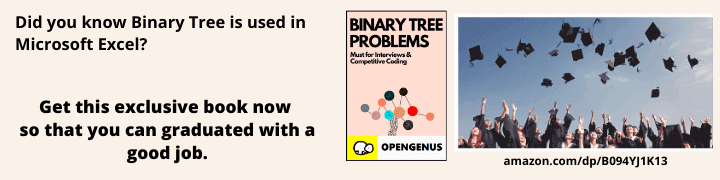
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
In this article, we will learn everything about the variables in C++ including rules of variable naming, types of variables and much more.
TABLE OF CONTENTS:
1.What are Variables?
2.Declaration of Variables
--- * With Initialisation
--- * Without initialisation
---* Declaring Multiple Variables
-----* Without Initialisation
-----* With Initialisation
3.Rules for Variable Name
4.Difference between variables and constants
5.Types of Variable(Based on Scope of Variables in c++)
---- * Static vs Instance Variable
6.Data Type of Variables
7.Print Variables
8.Arithmetic Operations on Variables
9.Exercise - Calculator!
What are Variables?
Variables as the name suggests are entities whose values are varying as opposed to constants whose value is fixed throughout the program.
They are like containers that holds a value.Variable names are the names given to memory location.When values in the memory for the variable changes ,the variable value also changes.
We have to declare all variables before using it.
Declaration of Variables
The variables can be declared by:
Without Initialisation
Datatype Variable_name;
Example:
int var1;
char name;
With Initialisation
Dtatype Variable_name=value;
Example:
int num=1;
char word='C',
--Donot forget the semicolon!!
Declaring Multiple Variables
Without Initialisation
We can declare multiple variables of same datatype by separating them using commas.
Datatype variable1,variable2,variable3;
Example:
int A,B,C,D;
With Initialisation
Here we have to assign values to individual variables.
int A=10,B=20,C=40;
Rules for Variable Name
-
A variable can have alphabets, digits and underscore.
-
A variable name can start with alphabet and underscore only. It can't start with digit.
-
No white space is allowed within variable name.
-
A variable name must not be any reserved word or keyword e.g. char, float etc.
Valid Variable Names
int c;
int _mjb;
int a980;
Invalid Variable Names
int 100;
int my name;
int float;
Difference between variables and constants
After declaration the value of variable can be changed anywhere in the program.We just have to assign the value to the variable .From that point whenever the variable is used the new value is taken.
#include <iostream>
using namespace std;
int main()
{
int a =10;
cout << a << endl;
a=20;
cout << a << endl;
return 0;
}
Output
10
20
whereas for a constant if we try to reassign value it will show error.
#include <iostream>
using namespace std;
int main()
{
const int a=10;
cout<< a << endl;
a=20;
cout<< a << endl;
return 0;
}
Output
main.cpp:17:6: error: assignment of read-only variable ‘a’
17 | a=20;
| ~^~~
Types of Variable(Based on Scope of Variables in C++)
There are 3 Types of variables in C++:
- Local variables
- Instance variables
- Static variables
Local Variables
- These are variables which are defined inside a function(method),block or constructor.
- Scope - Inside the block only
- Created when the method is called and destroyed when it exits the method
- Initialisation is mandatory
Instance Variables
- non-static variables that are declared in a class outside any method, constructor or block.
- created when an object of the class is created and destroyed when the object is destroyed.
- Can use access specifiers for instance variables. If we do not specify any access specifier then the default access specifier will be used.
- Initialisation of Instance Variable is not Mandatory.
- Instance Variable can be accessed only by creating objects.
Static Variables(Class Variables)
- Declared similarly as instance variables, the difference is that static variables are declared using the static keyword within a class outside any method constructor or block.
Example:
static int count=0;
- Unlike instance variables, we can only have one copy of a static variable per class irrespective of how many objects we create.
- created at the start of program execution and destroyed automatically when execution ends.
- Initialization is not Mandatory.
- Its default value is 0
- If we access the static variable through an object (like Instance variable ), the compiler will show the warning message and it won’t halt the program. The compiler will replace the object name to class name automatically.
- If we try to access the static variable without the class name, Compiler will automatically append the class name.
Static VS Instance Variable
static | Instance |
---|---|
only have one copy of a static variable per class (irrespective of how many objects we create ) | Each object will have its own copy |
changes made will be reflected in other objects (as static variables are common to all object of a class.) | Changes made in an instance variable using one object will not be reflected in other objects( as each object has its own copy of instance variable.) |
accessed directly using class name. | accessed through object references |
Data Type of Variables
While declaring a variable we have to define it's datatype.The various datatypes available are:
Data Type | size | Description |
---|---|---|
int | 4 bytes | Stores whole numbers, without decimals |
float | 4 bytes | Stores fractional numbers, containing one or more decimals. |
double | 8 bytes | Stores fractional numbers, containing one or more decimals |
boolean | 1 byte | Stores true or false values |
char | 1 byte | Stores a single character/letter/number, or ASCII values |
void | Represents the absence of type. |
Print Variables
We use cout to print/display variables
To combine the string and the variable we enclose the variable in << <<
Example:
int num=2;
cout<< "I have"" << num << "apples in my hand" ;
Arithmetic Operations on Variables
we can do arithmetic operations on variables of type float ,int,double etc.
1. Addition
Two variables of same datatype can de added and stored to a variable of same datatype.
#include <iostream>
using namespace std;
int main() {
int first_number, second_number, sum;
cout << "Enter two integers: ";
cin >> first_number >> second_number;
sum = first_number + second_number;
cout << first_number << " + " << second_number << " = " << sum;
return 0;
}
Output
Enter two integers: 4
5
4 + 5 = 9
2. Subtraction
Two variables of same datatype can de subtracted and stored to a variable of same datatype.
#include <iostream>
using namespace std;
int main() {
int first_number, second_number, diff;
cout << "Enter two integers: ";
cin >> first_number >> second_number;
diff = first_number - second_number;
cout << first_number << " - " << second_number << " = " << diff;
return 0;
}
Output
Enter two integers: 5
4
5 - 4 = 1
3. Division
Two variables of same datatype can de divided.
#include <iostream>
using namespace std;
int main() {
int first_number, second_number, div;
cout << "Enter two integers: ";
cin >> first_number >> second_number;
div = first_number / second_number;
cout << first_number << " / " << second_number << " = " << div;
return 0;
}
Output
Enter two integers: 6
2
6 / 2 = 3
4. Multiplication
Two variables of same datatype can de multiplied and stored to a variable of same datatype.
#include <iostream>
using namespace std;
int main() {
int first_number, second_number, mul;
cout << "Enter two integers: ";
cin >> first_number >> second_number;
mul = first_number * second_number;
cout << first_number << " * " << second_number << " = " << mul;
return 0;
}
Output
Enter two integers: 5
4
5 * 4 = 20
Exercise
Calculator
Now as we have learned about the basics of variables,how to define and use it.Let's do a small exercise - let's make a calculator.
1)Take input numbers from the user
2)Store it in 2 float variables(You can also use integer if you want to do integer operations only)
3)Take the operation to be done as a character input
4)Store it in another character variable
5)Define conditions to do operations according to the input operation symbol
The code for the same is given below:
# include <iostream>
using namespace std;
int main() {
char op;
float num1, num2;
cout << "Enter operator: +, -, *, /: ";
cin >> op;
cout << "Enter two operands: ";
cin >> num1 >> num2;
switch(op) {
case '+':
cout << num1 << " + " << num2 << " = " << num1 + num2;
break;
case '-':
cout << num1 << " - " << num2 << " = " << num1 - num2;
break;
case '*':
cout << num1 << " * " << num2 << " = " << num1 * num2;
break;
case '/':
cout << num1 << " / " << num2 << " = " << num1 / num2;
break;
default:
// If the operator is other than +, -, * or /, error message is shown
cout << "Error! operator is not correct";
break;
}
return 0;
}
Output:
Enter operator: +, -, *, /: -
Enter two operands: 3.4 8.4
3.4 - 8.4 = -5
Yay and that's it.We have learnt about variables and have executed a program using variables to perform the operations of a calculator.
With this article at OpenGenus, you must have the complete idea of variables in C++.