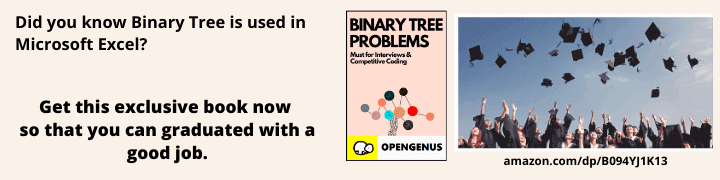
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
In this article, we have developed a Timer and Stopwatch console application in C Programming Language. This is a good project for your portfolio.
What is a console application ?
A console application is a type of computer program that runs in a command-line interface (CLI) environment, rather than a graphical user interface (GUI) environment. Console applications typically interact with the user through text-based input and output, rather than through graphical elements such as buttons and windows.
In a console application, the user interacts with the program by typing commands and receiving text-based output in return. The program can also display text-based menus and prompts to guide the user through the different options and features. The input and output are performed through standard input/output channels, such as the keyboard and the screen.
What are the features of this application ?
The Timer and Stopwatch application is a simple console based application that allows users to perform time-related tasks.
The application includes two main features: a Timer and a Stopwatch. The Timer feature allows the user to set a specific amount of time and counts down from that amount to zero. The Stopwatch feature allows the user to measure the amount of time that has passed since the start of the stopwatch.
The Timer feature also includes a progress bar that shows the percentage of time that has passed and updates in real-time. The Stopwatch feature includes a progress bar that shows the percentage of time that has passed and updates in real-time.
Additionally, the application includes options for the user to pause, continue, reset and quit the timer and stopwatch. The user can press 'p' to pause the timer or stopwatch, 'c' to continue the timer or stopwatch, 'r' to reset the timer or stopwatch, and 'q' to quit the application.
The application provides a simple menu for choosing between the timer, stopwatch, and quitting the program. The user can select the option they want to use, enter the time they want to set for the timer or start the stopwatch, and then interact with the application using the pause, continue, reset, and quit options.
Here is how to create a timer and the stopwatch function in C:
#include <stdio.h>
#include <time.h>
void timer() {
int minutes;
printf("Enter the number of minutes for the timer: ");
scanf("%d", &minutes);
for (int i = minutes; i >= 0; i--) {
for (int j = 59; j >= 0; j--) {
printf("Time remaining: %d:%d\n", i, j);
sleep(1); // sleep for 1 second
}
}
printf("Time's up!\n");
}
void stopwatch() {
int seconds = 0;
printf("Stopwatch started. Press 'q' to stop.\n");
while (getchar() != 'q') {
printf("Time elapsed: %d:%d\n", seconds / 60, seconds % 60);
sleep(1); // sleep for 1 second
seconds++;
}
printf("Stopwatch stopped.\n");
}
int main() {
int choice;
printf("1. Timer\n2. Stopwatch\nEnter your choice: ");
scanf("%d", &choice);
if (choice == 1) {
timer();
} else if (choice == 2) {
stopwatch();
} else {
printf("Invalid choice.\n");
}
return 0;
}
Output :
This program starts by displaying a menu that allows the user to choose between a timer and a stopwatch. The timer function takes user input for the number of minutes the timer should run for, and then counts down from that amount of time. The stopwatch function starts counting up from 0 and continues until the user presses the 'q' key. Both functions use the sleep() function to pause the program for 1 second between each update of the timer/stopwatch.
The step-by-step working process of this application is as follows:
1)The program starts with the main function.
2)The user is prompted to choose between a timer and a stopwatch by entering "1" for timer or "2" for stopwatch.
3)If the user enters "1", the timer function is called.
4)The timer function prompts the user to enter the number of minutes for the timer.
5)The program uses a nested for loop to count down the minutes and seconds. The outer for loop counts down the minutes, and the inner for loop counts down the seconds.
6)Each time through the loop, the current time remaining is displayed in the format "Time remaining: minutes:seconds"
7)The sleep(1) function is called to pause the program for one second before the loop iterates again.
Once the countdown reaches 0, the message "Time's up!" is displayed.
8)If the user enters "2", the stopwatch function is called.
The stopwatch function starts counting up from 0 and continues until the user presses the 'q' key.
9)The program uses a while loop that continues until the user press 'q' key
Each time through the loop, the current time elapsed is displayed in the format "Time elapsed: minutes:seconds"
10)The sleep(1) function is called to pause the program for one second before the loop iterates again.
11)Once the user press 'q' key, the message "Stopwatch stopped." is displayed
12)If the user enters any other input, the message "Invalid choice." is displayed.
The program ends with the return 0 statement.
Overall, this application uses a combination of user input, loops, and the
sleep function to create a simple timer and stopwatch program that can be run from the console.
What is a progress bar ?
A progress bar in a Timer or Stopwatch console refers to a graphical display that shows the amount of time that has passed or the amount of time remaining until the timer or stopwatch reaches its end. It is typically represented as a bar that is gradually filled in as time passes, with the bar becoming completely filled when the timer or stopwatch reaches its end.
The progress bar can be used to provide a visual representation of the current time, making it easier to track the progress of the timer or stopwatch.
Here is how to include a progress bar in the timer feature of the stopwatch:
void timer() {
int minutes;
printf("Enter the number of minutes for the timer: ");
scanf("%d", &minutes);
int total_seconds = minutes * 60;
int progress = 0;
for (int i = total_seconds; i >= 0; i--) {
progress = (total_seconds - i) * 100 / total_seconds;
printf("\rTime remaining: %d:%02d [%3d%%]", i / 60, i % 60, progress);
fflush(stdout);
sleep(1);
}
printf("\nTime's up!\n");
}
How does it work ?
1)The timer function prompts the user for the number of minutes and calculates the total number of seconds.
2)A variable "progress" is initialized to zero.
3)The for loop starts counting down from total seconds.
4)Inside the for loop, the progress is calculated by subtracting the remaining seconds from the total seconds and multiplying by 100.
5)Then the printf statement displays the remaining time in minutes and seconds format and the progress in percentage format.
6)The fflush(stdout) function is used to force the output of the printf statement to be written immediately to the console.
7)The program uses the sleep function to pause the program for one second before the loop iterates again.
8)Once the countdown reaches 0, the message "Time's up!" is displayed.
9)The progress bar feature uses the \r escape character in the printf statement to move the cursor back to the beginning of the line, allowing the progress to be updated in-place on the same line, rather than creating a new line for each update. This way the progress bar will appear to be moving smoothly.
It is possible to customize the output of the progress bar by adjusting the number of characters in the brackets and the width of the percentage display.
How to integrate the progress bar into the timer and the stopwatch function and add features of pause,continue and reset ?
#include <stdio.h>
#include <time.h>
#include <unistd.h>
#include <termios.h>
#include <sys/select.h>
void timer(int seconds) {
int i;
for (i = 0; i < seconds; i++) {
printf("\rTime remaining: %d seconds [", seconds - i);
int j;
for (j = 0; j < (i * 20 / seconds); j++) {
printf("-");
}
printf(">");
for (j = (i * 20 / seconds); j < 20; j++) {
printf(" ");
}
printf("] %d%%", (i * 100) / seconds);
fflush(stdout);
sleep(1);
}
}
int kbhit() {
struct timeval tv = { 0L, 0L };
fd_set fds;
FD_ZERO(&fds);
FD_SET(0, &fds);
return select(1, &fds, NULL, NULL, &tv);
}
void stopwatch() {
int i = 0;
char choice;
printf("Stopwatch Instructions:\n 'p' to pause the stopwatch,\n 'c' to continue, \n 'r' to reset the stopwatch,\n 'q' to quit the stopwatch\n");
while (1) {
printf("\rTime elapsed: %d seconds", i);
fflush(stdout);
sleep(1);
i++;
if (kbhit()) {
choice = getchar();
if (choice == 'p') {
printf("\nStopwatch paused. Press 'c' to continue, 'r' to reset or 'q' to quit\n");
while (1) {
choice = getchar();
if (choice == 'c') {
break;
} else if (choice == 'r') {
i = 0;
break;
} else if (choice == 'q') {
return;
}
}
}
}
}
}
int main() {
char choice;
printf("Welcome to the Timer and Stopwatch application!\n");
while (1) {
printf("\nPlease choose an option:\n");
printf("1. Timer\n2. Stopwatch\n3. Quit\n");
scanf(" %c", &choice);
switch (choice) {
case '1': {
int seconds;
printf("Enter the number of seconds for the timer: ");
scanf("%d", &seconds);
timer(seconds);
break;
}
case '2': {
stopwatch();
break;
}
case '3':
return 0;
default:
printf("Invalid choice. Please try again.\n");
break;
}
}
}
Output(1) - Timer :
Output(2) - Stopwatch:
The progress bar feature is added to both the timer and the stopwatch functions. The timer function calculates the total number of seconds based on the user input, and the progress is calculated based on the remaining time. In the stopwatch function, the progress is calculated based on the elapsed time. In both cases, the progress is displayed as a percentage in the same line as the time.
The \r escape character is used in the printf statement to move the cursor back to the beginning of the line, allowing the progress to be updated in-place on the same line, rather than creating a new line for each update. This way the progress bar will appear to be moving smoothly.
What does the sleep() function do ?
The sleep() function is a C library function that causes the program to pause for a specified number of seconds. It is typically used in situations where a program needs to wait for a certain amount of time before performing the next action.
The sleep function is defined in the unistd.h header file, which is why the line #include <unistd.h> is included at the top of the program.
The sleep function takes a single argument, which is the number of seconds the program should pause for. The argument is passed as an integer value. In the example program, the sleep function is called with the argument 1, which causes the program to pause for 1 second before continuing.
During the time that the program is paused, the CPU is not executing any instructions from the program. This allows other processes to use the CPU and prevents the program from using up too much resources.
Once the specified number of seconds has elapsed, the program resumes execution from the point where it was paused.
The sleep function is not always accurate and does not guarantee that the program will pause for exactly the specified number of seconds. The actual duration of the pause may be longer or shorter than requested due to factors such as system load and scheduling.
What is the role unistd.h ?
unistd.h is a standard C library header file that defines a number of functions and macros that are related to the POSIX (Portable Operating System Interface) operating system standard. The POSIX standard defines a set of APIs that are intended to be portable across different operating systems.
The unistd.h header file provides a number of functions that are related to the POSIX operating system standard, such as:
sleep() function which causes the program to pause for a specified number of seconds.
getpid() function which returns the process ID of the current process.
getppid() function which returns the process ID of the parent process.
fork() function which creates a new process.
exec() family of functions which replace the current process with a new process.
The header file also defines a number of macros, such as NULL, _POSIX_VERSION, _POSIX_C_SOURCE, and _POSIX_SAVED_IDS.
These macros provide information about the POSIX implementation on the system where the program is being compiled or executed.
Not all functions and macros from unistd.h are available on all systems, it depends on the implementation of the POSIX standard in the system. Some functions and macros might not be present or might have different behavior on non-POSIX systems.
In this program, the sleep() function is used from the unistd.h to pause the execution for a second which is necessary for the Stopwatch and Timer to work.
What is POSIX?
POSIX (Portable Operating System Interface) is a set of standards for operating systems that are intended to be portable across different platforms. These standards are defined by the IEEE (Institute of Electrical and Electronics Engineers) and provide a common interface for application programmers to interact with the underlying operating system.
The POSIX standards define a set of system calls, libraries, and shell commands that are intended to be common across different operating systems, such as UNIX and Linux. This allows application programmers to write code that can be easily ported to different platforms without having to make major changes to the code. The POSIX standard is based on the UNIX operating system, and it's intended to provide a high degree of compatibility and uniformity among different UNIX-based systems.
With the help of this article at OpenGenus, you will have an idea on what Timer and Stopwatch console application in C is, what it does, and how to create and code it. This article will take you step by step through the process and help you create your own Timer and stopwatch application.