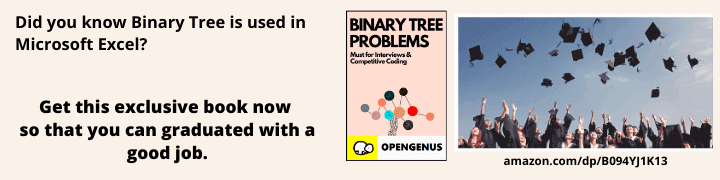
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
In this article, we have explained how to copy a struct in C Programming Language to another struct. We have covered different cases including Shallow and Deep copy.
Table of Contents
- Introduction
- Copy by assignment to structure variable
- Deep Copy and Shallow Copy
- Deep Copy of struct in C
- Shallow Copy of struct in C
- Conclusion
INTRODUCTION
What is a struct?
A structure is a collection of variables of various data types that can be manipulated together. Using a structure has the advantage of making coding easier and making the code easier to understand. In this article we will learn different ways to copy struct in C language.
Copying is to reuse some or all of the data of the original object, and create a new object by copying the original object.
In programming it is the process of making an exact copy of the object or variable, i.e., both the object or variables must have the same value.
When you create a copy of something, you expect it to be an independent object and similar to the thing you copied. In programming that definition can vary in many ways. So, we are also going to study in depth about shallow and deep copy.
Copy by assignment to structure variable
Approach Explained:
A variable defined in a structure is called a structure variable .
Since this structure variable is a variable, it can be assigned to another variable.
If the assignment destination variable has the same structure as the assignment source variable, the assignment expression can copy the structure variable as shown in the following implemented code.
Implemented Code:
#include <stdio.h>
struct Animal {
int age ;
char name [ 40 ];
};
int main ( void ) {
struct Animal cat = { 2 , "Kat" };
struct Animal dog = cat ;
printf ( "dog age[%d] name[%s] \n " , dog . age , dog . name );
return 0;
}
Output:
dog age[2] name[Kat]
Deep Copy and Shallow Copy
In programming to make a copy means to store the data in different variables.
However there is an extra factor to consider in all of this. That is whether the copy is a deep copy or a shallow copy. To understand copying in C language we have to first understand how C stores data first.
A deep copy means the data saved to a variable is independent of the original data.
A shallow copy means the data is still in someway connected to the original variable.
Deep Copy of struct in C
What is a deep copy?
Deep copy refers to copying the specific content of the object. Deep copy opens up a new memory address in the computer to store the copied object. Changes to the source data do not affect the replicated data.
Why use deep copy?
We hope that when changing the new array (object), the original array (object) will not be changed.
Implemented Code:
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
typedef struct Student
{
char *name;
int age;
}Student;
int main(int argc, char *argv[])
{
Student std1;
Student std2;
std1.name = (char *)malloc(10);
std1.age = 20;
strcpy(std1.name, "John");
printf("std1->name: %s, age: %d\n", std1.name, std1.age);
std2 = std1;
std2.name = (char *)malloc(10);
strcpy(std2.name, std1.name);
printf("std2->name: %s, age: %d\n", std2.name, std2.age);
free(std1.name);
free(std2.name);
return 0;
}
Output:
std1->name: John, age: 20
std2->name: John, age: 20
Code Explained:
In order to avoid for the structure containing pointer variables, and the pointer variable members in the structure point to a section of dynamically allocated memory space, we can use deep copy in the process of copying each other.We copy std1 to std2, the member name of std2 points to a new space again, and assigns the content of the space pointed to by std1.name to the space pointed to by std2.name.
The "copy" of the structure is successful.
Shallow Copy of struct in C
What is a shallow copy?
Implemented Code:
#include <stdlib.h>
#include <string.h>
typedef struct student
{
char *name;
int age;
}student;
int main()
{
student t1;
t1.name = (char*)malloc(30);
strcpy(t1.name, "John");
t1.age = 22;
student t2 = t1;
printf("%s %d", t2.name, t2.age);
if (t1.name != NULL)
{
free(t1.name);
t1.name = NULL;
}
return 0;
}
Output :
John 22
Code Explained:
First, we define a structure Student, and declare two structure variables t1 and t2 in the main function. Next, we open up a space in the heap area for the name of t1, and use the strcpy function to copy to the space area pointed to by name. Finally we use t1 to assign directly to t2.
The member names of t1 and t2 point to the same memory area in our computer.
Conclusion
With this article at OpenGenus, you must have the complete idea of how to copy struct in C.
Copying is to reuse some or all of the properties of the original object and create a new object based on the meta object.
When copying, all properties in an object are copied, including Object and array properties. This copy is called deep copy.
When copying, only part of the properties are copied, and Object and array properties are not copied, this kind of copy is called shallow copy.