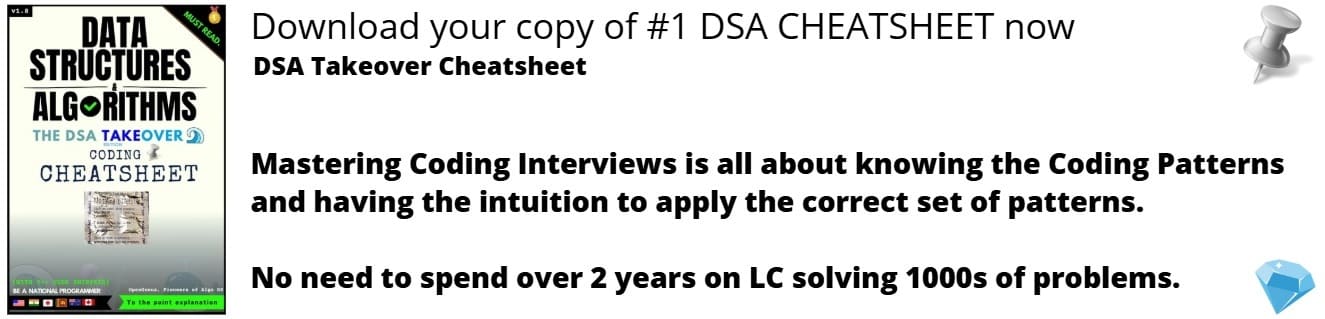
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
In this article, we have explained how to swap three numbers using functions and implement the technique in C Programming Language.
Table of contents
- Problem statement
- Approach to solve
- Implementation
- Output
Problem statement
In this problem, we have to swap three numbers using functions.
For example, a=5, b=7, c=9
then after swaping, a=7, b=9, c=5
We will implement this problem in C Programming Language.
Approach to solve
Following is the approach to solve the problem:
- First we create a function swap with the return type void.
- Then inside the main function we take the input of three numbers then call the function swap.
- The control now goes to the function swap.
- We now create a fourth variable and swap the values.
- This program is an example call by reference so we take the parameters of the function as the address of the variable and we swap the pointer value of the variable.
- This way the values are swaped in the main function also.
Implementation
Following is the implemention of the code in C programming language:
#include<stdio.h>
void swap(int,int,int);
int main()
{
int a,b,c;
printf("Enter three values");
scanf("%d%d%d",&a,&b,&c);
swap(&a,&b,&c);
printf("%d %d %d",a,b,c);
}
void swap(int *p,int *q,int *r)
{
int temp;
temp=*p;
*p=*q;
*q=*r;
*r=temp;
}
Output
Run the code as follows:
gcc code.c
./a.out
Following is the output of the program:
Enter three values5
7
9
7 9 5
With this article at OpenGenus, you must have the complete idea of how to Swap three numbers using functions in C Programming Language.