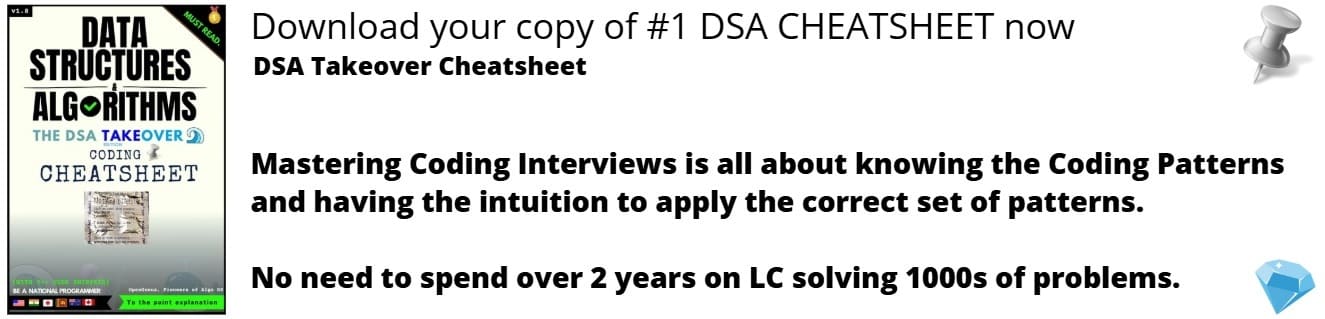
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
In this article, we will explore 5 different ways to delete elements in an array in JavaScript Programming Language.
What is an array?
First, let's talk about arrays. An array is a data structure that holds values in an ordered way. These values can be types of strings, numbers, booleans, objects, and other arrays and they are enclosed in square brackets. Each value or element has its own index. Indexes are numbers, which indicate the position of the elements, for example, since arrays start with a 0 index (the first element in the array), the second element will be at index 1, and so on.
const myArray = [1, 2, 'computer', 'program', true, {language: 'JavaScript', year: 1995}, ['apple', 'orange', 'pear']];
In the example above, we have a mix of elements from different types, the number 1 is at index 0, the number 2 is at index 1, the string 'computer' is at index 2, the string 'program' is at index 3, the boolean true is at index 4, the object is at index 5 and the array which holds the string fruits is at the last index, at 6 (or we can also say it's at index -1).
We can access the elements by using their indexes.
console.log(myArray[3]);
// 'program'
console.log(myArray[-2]);
// {language: 'JavaScript', year: 1995}
So now that we know what an array looks like, let's see how we can delete elements from it.
Deleting elements
The different ways to delete elements in an array in JavaScript are:
- pop()
- shift()
- splice()
- filter()
- slice()
1. pop()
Pop is a method that removes the last element of an array and also returns it.
const groceries = ['milk', 'bread', 'eggs', 'cheese', 'ham']
const removedElement = groceries.pop();
console.log(removedElement);
// 'ham'
console.log(groceries);
// ['milk', 'bread', 'eggs', 'cheese']
If we use the pop() method with an empty array, then we get back undefined.
const emptyArray = [];
const removed = emptyArray.pop();
console.log(removed);
// undefined
2. shift()
The shift() is very similar to the pop() method, but instead of removing the last element, it removes the first element of the array.
const removedFirst = groceries.shift();
console.log(removedFirst);
// 'milk'
console.log(groceries);
// ['bread', 'eggs', 'cheese']
Also, it's the same thing as with the pop() method, when the array is empty and we use the shift() method, we get back undefined.
3. splice()
With the splice() method, we can do more things, add, replace, and delete elements.
We need to pass in at least one argument inside the parentheses in order for the method to work.
To delete all the elements in the array, we need to pass in the number 0 as an argument. This means that we want to start the deleting process from the first element, which is at index 0. Since we don't have any additional arguments inside the parentheses, the deleting process will go all the way to the end until all the elements are removed.
Remove all the elements from the array:
groceries.splice(0);
console.log(groceries);
// []
Now, we have no elements in our groceries array.
Remove all the elements starting from a specific index:
const pets = ['dog', 'cat', 'fish', 'bird'];
pets.splice(2);
console.log(pets);
// ['dog', 'cat']
Here, we started the deleting process from index 2, so fish and bird is deleted.
Remove a given amount of elements from a specific index:
const favoriteNumbers = [91, 30, 10, 3, 1];
favoriteNumbers.splice(1, 2);
console.log(favoriteNumbers);
// [91, 3, 1]
We have passed two arguments in the above example, the first argument is the start of the process, so we will start deleting from index 1 (number 30), and the second argument means how many elements we are going to delete, in this case, 2 elements. So we start from index 1 which will be deleted and we need to delete one more which will be the number 10 because that comes after the number 30.
If we pass in a number as the second argument which is bigger than the elements remaining after the starting point, then all the elements will be deleted after the starting point.
console.log(favoriteNumbers);
// [91, 3, 1]
favoriteNumbers.splice(0, 3);
console.log(favoriteNumbers);
// [91]
We can pass in additional arguments inside the parentheses but that's for when we want to insert an element.
4. filter()
The filter() is a really useful method, but we need to understand first how it's working.
const users = [{
name: 'Jane Doe',
age: 34,
email: 'janedoe@example.com'
},
{
name: 'Bob Wright',
age: 56,
email: 'bobby@example.com'
},
{
name: 'Wendy Brown',
age: 23,
email: 'wbrown@example.com'
},
{
name: 'John Doe',
age: 40,
email: 'johnsm@example.com'
},
{
name: 'Mary Ann',
age: 34,
email: 'maryann@example.com'
}]
So we have an array of objects and each object contains some information about a user.
Let's try out the filter method to delete all the users whose age is 34.
users.filter(user => user.age !== 34);
console.log(users);
/*
{
name: 'Jane Doe',
age: 34,
email: 'janedoe@example.com'
},
{
name: 'Bob Wright',
age: 56,
email: 'bobby@example.com'
},
{
name: 'Wendy Brown',
age: 23,
email: 'wbrown@example.com'
},
{
name: 'John Doe',
age: 40,
email: 'johnsm@example.com'
},
{
name: 'Mary Ann',
age: 34,
email: 'maryann@example.com'
}
*/
What happened here?
Well, nothing really happened here, because the filter() method doesn't change or mutate the original array.
We can assign the filter functionality to a variable.
const newArray = users.filter(user => user.age !== 34);
console.log(newArray);
/*
{
name: 'Bob Wright',
age: 56,
email: 'bobby@example.com'
},
{
name: 'Wendy Brown',
age: 23,
email: 'wbrown@example.com'
},
{
name: 'John Doe',
age: 40,
email: 'johnsm@example.com'
},
*/
Now, we have a separate, new array which is actually a copy of the original array and we returned the users whose age is not 34.
The original users array didn't change.
5. slice()
The slice() method also creates a copy of the original array, so we can store the new array in a variable.
We need to pass in at least one argument inside the parentheses. Keep in mind, if you pass the number 0, then the method will do nothing.
const nums = [1, 2, 3];
const newNums = nums.slice(0);
console.log(newNums);
// [1, 2, 3]
Remove a given amount of elements:
const alphabet = ['a', 'b', 'c', 'd', 'e', 'f', 'g', 'h', 'i', 'j', 'k', 'l', 'm', 'n', 'o', 'p', 'q', 'r', 's', 't', 'u', 'v', 'w', 'x', 'y', 'z'];
const newList1 = alphabet.slice(15);
console.log(newList1);
// ['p', 'q', 'r', 's', 't', 'u', 'v', 'w', 'x', 'y', 'z']
We removed the first 15 elements from the array and stored the rest in the newList1 array.
Another example:
const newList2 = newList1.slice(15);
console.log(newList2);
// []
In this example, all the letters were removed from the newList1 array because the number(15) we passed in as an argument it's bigger than the length of the array, so it will remove everything.
Remove from a specific index:
const animals = ['lion', 'horse', 'bear', 'zebra', 'whale'];
const newAnimalsArray = animals.slice(0, 3);
console.log(newAnimalsArray);
// ['lion', 'horse', 'bear']
Let's see the arguments first, the number 0 is the starting point and the number 3 is the endpoint index, which means that in the variable newAnimalsArray, a slice of the animals array will be stored, in this case, the slice is from lion to bear. So from index 3, the elements will be removed. Don't forget that the original array is unchanged.
Another example:
const vegetables = ['carrot', 'potato', 'tomato', 'cabbage', 'cucumber'];
const newVeggieList = vegetables.slice(2, 4);
console.log(newVeggieList);
// ['tomato', 'cabbage']
We sliced out a piece from the vegetables array and stored it in the newVeggieList array.
The slice started from index 2 (so indexes 0 and 1 are removed) and went until index 4, which is not included (so index 4 is removed as well). We got a slice from index 2-3 which is tomato and cabbage.
Summary
- Mutating: pop(), shift(), splice()
- Non-mutating: filter(), slice()
We have seen a couple of ways of deleting elements from an array, some of them are mutating (changing) the original array, so the usage of which methods to use depends on the way you need to work with these arrays.