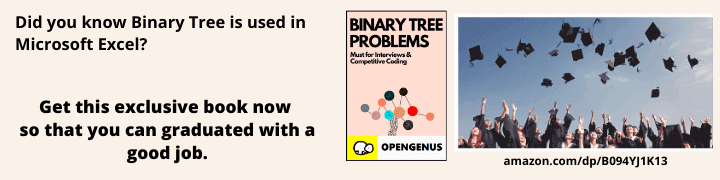
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
In this article, we will have a look at what actually is Structure, Union and Enumeration in C and will have look at differences between them.
Table of contents :
- Introduction and need
- Structure in C
- Union in C
- Enumerations in C
- Differences between them (table included)
Introduction:
Every rookie C programmer when comes to know the concept of array, is fascinated by the working principle of it. But as soon as they get to know that an array in C cannot store non-primitive data type by default, disappointment knocks. But here comes Structures, Unions and Enumerations to our rescue. They are real heroes in C.
Need of structure and Union:
Let us take an example by seeing why we even need Structures and Unions in C language.
It is fine till the point you want to store Math grades of 10 students starting from Roll. No 1 till Roll.No 10. Here, you'll simply need an array of size 11 where the index of the array denotes the Roll.No of student (since 0 is not a valid Roll. No in most schools :-) ) and the value stored at i th index is actually the grade of that with Roll.No i. But the default implementation of C array will definitely fail where you want to store the data collectively. i.e. You want to store the name of the student, Roll. No of student, Marks collectively at one place. Here we need to use the aforementioned terms. Let us look at them one by one.
Structures:
Structures in C language is one of the way to collectively group variables of different data-types that closely relate to each other. e.g in the above case, we needed to group variables related to a student such as student name, student roll, student grades etc. i.e a structure in C can store variables of different data types. Now you cannot only store math grade but pretty much every grade associated with student.
Syntax:
struct Student { // Structure declaration
int roll_no; // Member (int variable)
char* name; // Member (char* variable)
float math_grades; // Member (float variable)
float sociology_grades; // Member (float variable)
};
Some important points about structure in C:
- A structure in C language is defined with the help of struct keyword.
- Each variable present is called as member variable of that structure.
- A structure strictly needs to end with a semicolon (;).
- To access the structure, we must first create a reference of it.
- To create a reference variable of a structure, we have to use a struct keyword. That's a standard C syntax.
- Structure is often referred as a user-defined data type.
- Every single variable is assigned a different memory location in the physical memory.
- Structures cannot directly contain functions.
- When a structure is declared, memory is allocated for its members and initially they contain garbage value.
Accessing Members of Structure:
To access the members of a structure in C, you simple need to use the member access operator (.) on the reference created.
e.g.
struct Student { // Structure declaration with name
int roll_no; // Member (int variable)
char* name; // Member (char* variable)
float math_grades; // Member (float variable)
};
//Here size of strucutre will be (4 Bytes + 8 Bytes + 4 Bytes = 16 Bytes)
int main() //Entry Point
{
struct Student peter; // Confused why we used struct keyword?
// Refer point 5 in important points.
peter.roll_no = 12; // Peter's Roll. No is set to 12
printf("%d",peter.roll_no); // Accessing the peter's Roll.No
// through member access operator
}
Similarly you can assign and access other properties related with peter.
One more syntax of declaring structure could be :
struct Student peter ={ 12, "Peter", 90.0}; //Note curly braces here.
However, in this format, the order of inserting values must match order of data type of declared originally in the structure.
Unions in C:
Union in C is exactly same as the structure and also meant for the same purpose, except the only fact that Union in C uses something called as shared memory.
Some important points about union in C:
- A union in C language is defined with the help of union keyword.
- Each variable present is called as member variable of that union.
- A union definition strictly needs to end with a semicolon (;).
- To access the union, we must first create a reference of it.
- To create a reference variable of a union, we have to use a union keyword prior to it.
- Union is also often referred as a user-defined data type.
- In Union, unlike structure, each variable is not assigned a different physical memory location. Instead for one union, only one block of physical memory is assigned whose size is size of largest data-type in structure.
- When we assign a value to a union variable, the value is always stored in the same memory location. This means that if we change the value of one member of the union, the values of the other members will be overwritten.
e.g
union union_name {
int i;
float f; // Here Size of union will be 4 Bytes as float is the
char c; // largest data type it has and float is of 4 Bytes
};
Note that this is not a good idea to show how to efficiently use union. This is just to make you comfortable with syntax of union and its working.
union Student {
int roll_no;
float grades;
char* name ; // Here this entity (pointer to char array) is largest of
// all three and hence size of union will be
// size of this(i.e. 8 Bytes)
};
void main(){
union Student martin;
martin.roll_no = 10; // Same shared memory will be used.
printf("%d",martin.roll_no); // 10
martin.name ="martin"; // Same shared memory is used.
printf("%s",martin.name); //martin
}
Key Takeaway:
Unions are far more efficient than structures in terms of Memory usage and hence it is strongly recommended to use unions where data sets in multiple grouped entities are overlapping each other.
Enumeration in C:
Before diving into technical jargon, let us have a look at simple meaning of Enumeration in common English. It states that Enumeration is: the action of mentioning a number of things one by one.
In the same sense, in programming, enums( shorthand for Enumeration ) are used to assigned real-life names to integer-based constants.
Like structure and unions, enums are also user defined data types in C.
Syntax:
enum Enum_name{word_for_0, word_for_1, word_for_2 ... and so on};
enum week{Monday, Tuesday, Wednesday, Thursday};
In above example, Monday represents integer 0, Tuesday represents integer 1, Wednesday 2 and so on.
Some important points about enum in C:
- A enum in C language is defined with the help of enum keyword.
- A enum definition strictly needs to end with a semicolon (;).
- To create a reference variable of a enum, we have to use enum keyword prior to it.
- Enum is also often referred as a user-defined data type.
- Two enum variables can represent same integer constant (i.e.they can have same value.)
- If we do not explicitly assign values to enum names, the compiler by default assigns values starting from 0.
- The value assigned to enum names must be some integral constant, i.e., the value must be in range from minimum possible integer value to maximum possible integer value.
( INT_MIN < enumvalue < INT_MAX )
Sample Program:
enum week{Monday, Tuesday, Wednesday, Thursday, Friday, Saturday, Sunday};
int main()
{
enum week day;
day = Wednesday; // As wednesday represents 2 in above case
printf("%d",day);
return 0;
}
Output : 2
Differences between Structure,Union and Enumeration
Although Structure, Union and Enumeration are meant to provide similar functionality, still there are some subtle differences between them which will help us to get good grasp on topic and worth to note. Let us look at them in tabular format to summarize.
Sr. No | Structure | Union | Enumeration |
---|---|---|---|
1 | A structure in C is defined with struct keyword | A union in C is defined with union keyword | A Enumeration in C is defined with enum keyword |
2 | A structure is collection User-Defined Data types stored at different memory locations. | A union is collection User-Defined Data type stored at same memory location. | A Enumeration User-Defined data type for representing integer-based constants. |
3 | Size of a structure is summation of size of individual elements within it. | Size of Union is size of the largest element in the Union. | Size of enum is 4 X (number of constants inside) as each constant is an integer of 4 bytes |
4 | Structure is not memory efficient where repetitive occurrences of similar data elements are present as for every single repetitive occurrence, a new location in physical memory will be created. | Union is memory efficient where repetitive occurrences of similar data type are present as for every similar occurrence, only one location of physical memory is used. | Enumeration is only efficient if we want to deal in terms of fixed number of constants. |
5 | Structures can be nested inside Unions. | Unions can be nested inside structures | Enumerationcan be nested inside both structures and unions. |
6 | Unlike class, structure does not have a constructor. | Unlike class, union does not have a constructor. | Unlike class, enumeration does not have a constructor. |
7 | Structure is often regarded as a data organizational tool in C. | Union is often regarded as memory efficient way of organizing data in C. | Enumeration is known as constant collecting data structure in C. |
8 | Structure does not allow to define a function inside it. | We are not allowed to declare function inside Union in C. | We are not allowed to declare functions inside Enumeration in C. |
With this article at OpenGenus, you must have the complete idea of struct vs union vs enum in C.