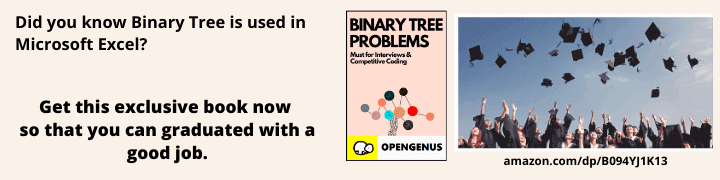
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
In this article, we have explored how to find Maximum, Minimum, Average of 3 numbers and implement the technique in C Programming Language.
Table of contents
- Problem statement
- Approach to solve
- Implementation
- Output
Problem statement
In this problem, we have to find the Maximum, Minimum, Average of 3 numbers.
For example, a=3, b=5, c=9
max=9 min=3 avg=5.666667
We will implement this problem in C Programming Language.
Approach to solve
Following is the approach to solve the problem:
- We first create a function max.
- For maximum we are using call by value and for minimum and average we use call by reference.
- Inside the main function we first take the input of three numbers.
- Then we call the function and store it in a variable d.
- Now the control goes to the function max were we find the maximum, minimum and average of three numbers.We store the maximum value in a variable k and return it.
- Now the control goes back to the main function where we had called the function max.
- Finally it prints the three answers
Implementation
Following is the implemention of the code in C programming language:
#include<stdio.h>
int max(int,int,int, float *,int*);
int main()
{
int a,b,c,d,f;
float e;
printf("Enter three values");
scanf("%d%d%d",&a,&b,&c);
d=max(a,b,c,&e,&f);
printf("%d %f %d",d,e,f);
}
int max(int p,int q,int r, float *e,int *g)
{
int k;
*e=(p+q+r)/3.0;
k=(p>q)?((p>r)?p:r):((q>r)?q:r);
*g=(p<q)?((p<r)?p:r):((q<r)?q:r);
return k;
}
Output
Run the code as follows:
gcc code.c
./a.out
Following is the output of the program:
Enter three values3
5
9
9 5.666667 3
With this article at OpenGnus, you must have the complete idea to find Maximum, Minimum, Average of 3 numbers in C.