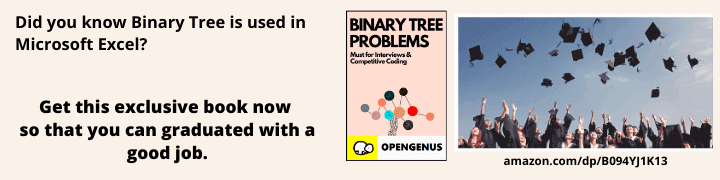
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
In some cases, it might happen that the method that you are calling goes wrong or generates an exception. An exception is an undesirable or unforeseen occasion, which happens during the execution of a program and disturbs the typical progression of the program's guidelines. Exception Handling is a way to deal with this possibility.
An exception is an object of type Exception. The process of creating such an object and handing it to the runtime system is known as "throwing" an exception. Exceptions can be classified into:
-
Checked exceptions: Exceptions that are checked by the compiler are called checked exceptions. The exceptions that are determined as Error,RuntimeExceptions and their sub-classes are excluded from this category. E.g. IOException, SQLException etc.
-
Unchecked exceptions: Exceptions that are a sub-class of RuntimeExceptions are called unchecked exceptions. They are not checked by the compiler at compile-time. E.g. ArithmeticException, NullPointerException, ArrayIndexOutOfBoundsException etc.
The part of code that handles exception is called an exception handler. The three main elements of an exception handler in Java are: try, catch and finally block.
Syntax
try
{
//code that might throw an exception
}
catch(ExceptionType ex)
{
//code that handles exception
}
finally
{
//code that gets executed either after try or try-catch
}
The try,catch and finally block
- The code that might generate an exception is written in the try block.The exception that arises in the try block is dealt by the exception handler linked to it.
- This task is done by the catch block. A catch block cannot be used without a try block paired with it and there can be more than one catch block linked to a try block.The exception thrown is caught by the catch block that matches its type.This checking is done till the exception is caught or doesn't find a catch statement that handles it.
Syntax
try
{
//try Block
}
catch(ExceptionType1 ex1)
{
//catch Block
}
catch(ExceptionType2 ex2)
{
//catch Block
}
catch(ExceptionType3 ex3)
{
//catch Block
}
-
The type of Exception or the argument of catch block decides if it can handle the exception.The Exception type must be a class inherited from the Throwable class.
-
In all versions after Java SE 6, more than one type of exception can be handled by a single catch block. This feature can cut down duplicate code.
Example
catch (IOException|SQLException ex) {
logger.log(ex);
throw ex;
}
- The catch block that handles more than one exception is implicitly final.In the above example, the catch parameter ex is final and therefore one cannot assign any values to it within the catch block.
- The finally block gets executed even if no exception occurs. It is an optional block used to put codes that always need to be executed.
- There can be only one finally block.
The try-with-resources statement
The try-with-resources statement was introduced in Java SE 7.A resource is an object that needs to be closed after its use e.g. BufferedReader. The try-with resource is declared with one or more resources that are automatically closed after the block is executed. The resource is closed even if the try statement terminates abruptly.
try(<declaration of resource 1 >;<declaration of resource 2>)
{
//body
}
NOTE: If there is a try block that throws the same exception as try-with-resources statement, then the exception thrown by the try-with-resources statement is suppressed,or we can say that the exception thrown by try block dominates the exception thrown by the try-with-resources statement.
The throw and throws keyword
The throw keyword is used to explicitly throw a pre-defined or custom exception.When an exception is thrown, the flow of program execution transfers from the try block to the catch block with the exception of same type. We use the throw keyword within a method and only single exception can be thrown using throw keyword.
Syntax:
throw ThrowableObject("Exception message");
public int div(int a,int b)
{
if(b==0)
throw new ArithmeticException("Cannot be divided by zero");
else
return a/b;
}
A throwable object is an instance or subclass of the Throwable class.The custom exception must extend Exception class.
The throws keyword is used declare one or more exceptions which may be thrown by a method or constructor.It is used for handling multiple checked exceptions,all at once i.e. multiple declaration is allowed.
The throws keyword does the same work as a try-catch block,it is just easier to use in case more than one method throw exception.
Syntax: public void example() throws SQLException , IOException
Questions
Consider this code:
import java.io.*;
public class example
{
public static void main(String[] args)
{
try
{ int n=6;
System.out.print("a");
int val = n / 0;
throw new IOException();
}
catch(EOFException e)
{
System.out.printf("b");
}
catch(ArithmeticException e)
{
System.out.printf("c");
}
catch(IOException e)
{
System.out.printf("d");
}
catch(Exception e)
{
System.out.printf("e");
}
}
}
What will be the output of the above code?
Consider this code:
import java.io.*;
public class Test
{
public static void main(String[] args)
{ for(int i=0;i<5;i++)
{
try
{
System.out.printf("1");
int data = 5 / i;
}
<br>catch(ArithmeticException e)
{
System.out.printf("2");
break;
}
finally
{
System.out.printf("3");
}
System.out.printf("4");
}
}
}
What will be the output of the above code?
Consider this code:
import java.io.*;
class prg {
public static void main(String args[]) {
try{
throw 1.5;
}
catch(int e) {
System.out.println("Got the Exception " + e);
}
}
}
What will be the output of the above code?
With this article at OpenGenus, you must have the complete idea of handling exceptions in Java using try catch finally block. Enjoy.