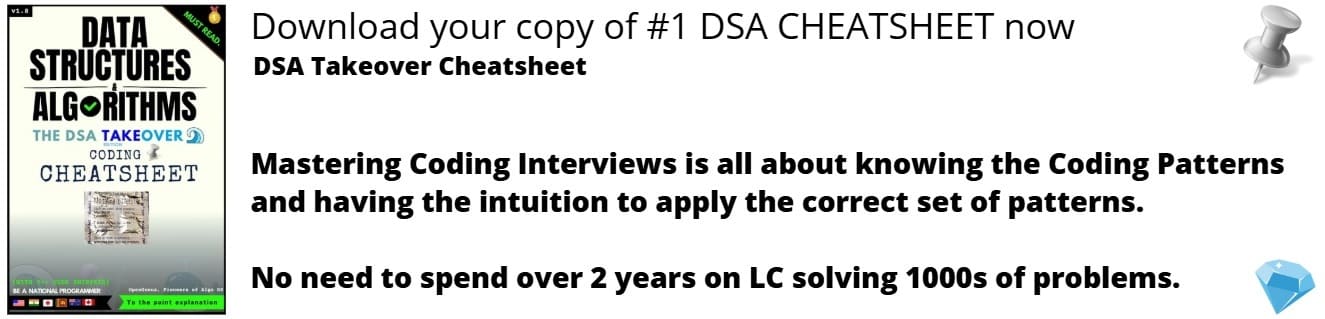
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
Reading time: 45 minutes
Set is a data structure that is used as a collection of objects. Java supports it through its Java Collection library. There are several ways to implement a set and Java supports three different implementations along with useful operations like intersection between sets.
Key ideas:
-
A set is a collection of objects like integers
-
It allows insertion of elements in the set and to search an element
-
Java set is an interface which extends collection.
-
set can not contain duplicate elements .
-
Java Set allow at most one null value.
-
Set interface contains only methods inherited from Collection.
-
Set provides basic Set operation like union, intersection and difference between sets as well
-
Java Provide three general purpose Set implementations :
- HashSet: using a hash map behind the set
- TreeSet: using a tree behind the set
- LinkedHashSet: using a linked list as a collison management technique in the hash map which is used behind the set
- this all three Set implementaions use different data structures to store elements.
This diagram captures the dependency between the various set implementations in Java:
How to define a set in Java?
To define a HashSet with integer datatypes, use the following code:
Set<Integer> s = new HashSet<Integer>();
To define a TreeSet with integer datatypes, use the following code:
Set<Integer> ts = new TreeSet<Integer>();
To define a LinkedHashSet with integer datatypes, use the following code:
Set<Integer> ls = new LinkedHashSet<Integer>();
How to add an element to a set? : add()
- add method is use to store element into set. it will Adds the specified element to this set if it is not already present.
Example:
s.add(1);
Java code to add element into Set :
import java.io.*;
import java.util.*;
public class SetAddExample{
public static void main(String[] args)
{ //set implementation using HashSet
Set<Integer> s = new HashSet<Integer>();
s.add(3);
s.add(20);
s.add(12);
System.out.println("Set Implementation Using HashSet :");
System.out.println(s);
// set implementation using LinkedHashSet
LinkedHashSet<Integer> ls =
new LinkedHashSet<Integer>();
ls.add(3);
ls.add(20);
ls.add(12);
System.out.println("Set Implementation Using LinkedHashSet :");
System.out.println(ls);
// set implementation using treeset
Set<Integer> ts = new TreeSet<Integer>();
ts.add(3);
ts.add(20);
ts.add(12);
System.out.println("Set Implementation Using TreeSet :");
System.out.println(ts);
}
}
Output :
Set Implementation Using HashSet :
[3, 20, 12]
Set Implementation Using LinkedHashSet :
[3, 20, 12]
Set Implementation Using TreeSet :
[3, 12, 20]
- Based on the above output we can say that TreeSet store element in sorted order.
Complexity Of add Method :
- HashSet add method have constant time complexity O(1).
- TreeSet add method have constant time complexity O(log(n)).
- LinkedHashSet add method have constant time complexity O(1).
How to remove element from a set? : remove()
- remove method is use to remove elements from set.
Java code to remove elements from Set :
import java.io.*;
import java.util.*;
public class SetRemoveExample{
public static void main(String[] args)
{ //set implementation using HashSet
Set<Integer> s = new HashSet<Integer>();
s.add(3);
s.add(2);
s.add(12);
System.out.println("Set Output Before Remove Operation :");
System.out.println(s);
s.remove(3);
System.out.println("Set Output After Remove Operation :");
System.out.println(s);
}
}
Output :
Set Output Before Remove Operation :
[2, 3, 12]
Set Output After Remove Operation :
[2, 12]
Complexity Of remove Method :
- HashSet remove method have constant time complexity O(1).
- TreeSet remove method have constant time complexity O(log(n)).
- LinkedHashSet remove method have constant time complexity O(1).
Some important methods :
1. clear() :
- remove all the elements from the Set.
Java code to remove all elements from Set :
import java.io.*;
import java.util.*;
public class SetClearExample{
public static void main(String[] args)
{ //set implementation using HashSet
Set<Integer> s = new HashSet<Integer>();
s.add(3);
s.add(2);
s.add(12);
System.out.println("Output Before Clear Operation :");
System.out.println(s);
s.clear();
System.out.println("Output After Clear Operation :");
System.out.println(s);
}
}
Output:
Output Before Clear Operation :
[2, 3, 12]
Output After Clear Operation :
[]
2. contains(Object o) :
- returns true if this set contains the specified element.
Java code for contains() method :
import java.io.*;
import java.util.*;
public class SetContainExample{
public static void main(String[] args)
{ //set implementation using HashSet
Set<Integer> s = new HashSet<Integer>();
s.add(3);
s.add(2);
s.add(12);
s.add(15);
if (s.contains(15)==true)
System.out.println(15+""+" is belong to Set");
if (s.contains(30))
System.out.println(30+""+" is belong to Set");
else
System.out.println(30+""+" is not belong to Set");
}
}
Output:
15 is belong to Set
30 is not belong to Set
3. equals(Object o) :
- Compares the specified object with this set for equality.
import java.io.*;
import java.util.*;
public class SetEqualsExample {
public static void main (String[] args) {
Set<Integer> s = new HashSet<Integer>();
s.add(11);
s.add(22);
s.add(33);
s.add(44);
Set<Integer> ts =new TreeSet<Integer>();
ts.add(11);
ts.add(22);
ts.add(33);
ts.add(44);
Set<Integer> ls =new LinkedHashSet<Integer>();
ls.add(11);
ls.add(22);
ls.add(33);
if (ts.equals(s))
System.out.println("True");
else
System.out.println("False");
if(s.equals(ls))
System.out.println("True");
else
System.out.println("False");
}
}
Output:
True
False
4. size() :
returns the number of elements in this set (its cardinality).
Java code to get size of the Set :
import java.io.*;
import java.util.*;
public class SetSizeExample{
public static void main(String[] args)
{ //set implementation using HashSet
Set<Integer> s = new HashSet<Integer>();
s.add(3);
s.add(2);
s.add(12);
s.add(15);
System.out.println("size of set is :");
System.out.println(s.size());
}
}
Output:
size of set is :
4
5. addAll(Collection<? extends E> c) :
- returns an array containing all of the elements in this set.
- Basically addAll() methode give an union of two sets.
Java code for union of the Sets :
import java.io.*;
import java.util.*;
class SetUnionExample {
public static void main (String[] args) {
Set<Integer> s = new HashSet<Integer>();
s.add(1);
s.add(4);
s.add(6);
s.add(5);
Set<Integer> ts =new HashSet<Integer>();
ts.add(1);
ts.add(2);
ts.add(3);
ts.add(4);
ts.addAll(s);
System.out.println("Union Of two set :")
System.out.println(ts);
}
}
Output:
Union Of two set :
[1, 2, 3, 4, 5, 6]
6. retainAll(Collection<?> c) :
- retainAll method is useful to find intersection of two set.
- retainAll method ratain all common element of two set.
import java.io.*;
import java.util.*;
class SetIntersectionExample {
public static void main (String[] args) {
Set<Integer> s = new HashSet<Integer>();
s.add(1);
s.add(4);
s.add(6);
s.add(5);
Set<Integer> ts =new HashSet<Integer>();
ts.add(1);
ts.add(2);
ts.add(3);
ts.add(4);
ts.retainAll(s);
System.out.println("Intersection Of two set :");
System.out.println(ts);
}
}
Output:
Intersection Of two set :
[1, 4]
HashSet vs TreeSet vs LinkedHashSet:
- HashSet :
- HashSet Use hashtable to store elements.
- Elements are not stored in sorted order(unsorted).
- add,remove,contain method have constant time complexitiy O(1).
- TreeSet :
- TreeSet Use RedBlack tree to store elements.
- Elements are stored in sorted order (sorted).
- add, remove, and contains methods has time complexity of O(log (n)).
- LinkedHashSet :
- LinkedHashSet is between HashSet and TreeSet. It is implemented as a hash table with a slinked list running through it.
- The time complexity of basic methods is O(1).
HashSet has best-performing implementation of Set compare to LinkedHashSet and TreeSet .
Applications of Set
- We can use Set interface to maintain data uniqueness.
- Set is used to avoid data redundancy.