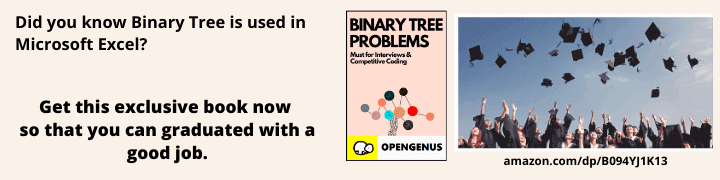
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
In this article, we have explained how to find the initials of a name and implement the technique in C Programming Language.
Table of contents
- Problem statement
- Approach to solve
- Implementation
- Output
Problem statement
In this problem, we have to find the initials of a name. Given a name N, we have to print the initials of the name followed by the full surname.
For example, if N = Ram Kumar Sharma, then the initials will be:
R.K.Sharma
We will implement this problem in C Programming Language.
Approach to solve
Following is the approach to solve the problem:
- First we take the input of the name in a variable named name.
- Then we start a for loop and measure the length of the name by given the condition that
name[i]
is not equal to the new line character. - We initialise a variable len with the value 0 and increment it till it satisfies the above condition.
- Now we count the number of spaces, for that we give the condition that
name[i]
is equal to space. If true variable count will be incremented by 1. - Now we again start a for loop from 0 to len.
- First we print the first letter by
name[0]
then break the loop. - Now we start another loop from 0 to len. We then check if
name[i]
is equal to space or not. If true then we check if c is less than count or not(we create a variable c at the beginning of the program and initialise it with a value 1).If this is true then we printname[i+1]
that is the character after space and increment the value of c by 1.If the above condition is false then it exits the loop and enters the next loop. - In the next loop we again check for a space, if true we had initialised a variable d with 0 at the beginning of the program, we increment it by 1.
- Now if d is equal to count, then we print
name[i+1]
that is the character after space.
Implementation
Following is the implemention of the code in C programming language:
#include<stdio.h>
int main(int argc, char** argv)
{
char name[100];
int count=0,len=0,c=1,d=0;
printf("Enter the name");
scanf("%[^\n]s",name);
int i;
for(i=0;i<100;i++)
{
if(name[i]!='\n')
{
len++;
}
if(name[i]==' ')
{
count++;
}
}
for(i=0;i<len;i++)
{
printf("%c.",name[0]);
break;
}
for(i=0;i<len;i++)
{
if(name[i]==' ')
{
if(c<count)
{
printf("%c.",name[i+1]);
c++;
}
}
}
for(i=0;i<len;i++)
{
if(name[i]==' ')
{
d++;
}
if(d==count)
{
printf("%c",name[i+1]);
}
}
return 0;
}
Output
Run the code as follows:
gcc code.c
./a.out
Following is the output of the program:
Enter the name Ram Kumar Sharma
R.K.Sharma