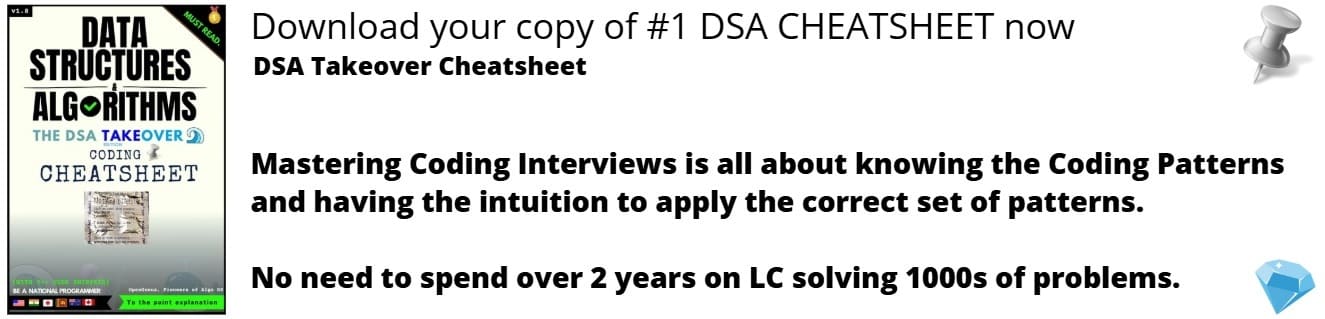
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
In this article, we have explained how to return last two digits of an Integer using functions and implemented the technique in C Programming Language.
Table of contents
- Problem statement
- Approach to solve
- Implementation
- Output
Problem statement
In this problem, we have to return last two digits of a number using functions.
For example, if input is 139245, then the last two digits (that is two least significant digits) are 45.
Approach to solve
Following is the approach to solve this problem:
- First we create a function digit(int a). int a inside the bracket is the parameter.
- Then inside this function we return the last two digits of the number.
- We do modulus 100 of the number and store it in a variable b of data type int and return it.
- Then we create the function main
- We create a variable named input of the data type int and take input through argv
- argv is an array of strings and it has to be converted to int. This can be done by atoi.
#include<stdlib.h>
is to be included at the top of the program before the functions as we have written atoi.- Then we create a variable named answer to store the return type and then print it.
139245
last digit = 139245 % 10 = 5
last 2 digits = 139245 % 100 = 45
Implementation
Following is the implemention of the code in C programming language:
#include<stdio.h>
#include<stdlib.h>
int digit(int a)
{
int b=a%100;
return b;
}
int main(int argc,char** argv)
{
int input=atoi(argv[1]);
int answer=digit(input);
printf("The last two digits of the number are %d",answer);
return 1;
}
Output
Run the code as follows:
gcc code.c
./a.out 789
Following is the output of the program:
The two digits ater decimal point are 89
With this article at OpenGenus, you must have the complete idea of how to return last two digits of an Integer in C.