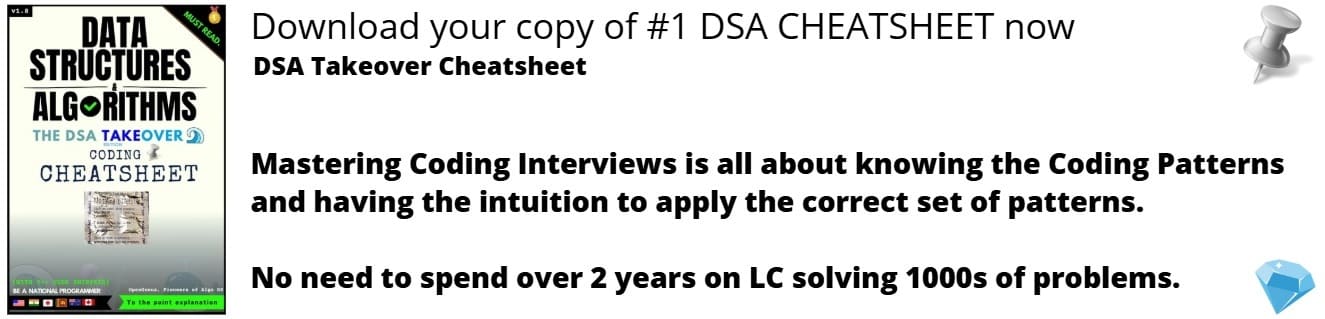
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
In this article, we have explained how to return two digits after decimal point using functions and implemented the technique in C Programming Language.
Table of contents
- Problem statement
- Approach to solve
- Implementation
- Output
Problem statement
In this problem, we have to return two digits ater decimal point using functions.
For example, if the input number is 5412.921445, then the first two digits after decimal point are 92.
Approach to solve
Following is the approach to solve the problem:
- First we create a function digit(double a). double a inside the bracket is the parameter.
- Then inside this function we return the two digits ater decimal point.
- First we multiply the number by 100.0 as the original number is of the data type double and then typecast it to int.
- Then we do modulus 100 to the above result and again typecast it to int as we want an integer result.
- Then we create the function main
- We create a variable named input of the data type double and take input through argv
- argv is an array of strings and it has to be converted to double. This can be done by atof.
#include<stdlib.h>
is to be included at the top of the program before the functions as we have written atof.- Then we create a variable named answer to store the return type and then print it.
Number: 5412.921445
N x 100 = 5412.921445 x 100
= 541292.1445
round = 541292
last 2 digits = N % 100
= 541292 % 100
= 92
Implementation
Following is the implemention of the code in C programming language:
#include<stdio.h>
#include<stdlib.h>
int digit(double a)
{
return (int)((int)(a*100.0))%100;
}
int main(int argc,char** argv)
{
double input=atof(argv[1]);
int answer=digit(input);
printf("The two digits ater decimal point are %d",answer);
return 1;
}
Output
Run the code as follows:
gcc code.c
./a.out 5.789
Following is the output of the program:
The two digits ater decimal point are 78
With this article at OpenGenus, you must have the complete idea of how to return two digit after decimal point in C.