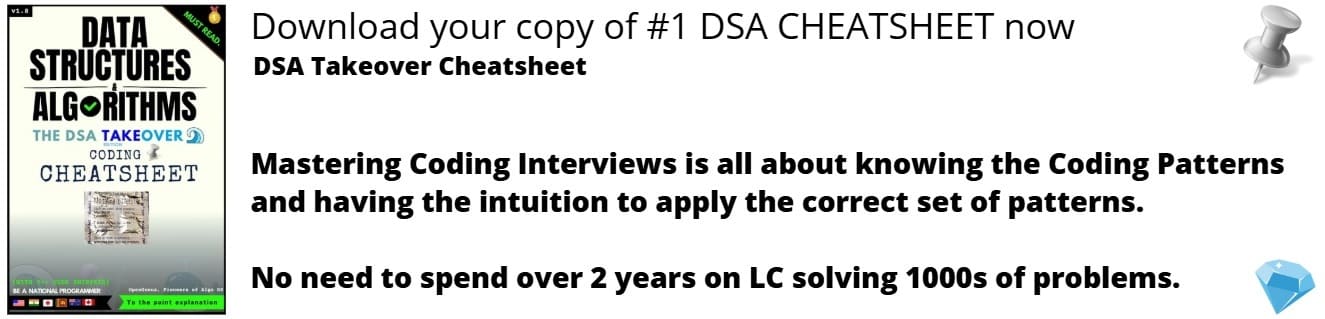
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
Reading time: 15 minutes
There are three basic OpenMP clauses namely firstprivate, lastprivate and ordered.
firstprivate
firstprivate clause is used to:
- initialize a variable from the serial part of the code
- private clause doesn't initialize the variable
Example of firstprivate
j = jstart;
#pragma omp parallel for firstprivate(j)
{
for(i=1; i<=n; i++){
if(i == 1 || i == n)
j = j + 1;
a[i] = a[i] + j;
}
}
lastprivate
lastprivate clause is used for:
- thread that executes the ending loop index copies its value to the master (serial) thread
- this gives the same result as serial execution
Example of lastprivate
#pragma omp parallel for lastprivate(x)
{
for(i=1; i<=n; i++){
x = sin( pi * dx * (float)i );
a[i] = exp(x);
}
}
lastx = x;
ordered
The ordered clause of OpenMP is used to:
- used when part of the loop must execute in serial order
- ordered clause plus an ordered directive
Example of ordered
#pragma omp parallel for private(myval) ordered
{
for(i=1; i<=n; i++){
myval = do_lots_of_work(i);
#pragma omp ordered
{
printf("%d %d\n", i, myval);
}
}
}