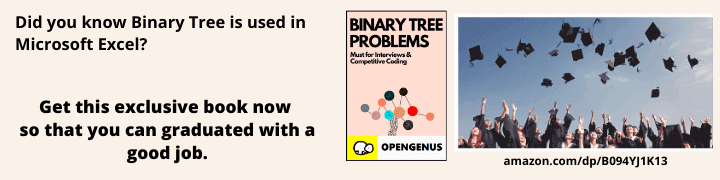
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
In this article, we will be discussing what scalar variable types are and how to use them correctly in Python. These are some basic and very important datatypes.
What you will learn from this article:
- What is a scalar variable
- The main scalar variables
- How to use the scalar variables
What is a Scalar Variable?
A scalar variable is a variable that contains a singular piece of data/information at a time. These variables are mostly immutable meaning you can not change the object once it has been created. A non-scalar variable like a list or array would connect one variable to many pieces of data/information and these types are mutable meaning we can change the data inside.
The Main Scalar Variable Types:
Python supports type inferencing. This means you do not have to declare variable types. It is still important, however, to know and understand the different of scalar variables.In Python there are 6 main scalar variable types:
- Integer
- Float
- String
- Boolean
- Complex
- None
You can declare these variables by assigning values to them as depicted in the code below.
num = 4
decimal_num = 4.0
str = "Hello"
true_or_false = True
true_or_false = False
no = None
How to use Scalar Variables:
1. Integer
Integers in Python don't vary too much from an integer in general. The integer is a whole number that can be zero, negative, or positive. In Python the length of an integer can is only restricted to the size of your computers memory. Unlike other languages in Python. The built-in method for converting an integer is int() and is used to convert other variables to integers. The following code will print the integer form of num_decimals which is 1.
num_decimals = 1.123456789
num_no_decimals = int(num_decimals)
print(num)
2. Float
A float is a short form for floating point variable and is exactly what it sounds like, which is a number with a decimal. The key difference between floats and integers is that decimal, integers can not have a decimal. If you do calculations between the two variables, the answer will be a float value. If you want to convert a variable to a float use the built-in float() function. A good to know function for floats is the round() function, you can round any float to the amount you want. The form of the function is round(float, i) where i is the amnount of decimals. The following code will round pi to two decimals.
pi = 3.14159265359
round(pi, 2)
print(pi)
3. String
A string represents a collective of characters inside either single or double quotes. Strings are immutable. As a result, in order to edit a string you need to use what are referred to as methods. These methods do not modify the strings but create a copy of the string, modify it and then return it. There are many valuable methods for editing strings, like capitalize() which you guessed it will capitalize the first letter of the string. The code below will print Hello.
str = "hello"
capitalized = str.capitalize()
print(capitalized)
4. Boolean
A boolean variable can carry one of two values: True or False. The first letter of True and False must be capitalized, otherwise you will get an error. Some common ways to use boolean variables is with arithmetic true and false logic. For example, you can ask if two variables are equal to each other. Another common use is to use the bool() function to check if a variable is True or False. Any non-0 value is represented as True. Below, you will see declaring a bool variable and the print functions will print False and True. Even though f is False it returns true because the two values are equivalent.
t = True
f = False
print(t == f)
print(f == f)
5. Complex
The complex variable can be taken quite literally if you are not strong in math as it involves algebra. A complex variable has two parts: real and imaginary. These numbers are represented in A + Bj where A and B are numbers. You can call on this variable with the complex(a,b) function or just initiating a variable with the complex form, z = a + bj any letter other than j will produce an error. The code below shows an example of how to set up a complex variable. both will produce 4 + 3j when printed.
z = 4 + 3j
z2 = complex(4,3)
print(z)
print(z2)
6. None
The None type is a special Python type in which there is only one possible value and that is None. Capitalization of None is important and has to be done or an error will occur. Why use a variable if all it does is hold the value None? The answer is that is that this variable is most commonly used in functions when there is no return statement or no following expression after a return statement. For example the print() function will always return None. If you ever want to find out the type of the function, use the type() function. the code below will show you how to declare a variable as None and shows how to use the type() function. The first print statement will print None and the second will print <class 'NoneType'>.
z = None
print(z)
print(type(print()))
Question
What will the following code output:
print(bool(0.0),(9.821983),(-39),(0),("HELLO"))
Key Takeaways
- Scalar Variables contain a singular piece of data/information at a time.
- Immutable objects are objects that do not allow for any change once the object is created.
- There are 6 main scalar variables: Integers, Floats, Strings, Booleans, Complex, and None.
- Python supports type inferencing meaning so you are not required to declare variable types on declaration. Knowing the types is very helpful.
- When converting variables you can use the type followed by brackets, ie int() or str().
- Any non-zero value is considered True.
- When you want to find out the type a variable is holding the type() function will return it.
With this article at OpenGenus, you must have the complete idea of Scalar Variable Types in Python.