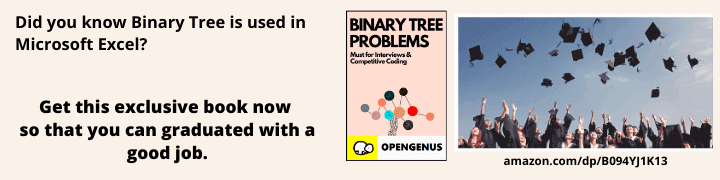
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
Reading time: 20 minutes | Coding time: 5 minutes
Vector is a sequential container class which is one of the four component of STL(Standard Template Library) in C++. Vector is used to store objects and data .
We have covered front() function of vector container in C++ STL in depth along with differences between front(), begin(), back() and end() Functions.
In arrays where we dedicate fixed amount of memory while declaring it but in vectors there is no such requirement and vector can resize itself accordingly while inserting and deleting element.Vector is similar to Dynamic arrays.
front()
function is used to access the first element of vector container.
- This returns the reference to the first element of the vector. Reference is indirect access to a particular element or data unlike Iterators.
- There is no parameter passed.
SYNTAX
vectorname.front()
COMPLEXITY
- time complexity:
O(1)
EXAMPLE
#include <iostream>
#include <vector>
using namespace std;
int main()
{
vector<int> vector1;
vector1.push_back(1);
vector1.push_back(3);
vector1.push_back(9);
vector1.push_back(18);
vector1.push_back(2);
// Vector1 becomes 1, 3, 9, 18, 2
cout << vector1.front();
return 0;
}
Output:
1
Explanation of code:
In this code , first the vector is initialise and using the modifier push_back ( It pushes the elements into a vector from the back). After pushing vector if we want to access first element directly of a vector than we can call front() function which in this code returns the first element of vector i.e 1.
Difference between front(), begin(), back() and end() Functions
These functions are often confused to provide the same end result but the difference between them is that the front() and back () functions are used to provide the refernce to the first and last element of the vector .
On the other hand , begin() function returns an iterator pointing to the first element of the vector and end() functions returns an iterator pointing to the last element of vector.
Iterators return the position of the element of the container. Precisely they are used to point at the memory address of element like pointers .
begin() and end() can be used to traverse through the elements of container but front() and back() returns only the refernce only of the first and last element
helping us to access those elements directly .
Example demonstrating front() , begin () , back() and end () Functions
#include <iostream>
#include <vector>
using namespace std;
int main()
{
vector<int> vector1;
for (int i = 1; i <= 5; i++)
vector1.push_back(i); // 1, 2, 3, 4, 5
cout << "Output of begin and end: ";
vector<int>::iterator i; // Declaring and iterator type
for (i = vector1.begin(); i != vector1.end(); ++i)
{
cout << *i << " ";
}
cout << "\nfront() : vector1.front() = " << vector1.front();
cout << "\nback() : vector1.back() = " << vector1.back();
}
Output:
Output of begin and end: 1 2 3 4 5
front() : vector1.front() = 1
back() : vector1.back() = 5
Explanation of code:
In the above written code we first declare a vector and then using the function push_back() we push the element one by one from back . To tarverse the vector using begin() and end() whose return type is an iterator we declare i .Now using the loop we tarverse through the vector and to print the value of an iterator at any memory address we use * (asterisk sign). Then by using front() and back() we print 1 and 5 as the first and last element of vector respectively.
Moving to other vector functions :
1. vector::empty()
empty function is used to to tell whether the container is empty or not .
It returns boolean value .
- Syntax
vectorname.empty()
- parameters are not passed.
Returns :
1, if vector is empty
0, Otherwise
EXAMPLE
#include <iostream>
#include <vector>
using namespace std;
int main()
{
vector<int> vector1;
if (vector1.empty()) {
cout << "True";
}
else {
cout << "False";
}
return 0;
}
Output:
True
Explanation of code:
Here in this code we create a vector with no vlaue . vector1.empty() here returns 1 since the vector is empty and thus we get the output as 1.
IMPLEMENTATION of empty() to count the number of elements in a vector
#include <iostream>
#include <vector>
using namespace std;
int main()
{
vector<int> vector1;
vector1.push_back(1);
vector1.push_back(3);
vector1.push_back(9);
vector1.push_back(18);
vector1.push_back(2);
// Vector1 becomes 1, 3, 9, 18, 2
int count=0;
while(!vector1.empty())
{
count++;
vector1.pop_back();
}
cout<<count; // computed size of vector
}
Output:
5
Explanation of code:
In the above code empty() function is used to traverse vector till it becomes empty
and within the loop we use pop_back() modifier to delete an element from back once it is counted . Therefore when all the elements are popped out we get the total number of elements which were present in our vector.
2. vector::operator[]
This is an operator used to return reference of the element directly by passing index of the desired element in []. When the position is not in the bounds of the size of vector,this operator causes undefined behaviour.
Syntax :
vectorname[position]
Parameters :
Position of the element to be fetched.
Returns :
Direct reference to the element at the given position.
Example
#include <iostream>
#include <vector>
using namespace std;
int main()
{
vector<int> vector1;
for (int i = 1; i <= 5; i++)
vector1.push_back(i); // 1, 2, 3, 4, 5
cout << "\nReference operator [g] : vector1[2] = " << vector1[2];
}
Output:
Reference operator [g] : vector1[2] = 3
Explanation of code:
In the above code , the reference operator directly returns the element present at index number [2] which is 3.
Question
What is the time complexity for removing elementy at the end of vector?
With this article at OpenGenus, you must have the complete idea of front() function in vector container in C++ STL. Enjoy.