 x 3= 21
7 x 4= 28
7 x 5= 35
7 x 6= 42
7 x 7= 49
7 x 8= 56
7 x 9= 63
7 x 10= 70
With this article at OpenGenus, you must have the complete idea of how to find multiplication table of 2 numbers.