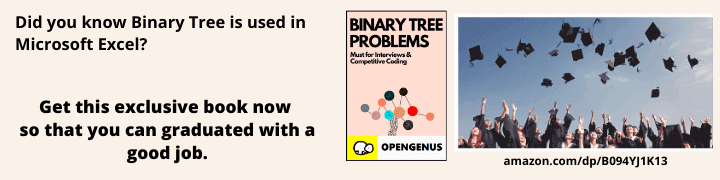
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
There are various ways of converting string to double in the C++ language; but before going into the ways, let me give you a quick intro to string and double.
C++ provides the programmers a wide variety of built-in as well as user defined data types. String and double are both data types but they differ in a few respects:
- Double is a built-in/primitive data type while std::string is a part of the standard library that comes with the C++ compiler which can be used after including the string.h header file.
- String is a sequence of 0 or more characters while a variable of type double can hold floating point numbers upto 8 bytes.
Now we shall look into the various ways we can convert double to string in C++. The diferent ways are listed below:
- double to String using C++’s std::to_string
- double to String using ostringstream
- double to String/character array using sprintf
- double to String boost’s lexical_cast
1. double to String using C++’s std::to_string
A double can be converted into a string in C++ using std::to_string. The required parameter is a double value which returns a string object that contains the double value as a sequence of characters.
Example:
#include <iostream>
#include <string>
using namespace std;
int main()
{
double d1 = 23.43;
double d2 = 1e-9;
double d3 = 1e40;
double d4 = 1e-40;
double d5 = 123456789;
string d_str1 = to_string(d1);
string d_str2 = to_string(d2);
string d_str3 = to_string(d3);
string d_str4 = to_string(d4);
string d_str5 = to_string(d5);
cout << "Number: " << d1 << '\n'
<< "to_string: " << d_str1 << "\n\n"
<< "Number: " << d2 << '\n'
<< "to_string: " << d_str2 << "\n\n"
<< "Number: " << d3 << '\n'
<< "to_string: " << d_str3 << "\n\n"
<< "Number: " << d4 << '\n'
<< "to_string: " << d_str4 << "\n\n"
<< "Number: " << d5 << '\n'
<< "to_string: " << d_str5 << '\n';
return 0;
}
In the above code, we declared 5 variables of double type, ranging from a very small to a very large number, to see the various kinds of outputs. These double values are converted to string objects using to_string.
Output of the above code is as follows:
Number: 23.43
to_string: 23.430000
Number: 1e-09
to_string: 0.000000
Number: 1e+40
to_string: 10000000000000000303786028427003666890752.000000
Number: 1e-40
to_string: 0.000000
Number: 1.23457e+08
tostring: 123456789.000000
Note:It has a default precision of six digits which cannot be changed.
2. double to String using ostringstream
A double can also be converted into a string in C++ using ostringstream in different ways according to our requirements. The following code can be used when we want large numbers to be converted to scientific notation.
Example:
#include <iostream>
#include <string>
#include <sstream>
using namespace std;
int main()
{
ostringstream ss1,ss2,ss3,ss4;
double d1 = 23.43;
double d2 = 6789898989.339994;
double d3 = 1e40;
double d4 = 1e-40;
ss1<<d1;
ss2<<d2;
ss3<<d3;
ss4<<d4;
string d_str1 = ss1.str();
string d_str2 = ss2.str();
string d_str3 = ss3.str();
string d_str4 = ss4.str();
cout << "Number: " << d1 << '\n'
<< "to string: " << d_str1 << "\n\n"
<< "Number: " << d2 << '\n'
<< "to string: " << d_str2 << "\n\n"
<< "Number: " << d3 << '\n'
<< "to string: " << d_str3 << "\n\n"
<< "Number: " << d4 << '\n'
<< "to string: " << d_str4 << "\n\n";
return 0;
}
In the above code, we declared 4 variables of double type ranging from a very small to a very large number to see the various kinds of outputs. These double values are converted to string objects using osstringstream.
Output of the above code is as follows:
Number: 23.43
to string: 23.43
Number: 6.7899ee+09
to string: 6.7899ee+09
Number: 1e+40
to string: 1e+40
Number: 1e-40
to string: 1e-40
Note: Including header file sstream is a must. For large numbers, ostringstream automatically converts it to scientific notation.
We can perform the following code to convert double to string with osstringstream to get the output as fixed-point notation inplace of scientific notation.
Example:
#include <iostream>
#include <string>
#include <sstream>
using namespace std;
int main()
{
ostringstream ss1,ss2,ss3,ss4;
double d1 = 23.43;
double d2 = 6789898989.339994;
double d3 = 1e40;
double d4 = 1e-40;
ss1<<fixed<<d1;
ss2<<fixed<<d2;
ss3<<fixed<<d3;
ss4<<fixed<<d4;
string d_str1 = ss1.str();
string d_str2 = ss2.str();
string d_str3 = ss3.str();
string d_str4 = ss4.str();
cout << "Number: " << d1 << '\n'
<< "to string: " << d_str1 << "\n\n"
<< "Number: " << d2 << '\n'
<< "to string: " << d_str2 << "\n\n"
<< "Number: " << d3 << '\n'
<< "to string: " << d_str3 << "\n\n"
<< "Number: " << d4 << '\n'
<< "to string: " << d_str4 << "\n\n";
return 0;
}
Output of the above code is as follows:
Number: 23.43
to string: 23.430000
Number: 6.7899ee+09
to string: 6789898989.339994
Number: 1e+40
to string: 10000000000000000303786028427003666890752.000000
Number: 1e-40
to string: 0.000000
Note:It has a default precision of six digits.
We can convert double to string with custom precision by setting the precision in stringstream as below.
Example:
#include <iostream>
#include <string>
#include <sstream>
using namespace std;
int main()
{
ostringstream ss1,ss2,ss3,ss4;
ss1.precision(2);
ss2.precision(2);
ss3.precision(2);
ss4.precision(2);
double d1 = 23.43;
double d2 = 6789898989.339994;
double d3 = 1e40;
double d4 = 1e-40;
ss1<<fixed<<d1;
ss2<<fixed<<d2;
ss3<<fixed<<d3;
ss4<<fixed<<d4;
string d_str1 = ss1.str();
string d_str2 = ss2.str();
string d_str3 = ss3.str();
string d_str4 = ss4.str();
cout << "Number: " << d1 << '\n'
<< "to string: " << d_str1 << "\n\n"
<< "Number: " << d2 << '\n'
<< "to string: " << d_str2 << "\n\n"
<< "Number: " << d3 << '\n'
<< "to string: " << d_str3 << "\n\n"
<< "Number: " << d4 << '\n'
<< "to string: " << d_str4 << "\n\n";
return 0;
}
Output of the above code is as follows:
Number: 23.43
to string: 23.43
Number: 6.7899ee+09
to string: 6789898989.34
Number: 1e+40
to string: 10000000000000000303786028427003666890752.00
Number: 1e-40
to string: 0.00
3. double to String/character array using sprintf
We can convert double to string or a character array with custom precision by specifying the precision in sprintf as below. Using sprintf we can add extra text (as per required) to the string at the same time.
Example:
#include <iostream>
#include <string>
#include <cstring>
using namespace std;
int main()
{
double d1 = 23.43;
double d2 = 6789898989.339994;
double d3 = 1e40;
double d4 = 1e-40;
char s[200];
sprintf(s," 23.43 converts to %.2f \n 6789898989.339994 converts to %.3f \n 1e40 converts to %.4f \n 1e-40 converts to %.5f",d1,d2,d3,d4);
cout<<s<<"\nsize of the above string is:"<<strlen(s);
return 0;
}
Output of the above code is as follows:
23.43 converts to 23.43
6789898989.339994 converts to 6789898989.340
1e40 converts to 10000000000000000303786028427003666890752.0000
1e-40 converts to 0.00000
size of the above string is:165
Note: Do not forget to include the cstring header while using the strlen function to find the length.
4. double to String boost’s lexical_cast
One of the best ways to convert double to string is using the lexical cast.
Example:
#include <iostream>
#include <string>
#include <boost/lexical_cast.hpp>
using namespace std;
int main()
{
double d1 = 23.43;
double d2 = 1e-9;
double d3 = 1e40;
double d4 = 1e-40;
double d5 = 123456789;
string d_str1 = boost::lexical_cast<string>(d1);
string d_str2 = boost::lexical_cast<string>(d2);
string d_str3 = boost::lexical_cast<string>(d3);
string d_str4 = boost::lexical_cast<string>(d4);
string d_str5 = boost::lexical_cast<string>(d5);
cout << "Number: " << d1 << '\n'
<< "to string: " << d_str1 << "\n\n"
<< "Number: " << d2 << '\n'
<< "to string: " << d_str2 << "\n\n"
<< "Number: " << d3 << '\n'
<< "to string: " << d_str3 << "\n\n"
<< "Number: " << d4 << '\n'
<< "to string: " << d_str4 << "\n\n"
<< "Number: " << d5 << '\n'
<< "to string: " << d_str5 << '\n';
return 0;
}
The output of the above code is as follows:
Number: 23.43
to string: 23.43
Number: 1e-09
to string: 1.0000000000000001e-09
Number: 1e+40
to string: 1e+40
Number: 1e-40
to string: 9.9999999999999993e-41
Number: 1.23457e+08
to string: 123456789
Note: Including header file boost/lexical_cast is a must.
With this article at OpenGenus, you must have the complete idea of different ways to convert double to string in C++. Enjoy.