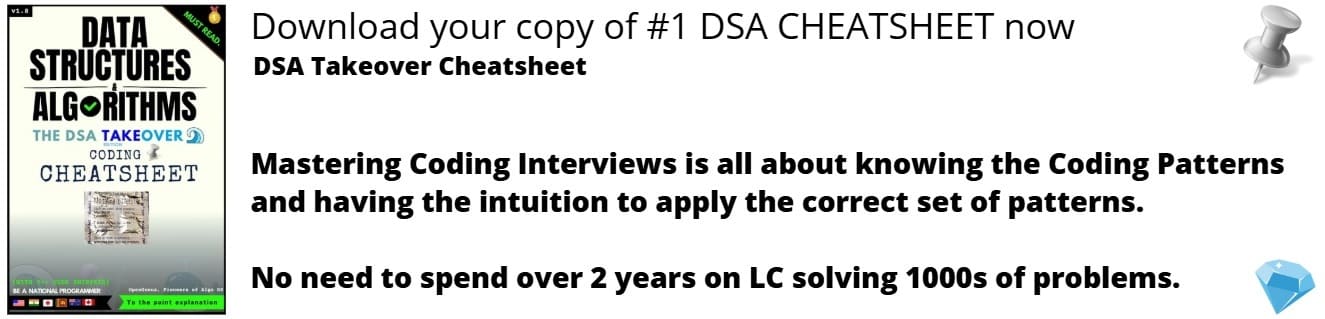
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
This article at OpenGenus explains the use of super keyword in Java. Also, we will discuss how it can be used for several purposes with various examples.
Super keyword is used to call the Constructor of the Parent Class. It is required when we need to pass some arguments to Parent Class and complete object instantiation. Recall that this keyword has two meanings: to denote a reference to the implicit parameter and to call another Constructor of the same class. Likewise, the super keyword has two meanings: to invoke a superclass method and to invoke a superclass Constructor.
Usage of Super Keyword
The use of super keyword in Java are:
- To refer to the variables of the Parent Class
- To call Overridden methods of the Parent Class
- To invoke Constructor of Parent Class
We will go through each of the above points in detail.
1. To refer to the variables of the Parent Class
This method is useful when both the Child Class and Parent Class have the some variables of the same name. Super is used to let the JVM(Java Virtual Machine) know which variable we are referring to.
Example:
class Vehicle {
int cost = 500000;
}
class Car extends Vehicle {
int cost = 300000;
void findCost() {
System.out.println(cost); // prints 300000
System.out.println(super.cost); // prints 500000
}
}
Vehicle is the Parent Class and Car is the Child Class. Here, we see that both these classes have a variable of same name cost. So, to access the cost variable of the Parent Class, we have to use super keyword.
2. To call Overridden methods of the Parent Class
If we want to call any method of the Parent Class which is not overriden, we can simply call it in the Child Class as it will be inherited. The use of super becomes necessary when we need to access any method of the Parent Class which has overriden in the Child Class.
Example:
class Vehicle {
int value = 500000;
int getCost() {
return value;
}
}
class Car extends Vehicle {
int cost = 300000;
int getCost() {
return cost;
}
void findCost() {
System.out.println(getCost()); // prints 300000
System.out.println(super.getCost()); // prints 500000
}
}
In the example above, we see that both the Vehicle Class and Car Class have a same method getCost(). This method has been overridden in the Car Class. So, to access the getCost() method of the Parent Class, we have used super keyword.
NOTE: When the Child Class doesn’t override the Parent Class method then we don’t need to use the super keyword to call the Parent Class method. This is because in this case we have only one version of each method and Child Class has access to the Parent Class methods so we can directly call the methods of Parent Class without using super.
3. To invoke Constructor of Parent Class
When we create an object of the Child Class, the Constructor of the Parent class is executed first and then the child class constructor is executed. It happens because compiler itself adds super() (Non-Parameterized Constructor of Parent Class) as the first statement in the Constructor of Child Class.
Example:
class Vehicle {
Vehicle() {
System.out.println("Vehicle Class Constructor");
}
}
class Car extends Vehicle {
Car() {
System.out.println("Car Class Constructor");
}
}
In the example above, it prints Vehicle Class Constructor first, and then Car Class Constructor.
Super keyword can be used to call either Parameterized or Non-Parameterized Constructors of the Parent Class.
Example:
class Vehicle {
Vehicle() {
System.out.println("Vehicle Class Constructor");
}
Vehicle(String name) {
System.out.println("Parameterized Vehicle Class Constructor");
}
}
class Car extends Vehicle {
Car() {
super("BMW");
System.out.println("Car Class Constructor");
}
}
In the example above, it prints Parameterized Vehicle Class Constructor first, and then Car Class Constructor.
NOTE: The call using super must be the first statement in the Constructor for the Child Class. Also, when we explicitly use super in Constructor the compiler doesn't invoke the Parent Constructor automatically.
You should call super() inside the Constructors only and they must be first statement in the Constructors.
Example:
class Vehicle {
Vehicle() {
System.out.println("Vehicle Class Constructor called");
}
}
class Car Extends Vehicle {
Car() {
System.out.println("Car Class Constructor Called");
super(); // compile time error
}
}
In the example above, we notice that the call to Parent Class Constructor, through super(), is not the first statement in the Child Class Constructor. As a result, compilation error occurs.
The Parent Class Constructor needs to be called before the Child Class Constructor. This will ensure that if you call any methods on the Parent Class in your constructor, the Parent Class has already been set up correctly.
How to access methods of Grand Parent Class?
We cannot directly access the methods of the Grand Parent Class using
super.super.methodName().
Example:
class Vehicle { // Grand Parent Class
void display() {
System.out.println("Vehicle Class Method");
}
}
class Car extends Vehicle { // Parent Class
void display() {
System.out.println("Car Class Method");
}
}
class Bike extends Car { // Base(Child) Class
void display() {
super.super.display(); // compile time error
System.out.println("Bike Class Method");
}
}
In the example above, we tried accessing the method display() of the Grand Parent Class, but compilation error occurred.
NOTE: In Java, we can only access methods of Parent Class. So, to access methods of Grand Parent Class, we need to access them through the Parent of the Base Class.
Example:
class Vehicle { // Grand Parent Class
void display() {
System.out.println("Vehicle Class Method");
}
}
class Car extends Vehicle { // Parent Class
void display() {
super.display(); // calls display method of Vehicle Class
System.out.println("Car Class Method");
}
}
class Bike extends Car { // Base(Child) Class
void display() {
super.display(); // calls display method of Car Class
System.out.println("Bike Class Method");
}
}
If we call the display() method of Bike Class now, the output of the above example will be:
Vehicle Class Method
Car Class Method
Bike Class Method
Can super keyword can not be used in a static method.
With this article at OpenGenus, you must have the complete idea of super reference in Java. Enjoy.