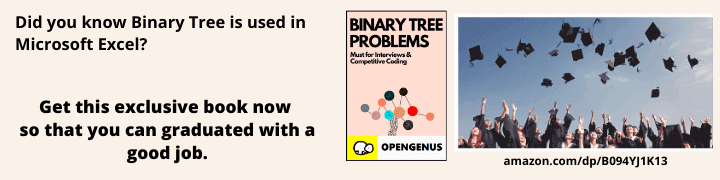
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
Reading time: 35 minutes | Coding time: 10 minutes
In this article, we will use the Geo API of Twitter API and use it to get details of a place and find out nearby places (co-ordinates) to it. This is interesting as one may think that Twitter is all about Tweets but classifying data across Geography is a major feature of Twitter.
We will review some basic information about Twitter API, its characteristics and get Twitter API keys. Following this, we will dive into Geo API and write a code in Python using the Requests library to achieve this.
Twitter API
- The Twitter API is simply a set of URLs that take parameters. They URLs let you access many features of Twitter, such as posting a tweet or finding tweets that contain a word, etc.
- Twitter allows you to interact with its data tweetsand several attributes about tweets using twitter API.
- Twitter API's can be accessed only via authenticated requests.
Characteristics of Twitter API
- The twitter API uses JSON data format for returning and receiving the data.
- The twitter API is HTTP-based (over SSL) API meaning we can use get method to retrieve data from twitter,post method to send requests to the twitter server and search method to search the twitter posts.
- The twitter API limits the number of requests that can be sent to the twitter server per access token or twitter account.This is called twitter rate limit.If you encounter twitter rate limit exceeded error it means that Twitter rejected consecutive attempts to access its API under your Twitter account.The rate limit is different for different methods of the API.
- The methods of twitter API accepts various parameters which are used to cusotmize the requests according to needs.
- There are twitter API libraries for almost all programming languages.
Read the Documentation of twitter API from here
Getting Twitter API keys
To start with, we will need to have a Twitter developer account and obtain credentials (i.e. API key, API secret, Access token and Access token secret) on the to access the Twitter API, following these steps:
- Create a Twitter developer account https://developer.twitter.com/
- Go to https://developer.twitter.com/en/apps and log in with your Twitter user account.
- Click “Create an app”
- Fill out the form, and click “Create”
- A pop up window will appear for reviewing Developer Terms. Click the “Create” button again.
- In the next page, click on “Keys and Access Tokens” tab, and copy your “API key” and “API secret” from the Consumer API keys section.
- Scroll down to Access token & access token secret section and click “Create”. Then copy your “Access token” and “Access token secret.
Geo API
- Geo API is a part of twitter API which is used to send Get queries to the twitter server.
- It searches the desired query in the twitter server and returns all the information about a known "place".
- It takes parameters in JSON format and returns the response data in JSON format.
- It takes only place_id as a parameter and returns all the information related to the place associated with the place_id.
(1) Get information about a place
This functionality returns all the information about a known "place". We need to specify the place id and the twitter server returns information in JSON format.
Resource URL for getting information about a place:
https://api.twitter.com/1.1/geo/id/:place_id.json
(2) Get places near a location
This allows us to search for places that can be attached to a twitter post by specify a latitude and a longitude pair, an IP address, or a name as parameter, this request will return a list of all the valid places that can be used as the place_id when updating a status.
Resource URL for getting nearby places:
https://api.twitter.com/1.1/geo/search.json
What is Requests package?
The requests module allows you to send HTTP requests using Python.
The HTTP request returns a Response Object with all the response data (content, encoding, status, etc).We will be using the post method of requests library to send a request to tweet.
Install requests package using command:
pip install requests
Get Requests
The get() method sends a GET request to the specified url.It is used to retrieve data from the server in JSON format.
Syntax
requests.get(url, params={key: value}, args)
Python Code And Explanation
After importing the dependencies, first we want to create variables(consumer_key and consumer_secret) that will authenticate with Twitter.You will find all the required variables in your developer's account dashboard and we can copy and paste each of them as strings.
#importing all dependencies
import numpy as np
import tweepy
import requests
import base64
#Define your keys from the developer portal
consumer_key = 'XXXXXXXXXXXXXXXXXXXXXX'
consumer_secret = 'XXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXX'
The twitter API requires a single key that is a string of a base64 encoded version of the two keys separated by a colon so we will encode the consumer keys into base64 which is the usable form.
#Reformat the keys and encode them
key_secret = '{}:{}'.format(consumer_key, consumer_secret_key).encode('ascii')
#Transform from bytes to bytes that can be printed
b64_encoded_key = base64.b64encode(key_secret)
#Transform from bytes back into Unicode
b64_encoded_key = b64_encoded_key.decode('ascii')
Now, We will use requests package of python to post an authentication request using twitter authentication resource URL to the twitter server and store the post response in a variable. We To check and make sure that the request worked , We will print the status code of the request response. If the status code printed is 200 then the request worked successfully.
base_url = 'https://api.twitter.com/'
auth_url = '{}oauth2/token'.format(base_url)
auth_headers = {
'Authorization': 'Basic {}'.format(b64_encoded_key),
'Content-Type': 'application/x-www-form-urlencoded;charset=UTF-8'
}
auth_data = {
'grant_type': 'client_credentials'
}
auth_resp = requests.post(auth_url, headers=auth_headers, data=auth_data)
print(auth_resp.status_code)
access_token = auth_resp.json()['access_token']
Now, we have to send a get request to the twitter server which will return the details of the place in JSON format according to the parameters specified. This request requires authentications so we will pass access token into the headers of this request. Now we will assign the parameters required for this request in JSON format. The parameter place_id specifies location of which we want the details.
Now, we will send a get request to the twitter server and store the response. These IDs can be retrieved from geo/reverse_geocode. We will print the status code of the request response. If the status code printed is 200 then the request worked successfully.
geo_headers = {
'Authorization': 'Bearer {}'.format(access_token)
}
geo_params = {
'place_id': 'df51dec6f4ee2b2c'
}
geo_url = 'https://api.twitter.com/1.1/geo/id/:place_id.json'
geo_resp = requests.get(geo_url, headers=geo_headers, params=geo_params)
Now , we will save the response in json format and print it. The response contains all the information about the location.
Now, we will send a get request to the twitter server to search and return upto 20 places to link it with our twitter posts. We can provide atitude and a longitude pair, an IP address, or a name as parameter. All of these parameters are optional.In our example we have provided query as Toronto
geo_data = geo_resp.json()
print(geo_data)
Go through this JSON Response to understand the details that are provided by Twitter API:
{
"id": "df51dec6f4ee2b2c",
"url": "https://api.twitter.com/1.1/geo/id/df51dec6f4ee2b2c.json",
"place_type": "neighborhood",
"name": "Presidio",
"full_name": "Presidio, San Francisco",
"country_code": "US",
"country": "United States",
"contained_within": [
{
"id": "5a110d312052166f",
"url": "https://api.twitter.com/1.1/geo/id/5a110d312052166f.json",
"place_type": "city",
"name": "San Francisco",
"full_name": "San Francisco, CA",
"country_code": "US",
"country": "United States",
"centroid": [
-122.4461400159226,
37.759828999999996
]
}
]
}
Nearby places using Geo API
We will, now, extend the above code to get the list of nearby places.
geosearch_headers = {
'Authorization': 'Bearer {}'.format(access_token)
}
geosearch_params = {
'query' : 'Toronto'
}
geosearch_url = 'https://api.twitter.com/1.1/geo/search.json'
geosearch_resp = requests.get(geo_url, headers=geo_headers, params=geo_params)
Now we will traverse through the json reponse to print the area we have gotten as the response
geosearch_data=geosearch_resp.json()
print(geosearch_data['result']['places'][0])
Go through this JSON response carefully to understand the data that is returned by Geo API of Twitter and you can parse it to get the information you need.
{
'attributes':{
},
'bounding_box':{
'coordinates':[
[
[
-96.647415,
44.566715
],
[
-96.630435,
44.566715
],
[
-96.630435,
44.578118
],
[
-96.647415,
44.578118
]
]
],
'type':'Polygon'
},
'contained_within':[
{
'attributes':{
},
'bounding_box':{
'coordinates':[
[
[
-104.057739,
42.479686
],
[
-96.436472,
42.479686
],
[
-96.436472,
45.945716
],
[
-104.057739,
45.945716
]
]
],
'type':'Polygon'
},
'country':'United States',
'country_code':'US',
'full_name':'South Dakota, US',
'id':'d06e595eb3733f42',
'name':'South Dakota',
'place_type':'admin',
'url':'https://api.twitter.com/1.1/geo/id/d06e595eb3733f42.json'
}
],
'country':'United States',
'country_code':'US',
'full_name':'Toronto, SD',
'id':'3e8542a1e9f82870',
'name':'Toronto',
'place_type':'city',
'url':'https://api.twitter.com/1.1/geo/id/3e8542a1e9f82870.json'
}
With this, you have the complete knowledge of using Geo API of Twitter. Enjoy.